现有好评和差评两个文本文件,我需要对这两个中文文本文件作为训练集,使用sklearn实现贝叶斯分类,区分好评和差评,并给出准确率,给出示例代码
时间: 2023-10-06 14:08:02 浏览: 79
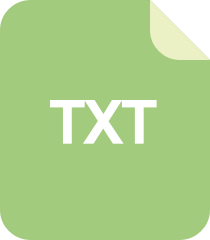
使用sklearn进行中文文本分类-附件资源
以下是示例代码:
```python
import jieba
import os
from sklearn.naive_bayes import MultinomialNB
from sklearn.feature_extraction.text import TfidfVectorizer
# 读取文件
def read_file(file_path):
with open(file_path, 'r', encoding='utf-8') as f:
content = f.read()
return content
# 中文分词
def cut_words(content):
words = list(jieba.cut(content))
return ' '.join(words)
# 加载数据
def load_data():
data = []
labels = []
for label in ['pos', 'neg']:
folder_path = './data/' + label
for file_name in os.listdir(folder_path):
file_path = folder_path + '/' + file_name
content = read_file(file_path)
words = cut_words(content)
data.append(words)
labels.append(label)
return data, labels
# 训练模型
def train():
data, labels = load_data()
# 生成tf-idf矩阵
tfidf_vectorizer = TfidfVectorizer()
x_train = tfidf_vectorizer.fit_transform(data)
# 训练模型
clf = MultinomialNB()
clf.fit(x_train, labels)
return tfidf_vectorizer, clf
# 测试模型
def test(tfidf_vectorizer, clf, text):
x_test = tfidf_vectorizer.transform([cut_words(text)])
y_predict = clf.predict(x_test)
return y_predict[0]
# 计算准确率
def accuracy(tfidf_vectorizer, clf):
data, labels = load_data()
x_test = tfidf_vectorizer.transform(data)
y_predict = clf.predict(x_test)
correct = 0
total = len(labels)
for i in range(total):
if y_predict[i] == labels[i]:
correct += 1
return correct / total
if __name__ == '__main__':
tfidf_vectorizer, clf = train()
acc = accuracy(tfidf_vectorizer, clf)
print('Accuracy: %.2f%%' % (acc * 100))
text = '这家餐厅真心不错'
result = test(tfidf_vectorizer, clf, text)
print(text, '->', result)
text = '这部电影太烂了'
result = test(tfidf_vectorizer, clf, text)
print(text, '->', result)
```
在`load_data()`函数中,我们遍历好评和差评文件夹,读取文件内容并进行中文分词,将处理后的文本和标签存储在`data`和`labels`列表中。
在`train()`函数中,我们使用`TfidfVectorizer`将文本转换为tf-idf矩阵,并使用`MultinomialNB`训练分类器。
在`test()`函数中,我们将待测试的文本转换为tf-idf向量,并使用训练好的分类器进行预测。
在`accuracy()`函数中,我们计算测试集上的准确率。
最后,我们在主函数中训练模型并输出准确率,然后测试两个文本的分类结果。
阅读全文
相关推荐





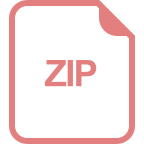
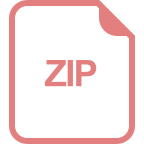
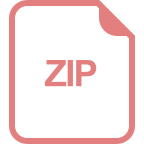
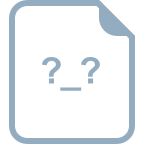
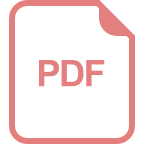
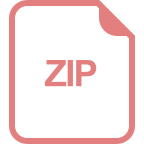
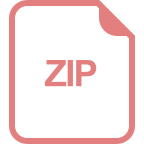
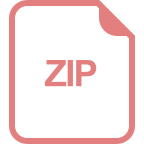
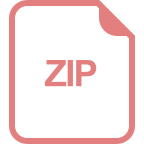
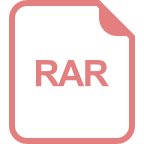