django models.AutoField
时间: 2023-11-27 12:03:10 浏览: 183
Django 的 models.AutoField 是一个自动增长的整数字段类型。它通常用作模型的主键,用于唯一标识每个记录。AutoField 字段在数据库中自动生成一个唯一的整数值,每次插入新记录时都会递增。
使用 AutoField 字段时,Django 将自动为每个模型添加一个名为 "id" 的字段作为主键,并将其类型设置为 AutoField。你不需要手动指定主键字段,Django 会为你处理。
下面是一个使用 AutoField 的示例:
```python
from django.db import models
class MyModel(models.Model):
# 默认情况下,Django 会自动为每个模型添加一个名为 "id" 的 AutoField 字段作为主键
# 这里只需要定义其他的字段
my_field = models.CharField(max_length=100)
```
在这个示例中,Django 会自动为 MyModel 模型创建一个名为 "id" 的自动增长整数字段作为主键。你可以根据需要在模型中定义其他字段,如上面的 "my_field" 字段。
希望这能回答你关于 Django models.AutoField 的问题!如果你还有其他问题,请随时提问。
相关问题
WARNINGS: ?: (staticfiles.W004) The directory 'static' in the STATICFILES_DIRS setting does not exist. buy.Cart: (models.W042) Auto-created primary key used when not defining a primary key type, by default 'django.db.models.AutoField'. HINT: Configure the DEFAULT_AUTO_FIELD setting or the BuyConfig.default_auto_field attribute to point to a subclass of AutoField, e.g. 'django.d b.models.BigAutoField'. buy.Orders: (models.W042) Auto-created primary key used when not defining a primary key type, by default 'django.db.models.AutoField'. HINT: Configure the DEFAULT_AUTO_FIELD setting or the BuyConfig.default_auto_field attribute to point to a subclass of AutoField, e.g. 'django.d b.models.BigAutoField'. sale.Brand: (models.W042) Auto-created primary key used when not defining a primary key type, by default 'django.db.models.AutoField'. HINT: Configure the DEFAULT_AUTO_FIELD setting or the SaleConfig.default_auto_field attribute to point to a subclass of AutoField, e.g. 'django. db.models.BigAutoField'. sale.Carinfo: (models.W042) Auto-created primary key used when not defining a primary key type, by default 'django.db.models.AutoField'. HINT: Configure the DEFAULT_AUTO_FIELD setting or the SaleConfig.default_auto_field attribute to point to a subclass of AutoField, e.g. 'django. db.models.BigAutoField'. userinfo.UserInfo: (models.W042) Auto-created primary key used when not defining a primary key type, by default 'django.db.models.AutoField'. HINT: Configure the DEFAULT_AUTO_FIELD setting or the UserinfoConfig.default_auto_field attribute to point to a subclass of AutoField, e.g. 'dja ngo.db.models.BigAutoField'. No changes detected
The warnings indicate the following issues:
- `staticfiles.W004`: The `STATICFILES_DIRS` setting is configured to use a directory named `static`, but it does not exist in your project. You should either create the directory or modify the setting to use an existing directory.
- `models.W042`: Auto-created primary keys are being used for several of your models (`buy.Cart`, `buy.Orders`, `sale.Brand`, `sale.Carinfo`, `userinfo.UserInfo`). You should explicitly define a primary key field for each model instead of relying on the default auto-generated primary key. To do this, add a `primary_key=True` argument to a field in the model definition, or specify a custom primary key field using the `primary_key` attribute.
- `No changes detected`: This is not a warning, but rather an informational message indicating that no changes were detected in your models that require migration.
To address the `models.W042` warnings, you can either add a `primary_key=True` argument to an existing field or create a new field with the `primary_key=True` argument. For example:
```python
class Cart(models.Model):
cart_id = models.AutoField(primary_key=True)
# ...
```
Alternatively, you can configure the `DEFAULT_AUTO_FIELD` setting in your project settings to specify a custom primary key field for all models in your project. For example:
```python
# settings.py
from django.conf import settings
class BuyConfig(AppConfig):
default_auto_field = 'django.db.models.BigAutoField'
settings.configure(BUY_CONFIG=BuyConfig)
```
This will set the default auto-generated primary key field for all models in the `buy` app to `BigAutoField`. You can repeat this pattern for other apps in your project as well.
WARNINGS: ?: (staticfiles.W004) The directory 'F:\PyCharmProjects\py-goods\static/' in the STATICFILES_DIRS setting does not exist. app01.List: (models.W042) Auto-created primary key used when not defining a primary key type, by default 'django.db.models.AutoField'. HINT: Configure the DEFAULT_AUTO_FIELD setting or the App01Config.default_auto_field attribute to point to a subclass of AutoField, e.g. 'django.db.models.BigAutoField'. app01.message: (models.W042) Auto-created primary key used when not defining a primary key type, by default 'django.db.models.AutoField'. HINT: Configure the DEFAULT_AUTO_FIELD setting or the App01Config.default_auto_field attribute to point to a subclass of AutoField, e.g. 'django.db.models.BigAutoField'. app01.product: (models.W042) Auto-created primary key used when not defining a primary key type, by default 'django.db.models.AutoField'. HINT: Configure the DEFAULT_AUTO_FIELD setting or the App01Config.default_auto_field attribute to point to a subclass of AutoField, e.g. 'django.db.models.BigAutoField'. Operations to perform: Apply all migrations: admin, app01, auth, contenttypes, sessions Running migrations: No migrations to apply.‘’
这是 Django 在运行时输出的一些警告信息。其中:
- 第一个警告是因为你在 `STATICFILES_DIRS` 中设置了一个不存在的目录,需要确认是否设置正确或者创建该目录。
- 后面三个警告是因为你在定义模型时没有显式地指定主键类型,Django 会自动使用 `AutoField`,但是该字段在 MySQL 中会默认为 `int(11)`,可能会导致一些问题。建议在模型定义中显式指定主键类型,或者为应用程序配置 `DEFAULT_AUTO_FIELD` 或 `App01Config.default_auto_field` 属性来指定自动主键类型。
- 最后的信息表示当前没有需要执行的数据库迁移操作。
阅读全文
相关推荐
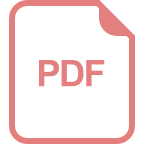
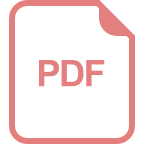






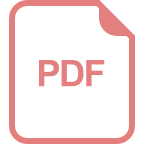
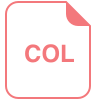
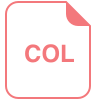





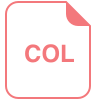