mgento2 自定义发送多个pdf附件的邮件
时间: 2023-06-30 09:16:54 浏览: 105
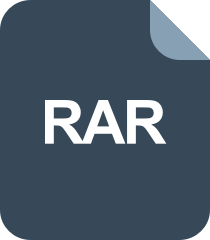
邮件多文件发送功能
要在Magento2中发送多个PDF附件的邮件,您可以使用以下步骤:
1. 创建一个新的Magento模块(如果您尚未创建)。
2. 在您的模块中创建一个新的PHP文件,例如“EmailSender.php”,并编写以下代码:
```php
<?php
namespace [YourNamespace]\[YourModule]\Helper;
use Magento\Framework\App\Filesystem\DirectoryList;
use Magento\Framework\Exception\MailException;
use Magento\Framework\Mail\Template\TransportBuilder;
use Magento\Framework\App\Helper\Context;
use Magento\Framework\App\Helper\AbstractHelper;
use Magento\Framework\Filesystem;
class EmailSender extends AbstractHelper
{
protected $transportBuilder;
protected $filesystem;
protected $directoryList;
public function __construct(
Context $context,
TransportBuilder $transportBuilder,
Filesystem $filesystem,
DirectoryList $directoryList
) {
parent::__construct($context);
$this->transportBuilder = $transportBuilder;
$this->filesystem = $filesystem;
$this->directoryList = $directoryList;
}
public function sendEmailWithAttachments($toEmail, $toName, $subject, $body, $attachments) {
try {
$transport = $this->transportBuilder
->setTemplateIdentifier('your_email_template_identifier')
->setTemplateOptions([
'area' => 'frontend',
'store' => \Magento\Store\Model\Store::DEFAULT_STORE_ID,
])
->setTemplateVars([
'subject' => $subject,
'body' => $body,
])
->setFrom([
'email' => 'your_email@example.com',
'name' => 'Your Name',
])
->addTo([
'email' => $toEmail,
'name' => $toName,
]);
foreach ($attachments as $attachment) {
$fileFullPath = $this->directoryList->getPath('var') . '/attachments/' . $attachment['file_name'];
file_put_contents($fileFullPath, $attachment['file_content']);
$transport->addAttachment(
file_get_contents($fileFullPath),
$attachment['file_name']
);
}
$transport->getTransport()->sendMessage();
} catch (MailException $e) {
// Handle mail sending errors here
}
}
}
```
3. 在您的模块的“etc”文件夹中创建一个新的XML文件,例如“email_templates.xml”,并编写以下代码:
```xml
<config xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:noNamespaceSchemaLocation="urn:magento:module:Magento_Email:etc/email_templates.xsd">
<template id="your_email_template_identifier" label="Your Email Template" file="your_email_template.html" type="html" module="[YourNamespace]_[YourModule]" area="frontend"/>
</config>
```
4. 在您的模块中创建一个新的HTML文件,例如“your_email_template.html”,并编写以下代码:
```html
<!-- Your email template HTML code here -->
<h1>{{var subject}}</h1>
<p>{{var body}}</p>
```
5. 在您的代码中调用“EmailSender”帮助程序中的“sendEmailWithAttachments”方法,并传递电子邮件地址,主题,正文和附件数组作为参数。 例如:
```php
$attachments = [
[
'file_name' => 'attachment1.pdf',
'file_content' => file_get_contents('/path/to/attachment1.pdf'),
],
[
'file_name' => 'attachment2.pdf',
'file_content' => file_get_contents('/path/to/attachment2.pdf'),
],
];
$this->emailSender->sendEmailWithAttachments(
'recipient@example.com',
'Recipient Name',
'Email Subject',
'Email Body',
$attachments
);
```
请注意,此代码示例中的附件数组包含每个附件的文件名和内容。您可以根据需要更改此代码以满足您的需求。
阅读全文
相关推荐
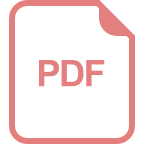
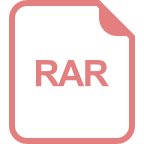
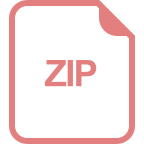
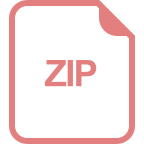
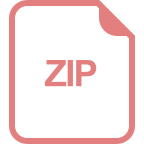
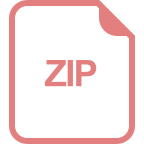
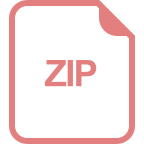
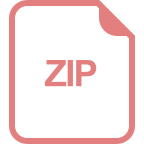
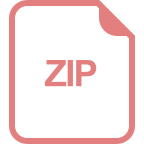
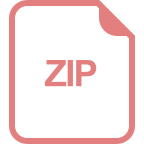
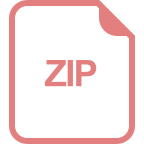