public static void show() {//系统选择交互界面方法 while (true) { System.out.println("--------欢迎进入汽车之家--------"); System.out.println("1-进入管理员系统"); System.out.println("2-进入普通租赁用户系统"); System.out.println("3-退出"); System.out.println("请输入您想要进行的操作:"); Scanner user = new Scanner(System.in); int temp = user.nextInt(); //输入命令判断 switch (temp) { case 1: CheckPwd(user);//管理员登录验证 break; case 2: UserSys.User(listCar,bus,GoodsCar, user);//用户系统 break; case 3: System.out.println("感谢您的信任与支持,再见!"); System.exit(0); break; default: System.out.println("请做个遵守规则的用户哦\n"); } } }
时间: 2023-12-29 09:06:45 浏览: 74
这段代码是一个 Java 程序的一部分,通过控制台实现交互式选择汽车租赁系统的功能。当程序运行时,会显示欢迎信息和操作选项,用户可以输入数字选择进入管理员系统、普通租赁用户系统或退出程序。根据用户的选择,程序会调用不同的方法进行处理。其中,管理员系统需要进行登录验证,而用户系统则需要传入车辆列表等参数来进行操作。如果用户输入的数字不在可选范围内,则会提示重新输入。程序会一直循环,直到用户选择退出。
相关问题
a) 定义一个名为Question的类,用于存储单个问题的文本、可选答案和正确答案索引; b) 编写一个方法,使用JFileChooser选择文件并将其中所有问题读入Question对象的数组/列表中(假设文件的结构如上所述); c) 设计并实现一个GUI,包含菜单、显示问题和答案的能力、选择答案的能力、显示结果和得分的能力,并且能够进入下一个问题。合适的布局(1P)、类扩展JFrame(0.25P)、类符合OOP原则(0.25P)和在main方法中进行全局设置(0.25P); d) 编写一个方法,在GUI上显示一个问题; e) 实现一个actionPerformed方法,以响应用户与GUI的交互。请确保根据需要启用和禁用交互组件,例如,用户在未选择答案的情况下不应该能够跳过下一个问题,也不应该在完成当前测验之前能够加载新的测验。 以上是整个程序的要求,需要按照要求实现一个包含多个问题的测验程序,并且具有良好的GUI界面。怎么写?
这是一个相对复杂的程序,需要多个类和方法来实现。以下是一个大致的实现思路:
1. 定义Question类,包含问题文本、可选答案和正确答案索引属性,并且编写相应的getters和setters方法。
2. 编写一个方法,使用JFileChooser选择文件并将其中所有问题读入Question对象的数组/列表中。可以使用Scanner类来读取文件,将每个问题的文本和答案读入Question对象中,并加入到列表中。
3. 设计并实现一个GUI,包含菜单、问题和答案的展示区、选项按钮、结果和得分的展示区,以及“下一题”和“提交”按钮。可以使用JFrame和JPanel等组件来实现,需要合适的布局方式。
4. 编写一个方法,用于在GUI上显示一个问题。该方法可以在“下一题”按钮的actionPerformed方法中调用,以显示下一个问题。
5. 实现一个actionPerformed方法,以响应用户与GUI的交互。在该方法中,需要判断用户是否选择了正确的答案,更新得分和结果展示区的内容,并且根据需要启用和禁用交互组件。例如,当用户未选择答案时,“下一题”按钮应该被禁用,直到选择了答案才能启用。
以下是一个简化的示例代码,用于说明实现思路:
```
import java.awt.BorderLayout;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import java.io.File;
import java.io.FileNotFoundException;
import java.util.ArrayList;
import java.util.List;
import java.util.Scanner;
import javax.swing.JButton;
import javax.swing.JFileChooser;
import javax.swing.JFrame;
import javax.swing.JLabel;
import javax.swing.JPanel;
import javax.swing.JRadioButton;
import javax.swing.ButtonGroup;
public class Quiz extends JFrame implements ActionListener {
private JLabel questionLabel;
private JRadioButton[] answerButtons;
private JButton nextButton, submitButton;
private JPanel questionPanel, answerPanel, buttonPanel, resultPanel;
private ButtonGroup answerGroup;
private List<Question> questions;
private int currentQuestion;
private int score;
private JLabel resultLabel;
public Quiz() {
// 设置窗口属性
setTitle("Quiz");
setSize(400, 300);
setDefaultCloseOperation(EXIT_ON_CLOSE);
// 初始化组件
questionLabel = new JLabel();
answerButtons = new JRadioButton[4];
for (int i = 0; i < 4; i++) {
answerButtons[i] = new JRadioButton();
}
nextButton = new JButton("Next");
nextButton.addActionListener(this);
submitButton = new JButton("Submit");
submitButton.addActionListener(this);
answerGroup = new ButtonGroup();
questionPanel = new JPanel();
questionPanel.add(questionLabel);
answerPanel = new JPanel();
for (int i = 0; i < 4; i++) {
answerPanel.add(answerButtons[i]);
answerGroup.add(answerButtons[i]);
}
buttonPanel = new JPanel();
buttonPanel.add(nextButton);
buttonPanel.add(submitButton);
resultPanel = new JPanel();
resultLabel = new JLabel();
resultPanel.add(resultLabel);
// 添加组件到窗口
add(questionPanel, BorderLayout.NORTH);
add(answerPanel, BorderLayout.CENTER);
add(buttonPanel, BorderLayout.SOUTH);
add(resultPanel, BorderLayout.EAST);
// 初始化题目列表和当前题目编号
questions = new ArrayList<Question>();
currentQuestion = 0;
score = 0;
}
// 从文件中读取题目
public void readQuestionsFromFile(File file) throws FileNotFoundException {
Scanner scanner = new Scanner(file);
while (scanner.hasNextLine()) {
String questionText = scanner.nextLine();
String[] answers = new String[4];
for (int i = 0; i < 4; i++) {
answers[i] = scanner.nextLine();
}
int correctAnswerIndex = scanner.nextInt();
scanner.nextLine(); // consume newline after correct answer index
Question question = new Question(questionText, answers, correctAnswerIndex);
questions.add(question);
}
scanner.close();
}
// 在GUI上显示一个问题
public void showQuestion(Question question) {
questionLabel.setText(question.getText());
String[] answers = question.getAnswers();
for (int i = 0; i < 4; i++) {
answerButtons[i].setText(answers[i]);
answerButtons[i].setSelected(false);
}
}
// 处理用户交互事件
public void actionPerformed(ActionEvent e) {
if (e.getSource() == nextButton) {
// 显示下一题
currentQuestion++;
if (currentQuestion < questions.size()) {
showQuestion(questions.get(currentQuestion));
nextButton.setEnabled(false);
} else {
// 所有题目已经回答完毕,显示得分和结果
questionPanel.setVisible(false);
answerPanel.setVisible(false);
buttonPanel.setVisible(false);
resultLabel.setText("Score: " + score + "/" + questions.size());
resultPanel.setVisible(true);
}
} else if (e.getSource() == submitButton) {
// 检查答案是否正确,并更新得分和结果
Question question = questions.get(currentQuestion);
int selectedAnswerIndex = -1;
for (int i = 0; i < 4; i++) {
if (answerButtons[i].isSelected()) {
selectedAnswerIndex = i;
}
}
if (selectedAnswerIndex == question.getCorrectAnswerIndex()) {
score++;
resultLabel.setText("Correct!");
} else {
resultLabel.setText("Incorrect. The correct answer is: " + question.getCorrectAnswer());
}
nextButton.setEnabled(true);
submitButton.setEnabled(false);
answerGroup.clearSelection();
}
}
public static void main(String[] args) {
Quiz quiz = new Quiz();
try {
JFileChooser chooser = new JFileChooser();
int result = chooser.showOpenDialog(quiz);
if (result == JFileChooser.APPROVE_OPTION) {
File file = chooser.getSelectedFile();
quiz.readQuestionsFromFile(file);
quiz.showQuestion(quiz.questions.get(0));
quiz.nextButton.setEnabled(false);
}
} catch (FileNotFoundException e) {
System.out.println("File not found.");
}
quiz.setVisible(true);
}
}
class Question {
private String text;
private String[] answers;
private int correctAnswerIndex;
public Question(String text, String[] answers, int correctAnswerIndex) {
this.text = text;
this.answers = answers;
this.correctAnswerIndex = correctAnswerIndex;
}
public String getText() {
return text;
}
public String[] getAnswers() {
return answers;
}
public int getCorrectAnswerIndex() {
return correctAnswerIndex;
}
public String getCorrectAnswer() {
return answers[correctAnswerIndex];
}
}
```
以上是一个简单的实现示例,可以根据实际需要进行修改和扩展。
用java编写1.编写一个程序,程序提供记事本功能: 1) 构建记事本类,该类能存储不定数量的记录;能获得已经存储的记录数 量;能追加记录;能展示已经存储的全部记录或其中任何一条记录;能 删除已经存储的全部记录或其中任何一条记录。 2) 构建测试类,该类实现与用户的交互,向用户提示操作信息,并接收用 户的操作请求。 程序应具有良好的人机交互性能,即:程序应向用户提示功能说明,并可根 据用户的功能选择,执行对应的功能,并给出带详细描述信息的最终执行结果
以下是一个简单的Java代码实现以上要求:
```
import java.util.ArrayList;
import java.util.Scanner;
public class Notepad {
private ArrayList<String> records;
public Notepad() {
records = new ArrayList<String>();
}
public int getRecordCount() {
return records.size();
}
public void addRecord(String record) {
records.add(record);
}
public void showRecords() {
if (records.size() == 0) {
System.out.println("No records found.");
} else {
for (int i = 0; i < records.size(); i++) {
System.out.println((i+1) + ". " + records.get(i));
}
}
}
public void deleteRecord(int index) {
if (index < 0 || index >= records.size()) {
System.out.println("Invalid index.");
} else {
records.remove(index);
System.out.println("Record deleted.");
}
}
public void deleteAllRecords() {
records.clear();
System.out.println("All records deleted.");
}
}
public class NotepadTest {
public static void main(String[] args) {
Notepad notepad = new Notepad();
Scanner scanner = new Scanner(System.in);
while (true) {
System.out.println("What would you like to do?");
System.out.println("1. Add record");
System.out.println("2. Show records");
System.out.println("3. Delete record");
System.out.println("4. Delete all records");
System.out.println("5. Exit");
int choice = scanner.nextInt();
switch (choice) {
case 1:
scanner.nextLine(); // clear the carriage return
System.out.println("Enter record:");
String record = scanner.nextLine();
notepad.addRecord(record);
System.out.println("Record added.");
break;
case 2:
notepad.showRecords();
break;
case 3:
System.out.println("Enter index of record to delete:");
int index = scanner.nextInt();
notepad.deleteRecord(index-1);
break;
case 4:
notepad.deleteAllRecords();
break;
case 5:
System.exit(0);
default:
System.out.println("Invalid choice.");
}
}
}
}
```
在这个程序中,我们首先定义了一个Notepad类来实现记事本的功能,包括添加记录、获取记录数量、展示记录、删除记录等方法。然后我们在NotepadTest类中实现与用户的交互,根据用户的选择调用Notepad类的相应方法来完成记事本的操作。
注意,在删除记录时,我们要求用户输入记录的索引,但是由于ArrayList的索引是从0开始的,而用户往往习惯从1开始计数,因此我们在删除记录时要将用户输入的索引减1。
阅读全文
相关推荐
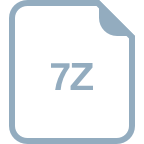
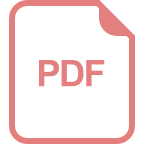
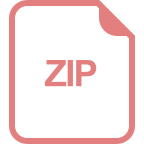
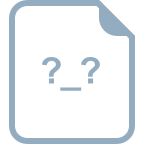
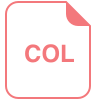
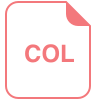
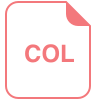
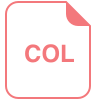
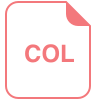
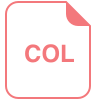
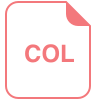
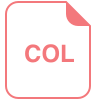
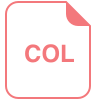
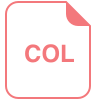
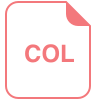
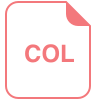
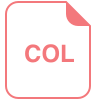
