C++对对象数组进行排序
时间: 2023-08-12 13:26:46 浏览: 181
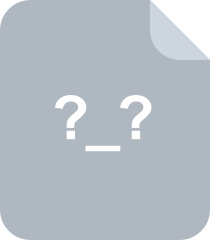
c++对数组的排序
可以使用STL库中的sort函数对对象数组进行排序。以学生类为例,示例代码如下:
```cpp
#include <iostream>
#include <vector>
#include <algorithm>
using namespace std;
class Student {
public:
string name;
int score;
Student(string _name, int _score)
: name(_name), score(_score) {}
bool operator < (const Student& other) const {
return this->score < other.score;
}
};
int main()
{
vector<Student> vec {{"Alice", 85}, {"Bob", 90}, {"Cindy", 80}, {"David", 95}};
// 使用sort函数进行排序
sort(vec.begin(), vec.end());
// 输出排序后的结果
for (auto stu : vec) {
cout << stu.name << " " << stu.score << " ";
}
return 0;
}
```
输出结果为:Cindy 80 Alice 85 Bob 90 David 95。
在代码中,我们定义了一个学生类Student,包含了学生姓名和分数两个成员变量,并定义了小于运算符重载函数,用于自定义排序规则。在主函数中,我们首先定义了一个学生类对象数组vec,其中包含了4个学生对象。然后我们使用sort函数对vec进行排序,sort函数会自动调用类的小于运算符重载函数进行排序。最后使用for循环输出排序后的结果。
本例中自定义排序规则为按照学生成绩从小到大排序,如果需要按照其他规则进行排序,只需要修改类中的小于运算符重载函数即可。
阅读全文
相关推荐
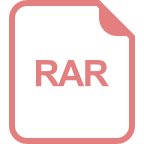
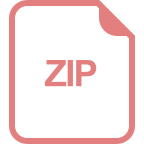
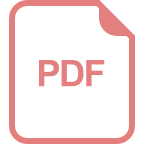
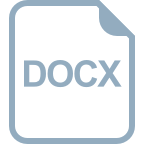
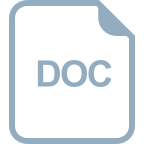
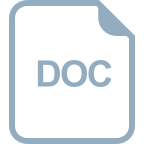
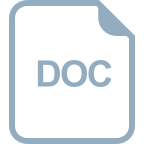
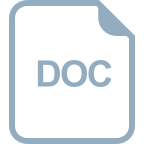


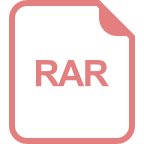
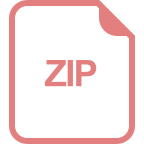
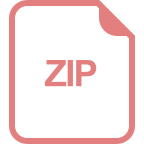
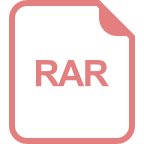
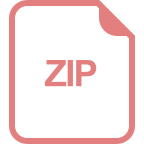
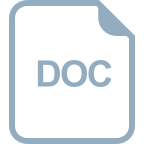