public void addMusicOff() { JButton button = new JButton("OFF"); button.setLocation(HEIGTH - 110, HEIGTH / 10 + 420); button.setSize(80, 60); button.setFont(new Font("Rockwell", Font.BOLD, 20)); add(button); button.addActionListener(new ActionListener() { @Override public void actionPerformed(ActionEvent e) { if (clip != null) { clip.stop(); } } }); } private Clip clip;怎么让这里的clip不为null
时间: 2024-01-15 08:03:02 浏览: 21
要让 `clip` 不为 `null`,你需要先实例化一个 `Clip` 对象并将其赋值给 `clip` 变量。你可以使用 `AudioSystem` 类的 `getClip()` 方法来获取 `Clip` 对象。例如:
```
AudioInputStream ais = AudioSystem.getAudioInputStream(new File("music.wav"));
clip = AudioSystem.getClip();
clip.open(ais);
```
这将从名为 "music.wav" 的音频文件中创建一个 `AudioInputStream` 对象,然后将其传递给 `getClip()` 方法来获取 `Clip` 对象。最后,调用 `open()` 方法将音频数据加载到 `Clip` 对象中,使其准备好播放。这样,你就可以在 `addMusicOff()` 方法中安全地访问 `clip` 对象了。
相关问题
private void addRules() { JButton button = new JButton("Rules"); button.setLocation(HEIGTH, HEIGTH / 10 + 540); button.setSize(200, 60); button.setFont(new Font("Rockwell", Font.BOLD, 20)); add(button); button.addActionListener(e -> { }); }完善这个方法,使其被点击时,能弹出一个可关闭的窗口,窗口中显示rules,并且可以通过调整参数改变窗口大小
可以使用 JDialog 类来实现这个功能,以下是完善后的代码:
```
private void addRules() {
JButton button = new JButton("Rules");
button.setLocation(HEIGTH, HEIGTH / 10 + 540);
button.setSize(200, 60);
button.setFont(new Font("Rockwell", Font.BOLD, 20));
add(button);
button.addActionListener(e -> {
// 创建一个 JDialog 对象
JDialog dialog = new JDialog();
dialog.setTitle("Rules");
dialog.setModal(true); // 设置为模态对话框
dialog.setSize(400, 300); // 设置对话框大小
dialog.setLocationRelativeTo(null); // 设置对话框居中显示
// 创建一个 JTextArea 对象,用于显示规则内容
JTextArea textArea = new JTextArea("这里是规则内容");
textArea.setEditable(false); // 设置为只读模式
textArea.setLineWrap(true); // 设置自动换行
textArea.setWrapStyleWord(true); // 设置换行时以单词为单位
JScrollPane scrollPane = new JScrollPane(textArea); // 创建一个滚动窗格,用于显示 JTextArea
dialog.add(scrollPane); // 将滚动窗格添加到对话框中
dialog.setVisible(true); // 显示对话框
});
}
```
这样,在用户点击 "Rules" 按钮时,就会弹出一个可关闭的对话框,其中显示着规则内容,并且可以通过调整参数改变对话框的大小。
public Calculator() { super("Calculator"); this.setLocationRelativeTo(null);// set up window this.setResizable(false); this.setDefaultCloseOperation(EXIT_ON_CLOSE); this.setSize(600, 200); result = new JPanel(); answer = 0.0 ; show = new JLabel(Double.toString(answer), SwingConstants.RIGHT);// let the result at the right of the field result.add(show); JTextField input = new JTextField(Double.toString(answer));// setup the size of the text field input.setColumns(6); input.setHorizontalAlignment(JTextField.RIGHT); button = new JPanel(); button.setLayout(new GridLayout(1, 5)); CLR = new JButton("CLR");// add the buttons of calculation. add = new JButton("+"); minus = new JButton("-"); Multiply = new JButton("*"); divide = new JButton("/"); button.add(CLR); button.add(add); button.add(minus); button.add(Multiply); button.add(divide); CLR.addActionListener(e -> {// clear answer = 0.0; show.setText(Double.toString(answer)); field.setText(" "); }); add.addActionListener(e -> {// add number = field.getText(); if (!number.equals(null)) { double value = Double.parseDouble(number); answer += value; show.setText(Double.toString(answer)); field.setText(""); } }); minus.addActionListener(e -> {// minus number = field.getText(); if (!number.equals("")) { double value = Double.parseDouble(number); answer -= value; show.setText(Double.toString(answer)); field.setText(""); } }); Multiply.addActionListener(e -> {// multiply number = field.getText(); if (!number.equals("")) { double value = Double.parseDouble(number); answer *= value; show.setText(Double.toString(answer)); field.setText(""); } }); divide.addActionListener(e -> {// divide number = field.getText(); if (!number.equals("")) { double value = Double.parseDouble(number); answer /= value; show.setText(Double.toString(answer)); field.setText(""); } }); add(button); add(field); add(result); this.setLayout(new GridLayout(3, 1)); this.setVisible(true); }错哪了
这段代码似乎是一个简单的计算器程序,但是在代码中没有定义 `field` 变量,因此在添加 `field` 变量时,需要先进行定义。此外,在 `Multiply` 和 `divide` 的实现中,没有对除数为0的情况进行处理,这会导致程序抛出异常。因此应该在这两个实现中添加对除数为0的情况进行处理的代码。
相关推荐
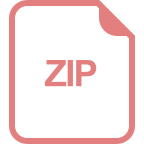
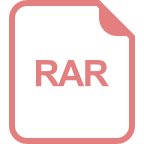











