C语言利用指针计算两个矩阵的和
时间: 2024-05-11 21:19:39 浏览: 12
下面是使用指针计算两个矩阵的和的C语言代码示例:
```c
#include <stdio.h>
#include <stdlib.h>
int main()
{
int rows, cols, i, j;
int *mat1, *mat2, *res;
printf("Enter the number of rows: ");
scanf("%d", &rows);
printf("Enter the number of columns: ");
scanf("%d", &cols);
// allocate memory for matrices
mat1 = (int *)malloc(rows * cols * sizeof(int));
mat2 = (int *)malloc(rows * cols * sizeof(int));
res = (int *)malloc(rows * cols * sizeof(int));
// input elements of matrix 1
printf("Enter elements of matrix 1:\n");
for(i=0; i<rows; i++)
{
for(j=0; j<cols; j++)
{
scanf("%d", mat1+i*cols+j);
}
}
// input elements of matrix 2
printf("Enter elements of matrix 2:\n");
for(i=0; i<rows; i++)
{
for(j=0; j<cols; j++)
{
scanf("%d", mat2+i*cols+j);
}
}
// add two matrices
for(i=0; i<rows; i++)
{
for(j=0; j<cols; j++)
{
*(res+i*cols+j) = *(mat1+i*cols+j) + *(mat2+i*cols+j);
}
}
// print the result
printf("Resultant matrix:\n");
for(i=0; i<rows; i++)
{
for(j=0; j<cols; j++)
{
printf("%d ", *(res+i*cols+j));
}
printf("\n");
}
// deallocate memory
free(mat1);
free(mat2);
free(res);
return 0;
}
```
在上面的代码中,我们首先从用户输入矩阵的行数和列数,然后动态分配了三个指针:mat1,mat2和res,分别用于存储两个输入矩阵和它们的和。在输入矩阵元素后,我们使用两个for循环遍历矩阵并使用指针进行操作。在计算和时,我们使用指针对应的值,并将结果存储在res指针中。最后,我们打印出结果并释放了动态分配的内存。
相关推荐
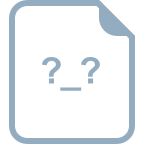
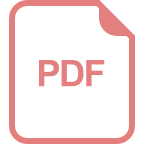
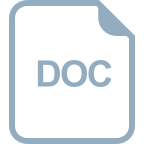
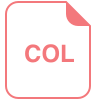
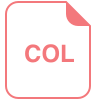
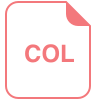
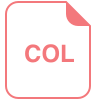
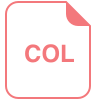









