C++Tom从小就对英文字母非常感兴趣,尤其是元音字母(a,e,i,o,u,A,E,I,O,U),他在写作文的时候都会把元音字母写成大写的,辅音字母则都写成小写, 你试试把一个句子翻译成他写作文的习惯吧。 请使用字符串流解决该问题,需要有main函数,要有注释(最好英文) 输入一个字符串S(长度不超过100,只包含大小写的英文字母和空格)。
时间: 2024-01-31 22:08:19 浏览: 60
Here is the code in C++:
```cpp
#include <iostream>
#include <sstream>
#include <string>
using namespace std;
int main() {
string s; // declare a string variable to store the input
getline(cin, s); // read a line of input from the console
stringstream ss(s); // create a stringstream object with the input
string word; // declare a string variable to store each word in the input
while (ss >> word) { // read each word from the stringstream
for (int i = 0; i < word.length(); i++) { // iterate through each character in the word
if (word[i] == 'a' || word[i] == 'e' || word[i] == 'i' || word[i] == 'o' || word[i] == 'u' ||
word[i] == 'A' || word[i] == 'E' || word[i] == 'I' || word[i] == 'O' || word[i] == 'U') {
// if the character is a vowel, convert it to uppercase
word[i] = toupper(word[i]);
} else {
// otherwise, convert it to lowercase
word[i] = tolower(word[i]);
}
}
cout << word << " "; // output the modified word with a space
}
return 0;
}
```
Explanation:
1. We first declare a string variable `s` to store the input.
2. We read a line of input using `getline(cin, s)`.
3. We create a `stringstream` object `ss` with the input string `s`.
4. We declare a string variable `word` to store each word in the input.
5. We use a `while` loop to read each word from the `stringstream` using `ss >> word`.
6. For each word, we iterate through each character using a `for` loop.
7. If the character is a vowel (either lowercase or uppercase), we convert it to uppercase using `toupper()`.
8. Otherwise, we convert it to lowercase using `tolower()`.
9. We output the modified word with a space using `cout << word << " "`.
10. The program terminates after all the words have been processed.
阅读全文
相关推荐
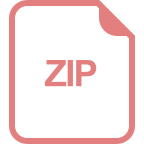
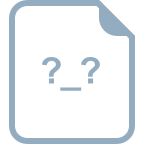
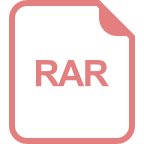
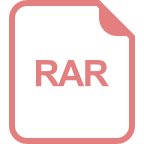
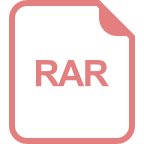
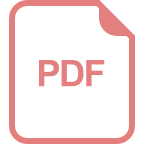
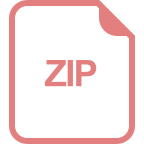
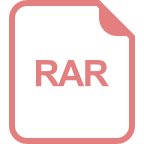
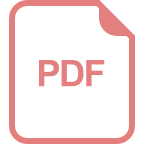
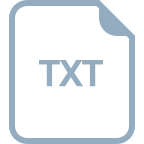
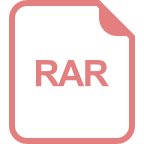