vue 使用js完成绘制导图
时间: 2023-09-17 20:13:37 浏览: 125
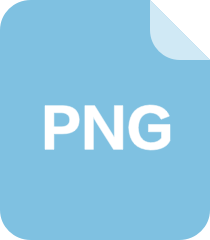
vue.js 体系结构导图

要使用Vue.js完成绘制导图,可以使用JavaScript绘图库,如d3.js、vis.js或mxGraph,结合Vue.js来实现。以下是一个使用d3.js和Vue.js绘制导图的简单示例:
1. 首先,在Vue.js组件中引入d3.js库:
```js
import * as d3 from 'd3';
```
2. 然后,在Vue.js组件的模板中创建一个包含SVG元素的div,用于绘制导图:
```html
<template>
<div ref="graph"></div>
</template>
```
3. 在Vue.js组件的JavaScript代码中,使用d3.js来绘制导图:
```js
<script>
export default {
data() {
return {
nodes: [
{ id: 1, name: 'Node 1' },
{ id: 2, name: 'Node 2' },
{ id: 3, name: 'Node 3' },
{ id: 4, name: 'Node 4' },
{ id: 5, name: 'Node 5' }
],
links: [
{ source: 1, target: 2 },
{ source: 1, target: 3 },
{ source: 2, target: 4 },
{ source: 3, target: 5 }
]
}
},
mounted() {
const svg = d3.select(this.$refs.graph)
.append('svg')
.attr('width', 500)
.attr('height', 500);
const link = svg.selectAll('.link')
.data(this.links)
.enter()
.append('line')
.attr('class', 'link')
.attr('x1', d => d.source.x)
.attr('y1', d => d.source.y)
.attr('x2', d => d.target.x)
.attr('y2', d => d.target.y);
const node = svg.selectAll('.node')
.data(this.nodes)
.enter()
.append('circle')
.attr('class', 'node')
.attr('r', 10)
.attr('cx', d => d.x)
.attr('cy', d => d.y)
.call(d3.drag()
.on('start', dragstarted)
.on('drag', dragged)
.on('end', dragended)
);
function dragstarted(d) {
d3.select(this).raise().classed('active', true);
}
function dragged(d) {
d3.select(this).attr('cx', d.x = d3.event.x).attr('cy', d.y = d3.event.y);
link.filter(l => l.source === d).attr('x1', d.x).attr('y1', d.y);
link.filter(l => l.target === d).attr('x2', d.x).attr('y2', d.y);
}
function dragended(d) {
d3.select(this).classed('active', false);
}
}
}
</script>
```
在这个示例中,我们在组件的data中定义了一些节点和链接,然后在mounted钩子函数中使用d3.js来创建SVG元素,并使用节点和链接数据来绘制导图。我们还使用d3.drag()方法来启用节点拖拽功能。
这只是一个简单的示例,您可以根据需要调整节点和链接的属性,以及添加更多的交互和效果。
阅读全文
相关推荐
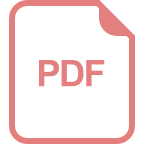
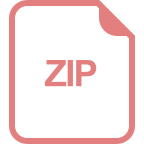
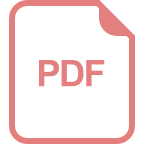
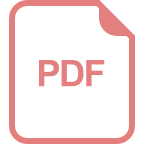
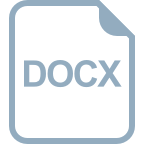
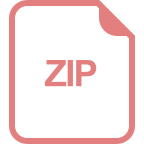
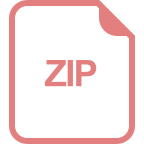
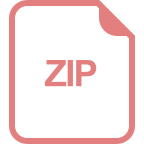
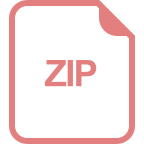
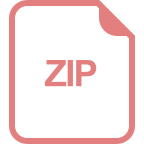
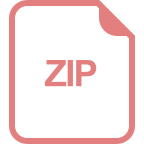
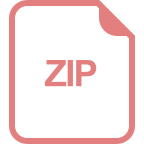
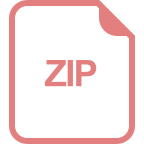
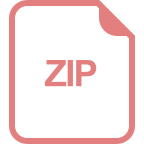
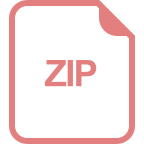
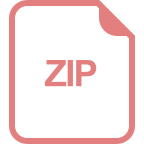
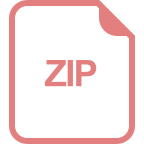