二分查找与左移运算符 来实现一个二分查找 举例c++代码
时间: 2024-08-16 15:08:15 浏览: 50
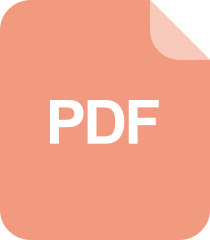
第2章 简单程序设计(C++版)_第二节 运算符和表达式-2021-07-21.pdf
通常,二分查找不会直接使用左移运算符来实现,因为它的核心逻辑是基于比较,而左移则主要用于数据的位操作。然而,在某些特殊场景下,如使用位向量(Bit Vector)来表示状态,可能会涉及两者结合。但是,这里我们还是关注常规的二分查找,它不需要左移操作。
以下是使用C++传统的方式实现二分查找的一个简单示例,假设我们要在一个有序数组中查找某个值:
```cpp
#include <iostream>
#include <vector>
int binarySearch(const std::vector<int>& arr, int target) {
int left = 0;
int right = arr.size() - 1;
while (left <= right) {
int mid = left + (right - left) / 2; // 这里没有使用左移,仅做整数除法
if (arr[mid] == target)
return mid;
else if (arr[mid] < target)
left = mid + 1;
else
right = mid - 1;
}
return -1; // 如果未找到,返回-1作为标识
}
int main() {
std::vector<int> sortedArray = {1, 3, 5, 7, 9};
int target = 5;
int result = binarySearch(sortedArray, target);
if (result != -1)
std::cout << "Element found at index " << result << std::endl;
else
std::cout << "Element not found" << std::endl;
return 0;
}
```
在这个例子中,我们只用到了加法和比较运算,没有使用左移操作。如果要将左移与位向量结合,那将是另一个话题了。
阅读全文
相关推荐
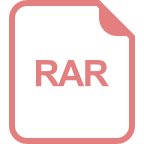
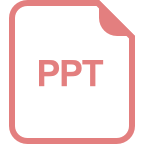
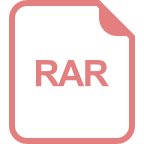
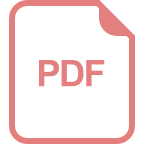
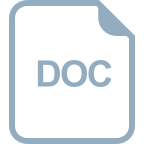
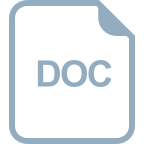
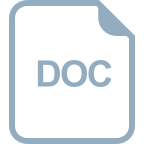
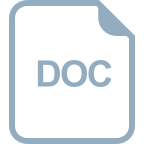
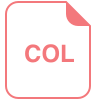
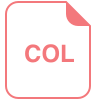
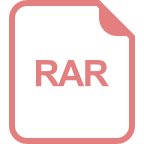
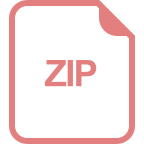