按以下要求定义一个乌龟类和鱼类并尝试编写游戏: 1. 假设游戏场景为范围(x,y)为0<=x<=10,0<=y<=10; 2. 游戏生成1只乌龟和10条鱼; 3. 它们的移动方向均随机; 4. 乌龟的最大移动能力为2(它可以随机选择1还是2移动),鱼儿的最大移动能力是1; 5. 当移动到场景边缘,自动向反方向移动; 6. 乌龟初始化体力为100(上限) 7. 龟每移动一次,体力消耗1; 8. 当乌龟和鱼坐标重叠,乌龟吃掉鱼,乌龟体力增加20; 9. 鱼暂不计算体力; 10. 当乌龟体力值为0(挂掉)或者鱼儿的数量为0游戏结束 提示: import random random.randint(0,10)生成[0,10]之间的随机整数 random.choice([1,2,-1,-2])随机选择列表中一个值 #判断移动后是否超出边界 if new_x<0: self.x=0-new_x elif new_x>10: self.x=10-(new_x-10)
时间: 2024-03-23 21:37:49 浏览: 135
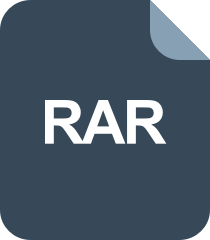
一个人工鱼群的类, 用DOS界面展示的. 类中有觅食,群集,追尾,计算食物密度等方法

好的,我已经了解了你的要求,下面是Python代码实现:
```python
import random
class Turtle:
def __init__(self):
self.power = 100 # 初始化体力为100
# 随机生成乌龟的初始位置
self.x = random.randint(0, 10)
self.y = random.randint(0, 10)
def move(self):
# 随机选择1或2的步长
new_x = self.x + random.choice([1, 2, -1, -2])
new_y = self.y + random.choice([1, 2, -1, -2])
# 判断移动后是否超出边界
if new_x < 0:
self.x = 0 - new_x
elif new_x > 10:
self.x = 10 - (new_x - 10)
else:
self.x = new_x
if new_y < 0:
self.y = 0 - new_y
elif new_y > 10:
self.y = 10 - (new_y - 10)
else:
self.y = new_y
# 每移动一次,体力消耗1
self.power -= 1
return (self.x, self.y)
def eat(self):
self.power += 20 # 乌龟吃鱼,体力增加20
if self.power > 100:
self.power = 100
class Fish:
def __init__(self):
# 随机生成鱼的初始位置
self.x = random.randint(0, 10)
self.y = random.randint(0, 10)
def move(self):
# 随机选择1的步长
new_x = self.x + random.choice([1, -1])
new_y = self.y + random.choice([1, -1])
# 判断移动后是否超出边界
if new_x < 0:
self.x = 0 - new_x
elif new_x > 10:
self.x = 10 - (new_x - 10)
else:
self.x = new_x
if new_y < 0:
self.y = 0 - new_y
elif new_y > 10:
self.y = 10 - (new_y - 10)
else:
self.y = new_y
return (self.x, self.y)
# 生成1只乌龟和10条鱼
turtle = Turtle()
fishes = []
for i in range(10):
fishes.append(Fish())
# 游戏开始
while True:
# 判断乌龟的体力值和鱼的数量
if turtle.power <= 0 or len(fishes) == 0:
break
# 移动乌龟和鱼
turtle_pos = turtle.move()
for fish in fishes[:]:
if fish.move() == turtle_pos:
# 鱼和乌龟的位置重叠,乌龟吃掉鱼,体力增加20
turtle.eat()
fishes.remove(fish)
print("游戏结束!")
```
你可以运行上面的代码进行测试。注意,由于移动步长是随机生成的,所以每次运行结果可能不同。
阅读全文
相关推荐
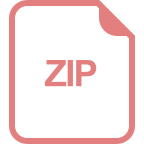
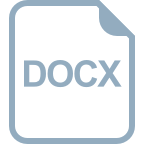
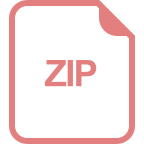
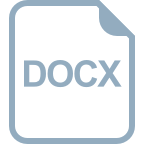
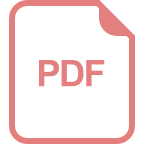
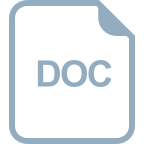
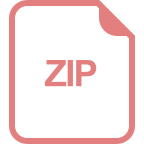
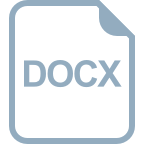
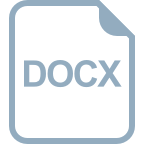
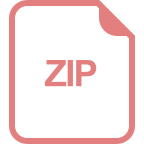
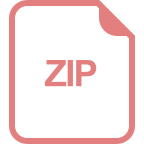
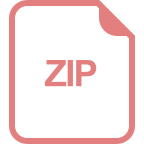
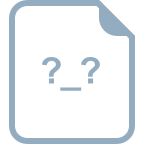
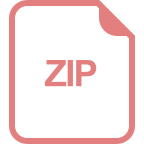
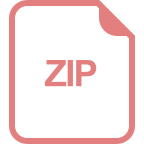
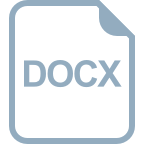
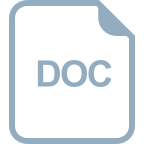