python 判断文件夹是否有文件
时间: 2024-03-12 07:47:22 浏览: 156
你可以使用 Python 的 `os` 模块来判断文件夹是否有文件。具体方法如下:
```python
import os
folder_path = "/path/to/folder"
# 判断文件夹是否存在
if os.path.exists(folder_path) and os.path.isdir(folder_path):
# 判断文件夹是否有文件
if len(os.listdir(folder_path)) > 0:
print("文件夹中有文件")
else:
print("文件夹中没有文件")
else:
print("文件夹不存在")
```
上面的代码首先判断文件夹是否存在,如果存在并且是一个文件夹,就使用 `os.listdir()` 函数获取文件夹中的文件列表,然后判断列表的长度是否大于 0,如果大于 0 就说明文件夹中有文件,否则就说明文件夹中没有文件。
相关问题
python判断文件夹是否存在.rar文件
在Python中,你可以使用`os`模块和`fnmatch`模块来检查文件夹中是否存在特定类型的文件,比如`.rar`文件。下面是一个简单的示例:
```python
import os
import fnmatch
def check_rar_files(folder_path):
rar_pattern = '*.rar'
for root, dirs, files in os.walk(folder_path):
for file in files:
if fnmatch.fnmatch(file, rar_pattern):
return True # 文件存在,返回True
return False # 没有找到.rar文件,返回False
folder_to_check = '/path/to/your/folder'
if check_rar_files(folder_to_check):
print(f"Folder contains at least one .rar file.")
else:
print("Folder does not contain any .rar files.")
python判断文件夹是否空
你可以使用Python的os模块来判断一个文件夹是否为空。具体方法如下:
```python
import os
def is_folder_empty(folder_path):
return len(os.listdir(folder_path)) == 0
```
其中,os.listdir()函数可以列出目录中的所有文件和文件夹,然后通过判断该列表的长度是否为0来判断该文件夹是否为空。如果该函数返回的列表长度为0,则说明文件夹为空,函数返回True;否则说明文件夹不为空,函数返回False。
阅读全文
相关推荐
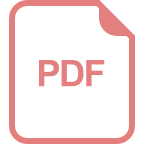




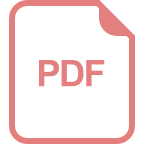
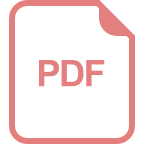







