give an python program to get the numerical solution of Mathieu Equation with example
时间: 2023-05-21 19:00:47 浏览: 122
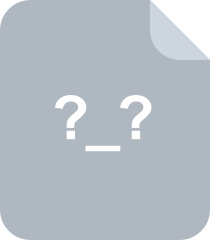
例子 程序求解
Sure, here is an example Python program to get the numerical solution of Mathieu Equation:
```
import numpy as np
from scipy.integrate import odeint
import matplotlib.pyplot as plt
# Define the Mathieu Equation
def mathieu_eq(y, t, q, a):
y1, y2 = y
dydt = [y2, -(a - 2*q*np.cos(2*t))*y1]
return dydt
# Set the initial conditions
y0 = [1, 0]
# Set the parameters
q = 1.5
a = 0.5
# Set the time points
t = np.linspace(0, 10*np.pi, 1000)
# Solve the Mathieu Equation
sol = odeint(mathieu_eq, y0, t, args=(q, a))
# Plot the solution
plt.plot(t, sol[:, 0], 'b', label='y1(t)')
plt.plot(t, sol[:, 1], 'g', label='y2(t)')
plt.legend(loc='best')
plt.xlabel('t')
plt.grid()
plt.show()
```
This program uses the `odeint` function from the `scipy.integrate` module to solve the Mathieu Equation. The equation is defined in the `mathieu_eq` function, and the initial conditions, parameters, and time points are set before calling `odeint`. The solution is then plotted using `matplotlib`.
阅读全文
相关推荐
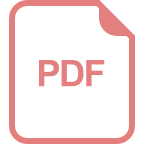
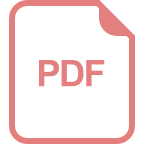
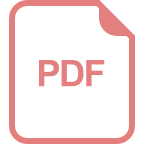
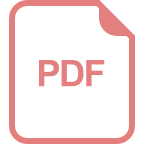
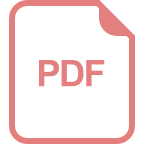
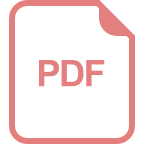
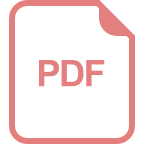
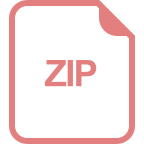
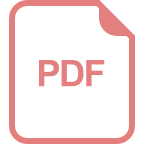
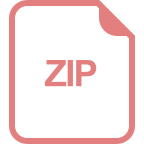
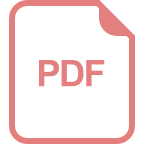
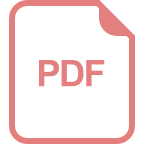
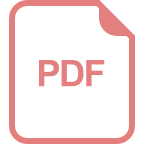
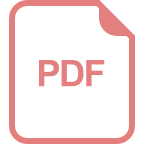