python操作mysql 读取出某一个数据
时间: 2024-05-11 16:16:52 浏览: 4
您好!关于您的问题,您可以使用Python中的MySQLdb模块来连接MySQL数据库,并使用SELECT语句来查询数据库中的数据,例如:
```
import MySQLdb
# 连接数据库
db = MySQLdb.connect(host='localhost', user='username', passwd='password', db='database')
# 获取游标对象
cursor = db.cursor()
# 执行SQL语句
cursor.execute("SELECT * FROM table_name WHERE id=1")
# 获取一条数据
data = cursor.fetchone()
print(data)
# 关闭游标和数据库连接
cursor.close()
db.close()
```
在上面的代码中,我们使用了SELECT语句来查询表中ID为1的数据,并打印出了查询结果。同时,我们还使用了MySQLdb模块来连接数据库和关闭游标和数据库连接。希望能够帮到您,如果您还有其他问题,欢迎继续提问!
相关问题
python mysql 数据类型
Python MySQL 数据类型包括以下几种:
1. 数值类型:包括整型、浮点型、Python MySQL 数据类型包括以下几种:
1. 数值类型:包括整型、浮点型、双精度型等。
2. 字符串类型:包括 char、varchar、text 等。
3. 日期和时间类型:包括 date、time、datetime 等。
4. 布尔类型:包括 bool 类型。
5. 二进制类型:包括 blob、binary 等。
在 Python 中,我们可以使用 pymysql 库来连接 MySQL 数据库,并使用 SQL 语句来操作数据库中的数据。当我们从数据库中取出 varchar 类型的数据时,可以将其转换为 Python 中的字符串类型,然后使用 json.loads() 方法将其转换为 Python 对应的 dict 或 list 类型。
python连接mysql并取数据
连接MySQL需要使用Python中的mysql-connector包,你可以使用以下代码来连接MySQL并取出数据:
```python
import mysql.connector
# 连接MySQL
conn = mysql.connector.connect(host='localhost', user='root', password='password', database='test')
# 创建游标对象
cursor = conn.cursor()
# 执行SQL语句
cursor.execute('SELECT * FROM mytable')
# 获取查询结果
result = cursor.fetchall()
# 打印查询结果
for row in result:
print(row)
# 关闭游标和连接
cursor.close()
conn.close()
```
注意,你需要将上面的 `host`、`user`、`password` 和 `database` 替换成你自己的MySQL服务器信息和数据库名称。另外,需要确保你已经安装了mysql-connector包。
相关推荐
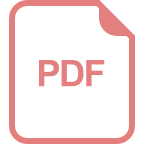
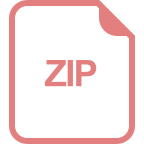












