在数据库中用户名和密码 我该怎么写呢
时间: 2024-03-27 14:37:10 浏览: 8
如果您希望将用户名和密码存储在数据库中,可以使用 Spring Security 提供的 JdbcUserDetailsManager 类来管理用户。以下是一个简单的示例:
1. 首先,在 pom.xml 文件中添加 Spring Security 和 JDBC 相关的依赖项:
```xml
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-security</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-jdbc</artifactId>
</dependency>
<dependency>
<groupId>com.h2database</groupId>
<artifactId>h2</artifactId>
<scope>runtime</scope>
</dependency>
```
2. 然后,您需要在 application.properties 文件中配置数据库连接信息:
```properties
spring.datasource.url=jdbc:h2:mem:testdb
spring.datasource.driverClassName=org.h2.Driver
spring.datasource.username=sa
spring.datasource.password=
```
3. 接下来,创建一个继承自 WebSecurityConfigurerAdapter 的配置类,并注入 DataSource 对象:
```java
@Configuration
@EnableWebSecurity
public class SecurityConfig extends WebSecurityConfigurerAdapter {
@Autowired
private DataSource dataSource;
@Override
protected void configure(AuthenticationManagerBuilder auth) throws Exception {
auth.jdbcAuthentication()
.dataSource(dataSource)
.usersByUsernameQuery("select username, password, enabled from users where username = ?")
.authoritiesByUsernameQuery("select username, authority from authorities where username = ?");
}
@Override
protected void configure(HttpSecurity http) throws Exception {
http.authorizeRequests()
.antMatchers("/api/**").authenticated()
.anyRequest().permitAll()
.and().httpBasic().realmName("MyRealm")
.and().csrf().disable();
}
}
```
4. 在上面的代码中,我们使用了注入的 DataSource 对象来配置 JdbcUserDetailsManager。具体来说,我们使用了 usersByUsernameQuery 和 authoritiesByUsernameQuery 方法来查询用户和权限信息,并使用了基本身份验证。我们还禁用了 CSRF 保护(因为我们的示例比较简单)。
5. 最后,您可以在您的数据库中创建一个 users 表和一个 authorities 表,并在其中存储用户信息和权限信息:
```sql
CREATE TABLE users (
username VARCHAR(50) NOT NULL PRIMARY KEY,
password VARCHAR(100) NOT NULL,
enabled BOOLEAN NOT NULL
);
CREATE TABLE authorities (
username VARCHAR(50) NOT NULL,
authority VARCHAR(50) NOT NULL,
CONSTRAINT fk_authorities_users FOREIGN KEY (username) REFERENCES users(username)
);
```
在上面的代码中,我们创建了一个名为 users 的表,其中包含用户名、密码和启用状态。我们还创建了一个名为 authorities 的表,其中包含用户名和权限信息。
希望这些信息能够帮助您!
相关推荐
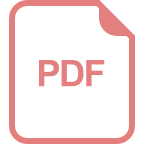
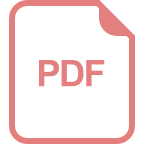















