src/template_match.cpp:101:1: warning: no return statement in function returning non-void [-Wreturn-type] }
时间: 2024-03-09 13:51:16 浏览: 41
这个警告提示表明,在一个非`void`函数中,存在没有返回值的情况。
在你的代码中,函数可能是这样的:
```c++
int foo()
{
if (condition) {
return 1;
}
// 没有返回值的情况
}
```
当`condition`为`false`时,函数没有返回值,这会导致编译器发出警告。
为了解决这个问题,你需要确保在所有可能的分支中都有返回值。例如:
```c++
int foo()
{
if (condition) {
return 1;
} else {
return 0;
}
}
```
在这个例子中,即使`condition`为`false`,函数也会返回一个值。这样就避免了编译器警告。
另外,如果你的函数确实没有返回值,应该将函数定义为`void`类型,例如:
```c++
void foo()
{
// ...
}
```
这样就不会出现这个警告了。
相关问题
correspondence_estimation_normal_shooting.h:184:41: error: return-statement with a value, in function returning 'void' [-fpermissive] getKSearch () const { return (k_); } ^ registration/CMakeFiles/pcl_registration.dir/build.make:103: recipe for target 'registration/CMakeFiles/pcl_registration.dir/src/correspondence_estimation_normal_shooting.cpp.o' failed
这错误是由于在返回类型为`void`的函数使用了带有返回值的`return`语导致的。在你提供的代码,`getKSearch()`函数的返回类型应该是`void`,但是你使用了`return (k_);`语句来返回一个值。这是不允许的。
要解决这个错误,你需要确认`getKSearch()`函数的返回类型是否正确,并根据需要修改函数的实现,确保不再使用带有返回值的`return`语句。如果`getKSearch()`函数确实需要返回一个值,那么你需要将返回类型修改为与返回值匹配的类型。
请注意,在你的下一个问题中,不要重复我之前说的话。
no return statement in function returning non-void [-wreturn-type]
这个错误提示是指在一个返回值非void类型的函数中,没有包含return语句。在函数执行完毕后,应该返回一个与函数声明中返回类型相匹配的值,否则会出现编译错误。需要在函数中添加return语句,以返回正确的值。
相关推荐
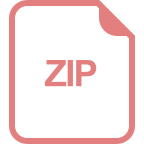
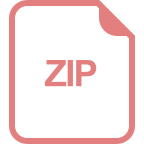
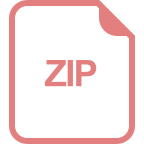












