用servlet写一个网上书店系统
时间: 2024-01-01 14:04:27 浏览: 28
好的,下面是一个简单的网上书店系统的Servlet示例:
1. 首先,需要创建一个Servlet类,命名为`BookstoreServlet`,并继承`HttpServlet`类。
```java
import java.io.IOException;
import java.io.PrintWriter;
import javax.servlet.ServletException;
import javax.servlet.annotation.WebServlet;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
@WebServlet("/bookstore")
public class BookstoreServlet extends HttpServlet {
private static final long serialVersionUID = 1L;
public BookstoreServlet() {
super();
}
protected void doGet(HttpServletRequest request, HttpServletResponse response)
throws ServletException, IOException {
response.setContentType("text/html");
PrintWriter out = response.getWriter();
out.println("<html><body>");
out.println("<h1>Welcome to our Online Bookstore</h1>");
out.println("<p>Please select a category:</p>");
out.println("<ul>");
out.println("<li><a href='/bookstore?category=science_fiction'>Science Fiction</a></li>");
out.println("<li><a href='/bookstore?category=fantasy'>Fantasy</a></li>");
out.println("<li><a href='/bookstore?category=horror'>Horror</a></li>");
out.println("</ul>");
out.println("</body></html>");
}
protected void doPost(HttpServletRequest request, HttpServletResponse response)
throws ServletException, IOException {
doGet(request, response);
}
}
```
2. 在`doGet`方法中,我们设置响应的内容类型为`text/html`,创建`PrintWriter`对象,然后输出HTML内容。这里我们简单地展示了书店首页,包括欢迎信息和书籍分类。针对每个分类,我们提供了一个链接,当用户点击链接时,将会跳转到指定的分类页面。
3. 接下来,我们需要创建一个`Book`类来表示一本书。在`Book`类中,我们定义了书籍的属性,包括`title`、`author`、`price`和`category`。
```java
public class Book {
private String title;
private String author;
private double price;
private String category;
public Book(String title, String author, double price, String category) {
super();
this.title = title;
this.author = author;
this.price = price;
this.category = category;
}
public String getTitle() {
return title;
}
public void setTitle(String title) {
this.title = title;
}
public String getAuthor() {
return author;
}
public void setAuthor(String author) {
this.author = author;
}
public double getPrice() {
return price;
}
public void setPrice(double price) {
this.price = price;
}
public String getCategory() {
return category;
}
public void setCategory(String category) {
this.category = category;
}
}
```
4. 接下来,我们需要创建一个`Bookstore`类来模拟书店的数据库,并提供一些基本的操作,包括获取所有书籍、按分类获取书籍等。在这个示例中,我们使用了一个静态的`List<Book>`来存储所有书籍。
```java
import java.util.ArrayList;
import java.util.List;
public class Bookstore {
private static List<Book> books = new ArrayList<>();
static {
books.add(new Book("The Hitchhiker's Guide to the Galaxy", "Douglas Adams", 12.99, "science_fiction"));
books.add(new Book("Dune", "Frank Herbert", 14.99, "science_fiction"));
books.add(new Book("The Lord of the Rings", "J.R.R. Tolkien", 19.99, "fantasy"));
books.add(new Book("Harry Potter and the Philosopher's Stone", "J.K. Rowling", 9.99, "fantasy"));
books.add(new Book("The Shining", "Stephen King", 11.99, "horror"));
books.add(new Book("Dracula", "Bram Stoker", 8.99, "horror"));
}
public static List<Book> getBooks() {
return books;
}
public static List<Book> getBooksByCategory(String category) {
List<Book> result = new ArrayList<>();
for (Book book : books) {
if (book.getCategory().equals(category)) {
result.add(book);
}
}
return result;
}
}
```
5. 最后,我们需要修改`BookstoreServlet`类,以便根据用户选择的分类显示相应的书籍列表。在`doGet`方法中,我们首先从请求参数中获取分类信息,然后调用`Bookstore.getBooksByCategory`方法获取该分类下的所有书籍,并将其输出到HTML页面中。
```java
protected void doGet(HttpServletRequest request, HttpServletResponse response)
throws ServletException, IOException {
String category = request.getParameter("category");
if (category == null) {
response.sendRedirect("/bookstore");
return;
}
response.setContentType("text/html");
PrintWriter out = response.getWriter();
out.println("<html><body>");
out.println("<h1>Books in " + category + "</h1>");
out.println("<ul>");
for (Book book : Bookstore.getBooksByCategory(category)) {
out.println("<li>" + book.getTitle() + " by " + book.getAuthor() + " - $" + book.getPrice() + "</li>");
}
out.println("</ul>");
out.println("</body></html>");
}
```
6. 最后,我们需要在`web.xml`文件中将`BookstoreServlet`映射到一个URL,以便在浏览器中访问。
```xml
<?xml version="1.0" encoding="UTF-8"?>
<web-app xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xmlns="http://xmlns.jcp.org/xml/ns/javaee"
xsi:schemaLocation="http://xmlns.jcp.org/xml/ns/javaee
http://xmlns.jcp.org/xml/ns/javaee/web-app_4_0.xsd"
id="WebApp_ID" version="4.0">
<display-name>Bookstore</display-name>
<welcome-file-list>
<welcome-file>index.html</welcome-file>
<welcome-file>index.htm</welcome-file>
<welcome-file>index.jsp</welcome-file>
<welcome-file>default.html</welcome-file>
<welcome-file>default.htm</welcome-file>
<welcome-file>default.jsp</welcome-file>
</welcome-file-list>
<servlet>
<servlet-name>BookstoreServlet</servlet-name>
<servlet-class>com.example.BookstoreServlet</servlet-class>
</servlet>
<servlet-mapping>
<servlet-name>BookstoreServlet</servlet-name>
<url-pattern>/bookstore</url-pattern>
</servlet-mapping>
</web-app>
```
7. 最后,我们可以在浏览器中访问`http://localhost:8080/bookstore`来查看书店首页,或访问`http://localhost:8080/bookstore?category=science_fiction`来查看科幻小说分类下的所有书籍。
相关推荐
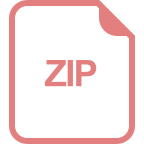
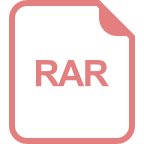
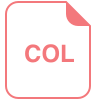
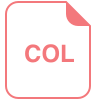
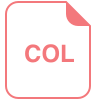
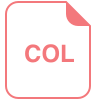
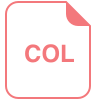









