linux中,使用mmap函数,实现对文件的读写操作,程序实现以下功能: (1)使用cp命令,将/etc/passwd文件复制到/tmp目录下。 cp /etc/passwd /tmp/
时间: 2023-08-19 08:04:05 浏览: 121
使用mmap函数实现对文件的读写操作可以分为以下几个步骤:
1. 打开源文件和目标文件
```c
int src_fd = open("/etc/passwd", O_RDONLY);
int dest_fd = open("/tmp/passwd", O_RDWR | O_CREAT, 0666);
```
2. 获取源文件大小
```c
struct stat s;
fstat(src_fd, &s);
off_t filesize = s.st_size;
```
3. 将目标文件扩展到与源文件一样大
```c
ftruncate(dest_fd, filesize);
```
4. 使用mmap函数将源文件和目标文件映射到内存中
```c
char *src_ptr = mmap(NULL, filesize, PROT_READ, MAP_PRIVATE, src_fd, 0);
char *dest_ptr = mmap(NULL, filesize, PROT_READ | PROT_WRITE, MAP_SHARED, dest_fd, 0);
```
5. 将源文件的内容复制到目标文件中
```c
memcpy(dest_ptr, src_ptr, filesize);
```
6. 取消文件映射并关闭文件
```c
munmap(src_ptr, filesize);
munmap(dest_ptr, filesize);
close(src_fd);
close(dest_fd);
```
完整代码如下所示:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <sys/mman.h>
#include <sys/stat.h>
#include <fcntl.h>
#include <unistd.h>
int main() {
// 打开源文件和目标文件
int src_fd = open("/etc/passwd", O_RDONLY);
int dest_fd = open("/tmp/passwd", O_RDWR | O_CREAT, 0666);
if (src_fd == -1 || dest_fd == -1) {
perror("open");
exit(1);
}
// 获取源文件大小
struct stat s;
fstat(src_fd, &s);
off_t filesize = s.st_size;
// 将目标文件扩展到与源文件一样大
ftruncate(dest_fd, filesize);
// 使用mmap函数将源文件和目标文件映射到内存中
char *src_ptr = mmap(NULL, filesize, PROT_READ, MAP_PRIVATE, src_fd, 0);
char *dest_ptr = mmap(NULL, filesize, PROT_READ | PROT_WRITE, MAP_SHARED, dest_fd, 0);
if (src_ptr == MAP_FAILED || dest_ptr == MAP_FAILED) {
perror("mmap");
exit(1);
}
// 将源文件的内容复制到目标文件中
memcpy(dest_ptr, src_ptr, filesize);
// 取消文件映射并关闭文件
munmap(src_ptr, filesize);
munmap(dest_ptr, filesize);
close(src_fd);
close(dest_fd);
printf("Copy /etc/passwd to /tmp/passwd successfully.\n");
return 0;
}
```
执行程序后,可以使用ls命令查看/tmp目录下是否已经存在passwd文件。
阅读全文
相关推荐
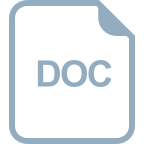
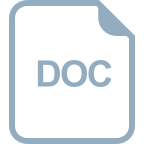










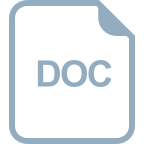

