SpringBoot全栈项目实战
时间: 2023-11-19 15:42:37 浏览: 39
SpringBoot是一个非常流行的Java框架,它可以快速搭建一个Web应用程序。下面是一个简单的全栈项目实战,使用SpringBoot、MySQL、MyBatis、Thymeleaf和Bootstrap等技术。
首先,创建一个SpringBoot项目,引入相关依赖。在这个项目中,我们将使用MySQL数据库,因此需要引入MySQL依赖。在pom.xml文件中添加以下依赖:
```xml
<dependencies>
<!--SpringBoot Web-->
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<!--MySQL-->
<dependency>
<groupId>mysql</groupId>
<artifactId>mysql-connector-java</artifactId>
</dependency>
<!--MyBatis-->
<dependency>
<groupId>org.mybatis.spring.boot</groupId>
<artifactId>mybatis-spring-boot-starter</artifactId>
<version>2.2.0</version>
</dependency>
<!--Thymeleaf-->
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-thymeleaf</artifactId>
</dependency>
<!--Bootstrap-->
<dependency>
<groupId>org.webjars</groupId>
<artifactId>bootstrap</artifactId>
<version>5.0.0-beta1</version>
</dependency>
</dependencies>
```
接下来,创建一个数据库表。在MySQL中创建一个名为“student”的表,包含id、name和age三个字段。
```sql
CREATE TABLE student (
id INT(11) NOT NULL AUTO_INCREMENT PRIMARY KEY,
name VARCHAR(50) NOT NULL,
age INT(11) NOT NULL
) ENGINE=InnoDB DEFAULT CHARSET=utf8;
```
然后,创建一个实体类Student,包含id、name和age三个属性。
```java
public class Student {
private Integer id;
private String name;
private Integer age;
// 省略getter和setter方法
}
```
接下来,创建一个MyBatis的Mapper接口,用于定义SQL语句。创建一个名为StudentMapper的接口,包含查询所有学生、插入学生和删除学生三个方法。
```java
@Mapper
public interface StudentMapper {
@Select("SELECT * FROM student")
List<Student> findAll();
@Insert("INSERT INTO student(name, age) VALUES(#{name}, #{age})")
int insert(Student student);
@Delete("DELETE FROM student WHERE id=#{id}")
int deleteById(Integer id);
}
```
然后,创建一个Service层,用于调用Mapper接口。创建一个名为StudentService的类,包含查询所有学生、插入学生和删除学生三个方法。在这个类中,我们将使用@Autowired注解自动注入StudentMapper接口。
```java
@Service
public class StudentService {
@Autowired
private StudentMapper studentMapper;
public List<Student> findAll() {
return studentMapper.findAll();
}
public int insert(Student student) {
return studentMapper.insert(student);
}
public int deleteById(Integer id) {
return studentMapper.deleteById(id);
}
}
```
接下来,创建一个Controller层,用于处理HTTP请求。创建一个名为StudentController的类,包含查询所有学生、插入学生和删除学生三个方法。
```java
@Controller
public class StudentController {
@Autowired
private StudentService studentService;
@GetMapping("/")
public String index(Model model) {
List<Student> students = studentService.findAll();
model.addAttribute("students", students);
return "index";
}
@PostMapping("/add")
public String add(Student student) {
studentService.insert(student);
return "redirect:/";
}
@GetMapping("/delete/{id}")
public String delete(@PathVariable Integer id) {
studentService.deleteById(id);
return "redirect:/";
}
}
```
最后,创建一个Thymeleaf模板,用于展示学生数据。在src/main/resources/templates目录下创建一个名为index.html的文件,包含一个表格和一个表单。
```html
<!DOCTYPE html>
<html xmlns:th="http://www.thymeleaf.org">
<head>
<meta charset="UTF-8">
<title>SpringBoot全栈项目实战</title>
<link rel="stylesheet" href="/webjars/bootstrap/5.0.0-beta1/css/bootstrap.min.css">
</head>
<body>
<div class="container">
<h1>学生信息</h1>
<table class="table table-striped">
<thead>
<tr>
<th>ID</th>
<th>姓名</th>
<th>年龄</th>
<th>操作</th>
</tr>
</thead>
<tbody>
<tr th:each="student : ${students}">
<td th:text="${student.id}"></td>
<td th:text="${student.name}"></td>
<td th:text="${student.age}"></td>
<td><a th:href="@{/delete/{id}(id=${student.id})}">删除</a></td>
</tr>
</tbody>
</table>
<form method="post" th:action="@{/add}">
<div class="form-group">
<label for="name">姓名:</label>
<input type="text" class="form-control" id="name" name="name" required>
</div>
<div class="form-group">
<label for="age">年龄:</label>
<input type="number" class="form-control" id="age" name="age" required>
</div>
<button type="submit" class="btn btn-primary">添加</button>
</form>
</div>
</body>
</html>
```
这个项目就完成了。启动项目,在浏览器中访问http://localhost:8080/,就可以看到学生信息的表格和表单。在表单中输入学生信息并提交,就可以添加一条学生记录。在表格中点击“删除”按钮,就可以删除一条学生记录。
相关推荐
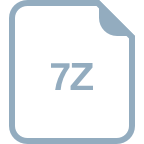
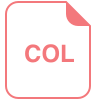
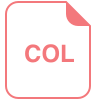
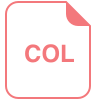
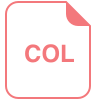










