多线程插入
时间: 2023-06-28 20:07:10 浏览: 77
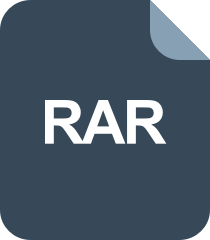
多线程插入技术
多线程插入可以提高插入数据的效率,下面是一个示例代码:
```python
import threading
import pymysql
class InsertThread(threading.Thread):
def __init__(self, db, data):
super().__init__()
self.db = db
self.data = data
def run(self):
conn = pymysql.connect(host='localhost', user='root', password='password', db=self.db)
cursor = conn.cursor()
sql = "INSERT INTO table_name (column1, column2, column3) VALUES (%s, %s, %s)"
try:
cursor.executemany(sql, self.data)
conn.commit()
except Exception as e:
conn.rollback()
print(e)
finally:
cursor.close()
conn.close()
if __name__ == '__main__':
db_name = 'test'
data_list = [[1, 'a', 10], [2, 'b', 20], [3, 'c', 30], [4, 'd', 40], [5, 'e', 50]]
thread_list = []
for i in range(0, len(data_list), 2):
t = InsertThread(db_name, data_list[i:i+2])
thread_list.append(t)
for t in thread_list:
t.start()
for t in thread_list:
t.join()
```
该代码使用了多线程方式插入数据,其中每个线程处理两个数据,可以根据数据量和机器配置进行调整。当有大量数据需要插入时,使用多线程可以显著提高效率。
阅读全文
相关推荐



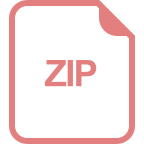
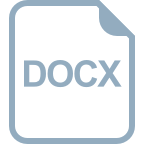
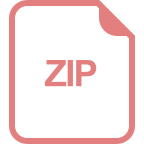
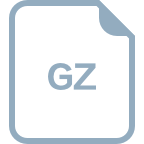




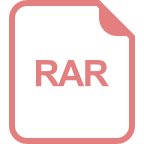
