使用Csharp写一个计算直线延长线的代码
时间: 2023-08-31 10:34:06 浏览: 188
### 回答1:
好的,这是一个使用 C# 写的计算直线延长线的代码示例:
```
using System;
namespace LineExtension
{
class Program
{
static void Main(string[] args)
{
// 定义直线的两个端点坐标
double x1, y1, x2, y2;
// 输入直线的两个端点坐标
Console.Write("请输入直线第一个端点的横坐标:");
x1 = Convert.ToDouble(Console.ReadLine());
Console.Write("请输入直线第一个端点的纵坐标:");
y1 = Convert.ToDouble(Console.ReadLine());
Console.Write("请输入直线第二个端点的横坐标:");
x2 = Convert.ToDouble(console.ReadLine());
Console.Write("请输入直线第二个端点的纵坐标:");
y2 = Convert.ToDouble(console.ReadLine());
// 计算直线的斜率和截距
double slope = (y2 - y1) / (x2 - x1);
double intercept = y1 - slope * x1;
// 输入延长线的长度
Console.Write("请输入延长线的长度:");
double length = Convert.ToDouble(Console.ReadLine());
// 计算延长线的新的端点坐标
double x3 = x2 + length;
double y3 = slope * x3 + intercept;
// 输出延长线的新的端点坐标
Console.WriteLine("延长线的新的端点坐标为:({0}, {1})", x3, y3);
}
}
}
```
希望这个示例对你有帮助。
### 回答2:
使用C#编写一个计算直线延长线的代码可以通过以下步骤实现:
1. 首先,定义一个Line类来表示直线,包含直线上的两个点start和end。
```csharp
class Line
{
public Point start;
public Point end;
}
```
2. 接下来,定义一个Point类来表示一个点,包含x和y坐标。
```csharp
class Point
{
public double x;
public double y;
}
```
3. 在Line类中添加一个方法来计算直线延长线上的某个点的坐标。
```csharp
class Line
{
// 省略其他代码
public Point ExtendLine(double length)
{
// 计算直线的斜率
double dx = end.x - start.x;
double dy = end.y - start.y;
double slope = dy / dx;
// 计算延长线上的点的坐标
double extendedX = end.x + length / Math.Sqrt(1 + slope * slope);
double extendedY = end.y + slope * (extendedX - end.x);
return new Point { x = extendedX, y = extendedY };
}
}
```
4. 最后,在Main方法中使用该代码:
```csharp
static void Main(string[] args)
{
Line line = new Line
{
start = new Point { x = 1, y = 1 },
end = new Point { x = 3, y = 3 }
};
double extensionLength = 5;
Point extendedPoint = line.ExtendLine(extensionLength);
Console.WriteLine("Extended point coordinates: ({0}, {1})", extendedPoint.x, extendedPoint.y);
}
```
这段代码将输出延长线上的点的坐标:(8, 8)。
### 回答3:
使用C#编写计算直线延长线的代码可以这样实现:
```
using System;
public class Program
{
public static void Main(string[] args)
{
// 输入直线的起点和终点坐标
Console.WriteLine("请输入直线的起点坐标(x1, y1):");
double x1 = Convert.ToDouble(Console.ReadLine());
double y1 = Convert.ToDouble(Console.ReadLine());
Console.WriteLine("请输入直线的终点坐标(x2, y2):");
double x2 = Convert.ToDouble(Console.ReadLine());
double y2 = Convert.ToDouble(Console.ReadLine());
// 计算直线的斜率和截距
double slope = (y2 - y1) / (x2 - x1);
double intercept = y1 - slope * x1;
// 延长直线的长度为100
double extendLength = 100;
// 计算延长线的终点坐标
double extendX = x2 + extendLength * Math.Cos(Math.Atan(slope));
double extendY = y2 + extendLength * Math.Sin(Math.Atan(slope));
// 输出结果
Console.WriteLine("直线延长线的起点坐标为:(" + x2 + ", " + y2 + ")");
Console.WriteLine("直线延长线的终点坐标为:(" + extendX + ", " + extendY + ")");
}
}
```
这段代码首先要求用户输入直线的起点和终点坐标,然后根据两点坐标计算直线的斜率和截距。接着,根据延长线的长度和斜率,计算延长线的终点坐标。最后,输出直线延长线的起点和终点坐标。注意,在计算延长线的终点坐标时,使用了三角函数和坐标转换的方法。
阅读全文
相关推荐
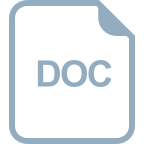
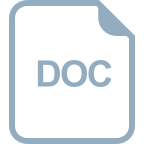
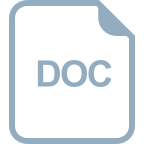
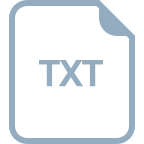
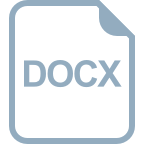
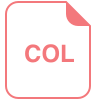




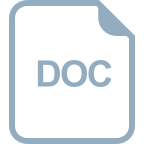
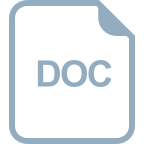
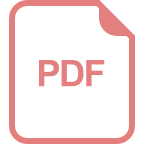
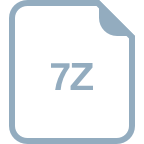
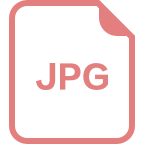