用python写一个处理时间日期得控件
时间: 2024-05-11 18:16:45 浏览: 6
很高兴为您解答,请问您需要一个日期选择器吗?
如果是日期选择器,可以使用 Python 内置的 datetime 和 tkinter 库来创建一个简单的应用程序。以下是一个示例代码:
```python
import tkinter as tk
from datetime import datetime
class DatePicker(tk.Frame):
def __init__(self, master=None):
super().__init__(master)
self.pack()
self.date_label = tk.Label(self, text='请选一个日期:')
self.date_label.pack()
# 年份下拉框
self.year_label = tk.Label(self, text='年份:')
self.year_label.pack(side='left')
self.years = [str(year) for year in range(1900, 2050)]
self.year_combobox = tk.ttk.Combobox(self, width=6, values=self.years, state='readonly')
self.year_combobox.set(datetime.today().year) # 设置默认值为当前年份
self.year_combobox.pack(side='left')
# 月份下拉框
self.month_label = tk.Label(self, text='月份:')
self.month_label.pack(side='left')
self.months = [str(month) for month in range(1, 13)]
self.month_combobox = tk.ttk.Combobox(self, width=4, values=self.months, state='readonly')
self.month_combobox.set(datetime.today().month) # 设置默认值为当前月份
self.month_combobox.pack(side='left')
# 日子下拉框
self.day_label = tk.Label(self, text='日期:')
self.day_label.pack(side='left')
self.days = [str(day) for day in range(1, 32)]
self.day_combobox = tk.ttk.Combobox(self, width=4, values=self.days, state='readonly')
self.day_combobox.set(datetime.today().day) # 设置默认值为当前日期
self.day_combobox.pack(side='left')
# 星期显示框
self.weekday_label = tk.Label(self, text='')
self.weekday_label.pack()
# 确认按钮
self.ok_button = tk.Button(self, text='确定', command=self.on_ok_button_click)
self.ok_button.pack()
def on_ok_button_click(self):
year = int(self.year_combobox.get())
month = int(self.month_combobox.get())
day = int(self.day_combobox.get())
date = datetime(year, month, day)
weekday = date.strftime("%A") # 星期几的简写
self.weekday_label.configure(text=f'{year}-{month}-{day}是{weekday}')
if __name__ == '__main__':
root = tk.Tk()
root.title('日期选择器')
root.geometry('300x120')
date_picker = DatePicker(root)
date_picker.mainloop()
```
这是一个基于 tkinter 的 GUI 应用程序,实现了年月日三个下拉框,用户可以选择一个日期后点击确定按钮,程序会显示这个日期对应的星期几。
注意,上述示例代码仅供参考,还有很多细节需要完善,例如输入非法日期时的错误处理、界面美化等。
相关推荐
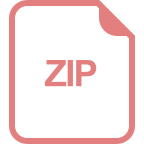
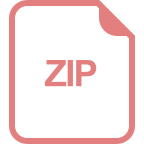














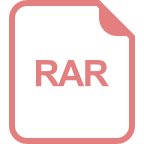