pytroch resnet-50 图像分类
时间: 2023-09-21 12:10:47 浏览: 53
要使用 PyTorch 中的 ResNet-50 进行图像分类,你需要按照以下步骤进行操作:
1. 导入必要的库
```python
import torch
import torch.nn as nn
import torchvision.models as models
import torchvision.transforms as transforms
import PIL.Image as Image
```
2. 加载预训练的 ResNet-50 模型
```python
model = models.resnet50(pretrained=True)
```
3. 冻结模型的参数(可选)
```python
for param in model.parameters():
param.requires_grad = False
```
4. 更改最后一层全连接层的输出大小以匹配你的数据集
```python
num_features = model.fc.in_features
model.fc = nn.Linear(num_features, num_classes)
```
其中,`num_classes` 是你的数据集中的类别数。
5. 定义数据预处理函数
```python
preprocess = transforms.Compose([
transforms.Resize(256),
transforms.CenterCrop(224),
transforms.ToTensor(),
transforms.Normalize(
mean=[0.485, 0.456, 0.406],
std=[0.229, 0.224, 0.225]
)
])
```
6. 加载测试图像并进行预处理
```python
image = Image.open('test_image.jpg')
image = preprocess(image)
```
7. 将图像传入模型进行预测
```python
model.eval()
with torch.no_grad():
output = model(image.unsqueeze(0))
_, predicted = torch.max(output.data, 1)
```
其中,`predicted` 是预测的类别。
这就是使用 PyTorch 中的 ResNet-50 进行图像分类的基本步骤。当然,在实际应用中,你可能还需要进行更多的调整和优化。
相关推荐
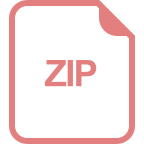
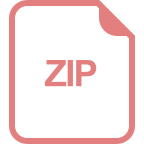
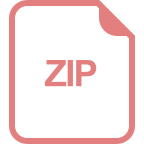


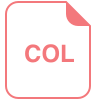
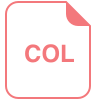
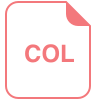









