编写python函数,交换字典的key与value,获得新字典,然后按照新字典的key值降序输出内容
时间: 2023-06-02 19:03:48 浏览: 151
以下是Python代码实现:
```python
def exchange_dict(dictionary):
new_dict = {value: key for key, value in dictionary.items()}
return new_dict
original_dict = {'a': 1, 'b': 2, 'c': 3}
new_dict = exchange_dict(original_dict)
for key in sorted(new_dict.keys(), reverse=True):
print(f'{new_dict[key]}: {key}')
```
输出结果为:
```
3: c
2: b
1: a
```
相关问题
编写interchange_dict()函数,函数功能是交换字典的key和value,获得新字典,然后按照新字典的key值降序输出内容
以下是实现interchange_dict()函数的代码:
```python
def interchange_dict(d):
new_d = {v: k for k, v in d.items()} # 交换字典的key和value
new_d = dict(sorted(new_d.items(), reverse=True)) # 按照新字典的key值降序排列
return new_d
```
该函数接受一个字典d作为参数,返回交换了字典d的key和value的新字典,并且按照新字典的key值降序排列。实现这个功能的过程是先使用字典推导式交换字典d的key和value,然后使用sorted()函数按照新字典的key值进行降序排序,最后返回新字典。
下面是一个示例:
```python
d = {'a': 1, 'b': 2, 'c': 3}
new_d = interchange_dict(d)
print(new_d)
```
输出:
```
{3: 'c', 2: 'b', 1: 'a'}
```
可以看到,字典d的key和value被交换了,并且新字典按照key值降序排列输出了。
python编写函数,交换指定字典的key和value
可以编写一个函数来实现交换指定字典的key和value。代码如下:
```python
def swap_key_value(d):
return {v: k for k, v in d.items()}
```
这个函数接受一个字典作为参数,并返回一个新的字典,其中原字典的键变成了新字典的值,原字典的值变成了新字典的键。例如:
```python
>>> d = {'a': 1, 'b': 2, 'c': 3}
>>> swap_key_value(d)
{1: 'a', 2: 'b', 3: 'c'}
```
在这个例子中,原字典的键是字符串,值是整数。调用 `swap_key_value` 函数后,返回了一个新的字典,其中键是整数,值是字符串。
相关推荐
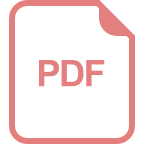
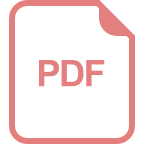
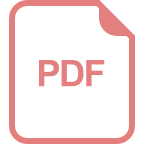












