import cv2 class VideoCamera(object): url = "rtsp://admin:mliszlm79+-12@192.168.0.108:554/cam/realmonitor?channel=1&subtype=0" def __init__(self): #capturing video self.video = cv2.VideoCapture(self.url) def __del__(self): #releasing camera self.video.release()
时间: 2023-06-04 22:07:02 浏览: 238

rtsp-1.0.1.tar.gz_C++ RTSP/RTP_RTSP c++_RTSP 代码_rtsp-1.0.1.tar_r

这段代码定义了一个名为VideoCamera的对象类,其中import了cv2库用于图像处理。在类内部定义了一个常量url,被赋值为一个RTSP视频流地址,这个地址需要连接到指定的IP摄像头,并提供相应的用户名和密码。视频流地址的最后一个参数指明了要连接的视频通道序号。
阅读全文
相关推荐
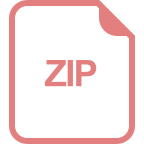
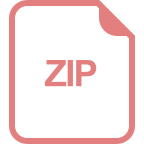
















