excel如何使用VBA实现下拉列表模糊搜索
时间: 2023-07-20 14:14:01 浏览: 353
实现下拉列表模糊搜索的方法如下:
1. 首先,在Excel中创建一个下拉列表。
2. 在VBA中创建一个函数,该函数将返回一个字符串类型的数组,该数组包含与用户输入的搜索字符串匹配的所有下拉列表项。
3. 在下拉列表的输入单元格上使用数据验证功能,并为此单元格指定数据验证列表,该列表使用VBA函数返回的数组。
4. 当用户在输入单元格中输入字符时,应该自动更新下拉列表,以显示与输入字符串匹配的所有项。
以下是一个示例代码,可以实现上述功能:
```VBA
Option Explicit
Function FilterData(sht As Worksheet, searchStr As String, _
filterRange As Range) As String()
Dim arrData() As String
Dim i As Long, j As Long, k As Long
Dim matchFound As Boolean
'Initialize the array
ReDim arrData(1 To filterRange.Rows.Count)
'Loop through each cell in the filter range
For i = 1 To filterRange.Rows.Count
matchFound = False
'Check if the current cell contains the search string
If InStr(1, filterRange.Cells(i, 1).Value, searchStr, vbTextCompare) > 0 Then
matchFound = True
End If
'If a match is found, add it to the array
If matchFound Then
k = k + 1
arrData(k) = filterRange.Cells(i, 1).Value
End If
Next i
'Resize the array to remove any empty elements
If k > 0 Then
ReDim Preserve arrData(1 To k)
Else
ReDim arrData(1 To 1)
arrData(1) = ""
End If
'Sort the array
Call QuickSort(arrData, LBound(arrData), UBound(arrData))
'Return the filtered data
FilterData = arrData
End Function
Sub UpdateDataValidation()
Dim sht As Worksheet
Dim searchStr As String
Dim filterRange As Range
Dim arrData() As String
Dim i As Long
'Get the active sheet
Set sht = ActiveSheet
'Get the search string from the input cell
searchStr = sht.Range("A1").Value
'Get the filter range
Set filterRange = sht.Range("B1:B10")
'Filter the data based on the search string
arrData = FilterData(sht, searchStr, filterRange)
'Clear the existing data validation
With sht.Range("A2").Validation
.Delete
End With
'Add the new data validation
With sht.Range("A2").Validation
.Add Type:=xlValidateList, AlertStyle:=xlValidAlertStop, _
Formula1:=Join(arrData, ",")
End With
End Sub
Sub QuickSort(arr() As String, left As Long, right As Long)
Dim i As Long
Dim j As Long
Dim temp As String
Dim pivot As String
i = left
j = right
pivot = arr((left + right) \ 2)
While i <= j
While arr(i) < pivot And i < right
i = i + 1
Wend
While pivot < arr(j) And j > left
j = j - 1
Wend
If i <= j Then
temp = arr(i)
arr(i) = arr(j)
arr(j) = temp
i = i + 1
j = j - 1
End If
Wend
If left < j Then Call QuickSort(arr, left, j)
If i < right Then Call QuickSort(arr, i, right)
End Sub
```
这个示例代码将会在当前工作表中,使用单元格 A1 作为搜索字符串的输入单元格,使用单元格 A2 作为下拉列表的输入单元格,使用单元格 B1:B10 作为下拉列表的数据源。您可以根据需要修改这些参数。
阅读全文
相关推荐
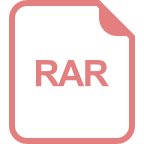



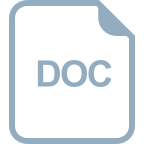
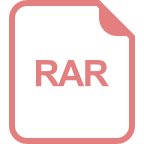
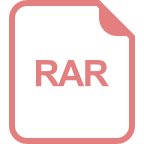
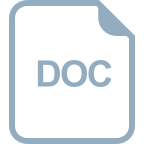
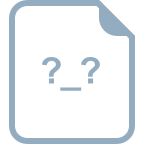
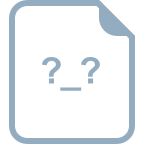
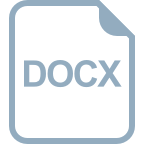
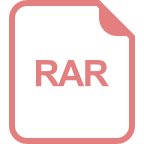
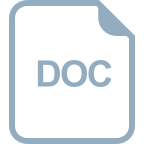
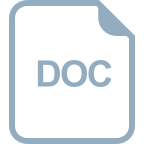
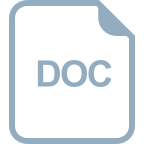
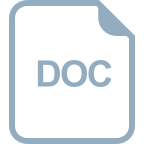
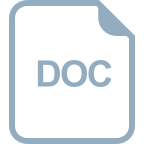