写一个图书管理系统,链表方式存储,c语言实现插入,删除功能
时间: 2023-06-09 11:03:50 浏览: 50
好的,以下是实现步骤:
1. 定义图书结构体,包含图书编号、名称、作者、出版社等信息。
2. 定义链表结构体,包含指向下一本图书的指针和指向前一本图书的指针。
3. 定义链表头指针和链表尾指针,初始值均为 NULL。
4. 实现插入函数,将图书信息插入链表中,并保持链表有序。
5. 实现删除函数,可以根据图书编号或图书名称进行删除操作。
6. 实现打印函数,可以将链表中的所有图书信息打印出来,方便用户查看。
7. 在主函数中实现菜单功能,包括添加图书、删除图书和打印图书列表等选项。
具体实现请参考下面的代码示例:
```
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
typedef struct _book
{
int id;
char name[50];
char author[50];
char publisher[50];
} Book;
typedef struct _node
{
Book book;
struct _node *prev;
struct _node *next;
} Node;
Node *head = NULL;
Node *tail = NULL;
void insert(Book book)
{
Node *new_node = (Node *)malloc(sizeof(Node));
new_node->book = book;
new_node->prev = NULL;
new_node->next = NULL;
if (head == NULL)
{
head = new_node;
tail = new_node;
return;
}
Node *prev_node = NULL;
Node *curr_node = head;
while (curr_node != NULL && strcmp(curr_node->book.name, book.name) < 0)
{
prev_node = curr_node;
curr_node = curr_node->next;
}
if (prev_node == NULL)
{
new_node->next = head;
head->prev = new_node;
head = new_node;
}
else if (curr_node == NULL)
{
new_node->prev = tail;
tail->next = new_node;
tail = new_node;
}
else
{
new_node->prev = prev_node;
new_node->next = curr_node;
prev_node->next = new_node;
curr_node->prev = new_node;
}
}
void delete_by_id(int id)
{
Node *prev_node = NULL;
Node *curr_node = head;
while (curr_node != NULL && curr_node->book.id != id)
{
prev_node = curr_node;
curr_node = curr_node->next;
}
if (curr_node == NULL)
{
printf("No book with id %d found.\n", id);
return;
}
if (prev_node == NULL)
{
head = curr_node->next;
if (head == NULL)
tail = NULL;
else
head->prev = NULL;
}
else if (curr_node->next == NULL)
{
tail = prev_node;
tail->next = NULL;
}
else
{
prev_node->next = curr_node->next;
curr_node->next->prev = prev_node;
}
free(curr_node);
printf("Book with id %d deleted.\n", id);
}
void delete_by_name(char *name)
{
Node *prev_node = NULL;
Node *curr_node = head;
while (curr_node != NULL && strcmp(curr_node->book.name, name) != 0)
{
prev_node = curr_node;
curr_node = curr_node->next;
}
if (curr_node == NULL)
{
printf("No book with name \"%s\" found.\n", name);
return;
}
if (prev_node == NULL)
{
head = curr_node->next;
if (head == NULL)
tail = NULL;
else
head->prev = NULL;
}
else if (curr_node->next == NULL)
{
tail = prev_node;
tail->next = NULL;
}
else
{
prev_node->next = curr_node->next;
curr_node->next->prev = prev_node;
}
free(curr_node);
printf("Book with name \"%s\" deleted.\n", name);
}
void print_all()
{
Node *curr_node = head;
while (curr_node != NULL)
{
printf("Id: %d, Name: %s, Author: %s, Publisher: %s\n",
curr_node->book.id, curr_node->book.name,
curr_node->book.author, curr_node->book.publisher);
curr_node = curr_node->next;
}
}
int main()
{
while (1)
{
printf("Menu:\n");
printf("1. Add book.\n");
printf("2. Delete book by id.\n");
printf("3. Delete book by name.\n");
printf("4. Print all books.\n");
printf("5. Exit.\n");
printf("Please select an option:");
int option;
scanf("%d", &option);
switch (option)
{
case 1:
{
Book book;
printf("Please enter book id:");
scanf("%d", &book.id);
printf("Please enter book name:");
scanf("%s", book.name);
printf("Please enter book author:");
scanf("%s", book.author);
printf("Please enter book publisher:");
scanf("%s", book.publisher);
insert(book);
printf("Book added successfully.\n");
break;
}
case 2:
{
int id;
printf("Please enter book id to be deleted:");
scanf("%d", &id);
delete_by_id(id);
break;
}
case 3:
{
char name[50];
printf("Please enter book name to be deleted:");
scanf("%s", name);
delete_by_name(name);
break;
}
case 4:
{
print_all();
break;
}
case 5:
{
return 0;
}
default:
{
printf("Invalid option selected, please try again.\n");
break;
}
}
}
return 0;
}
```
相关推荐
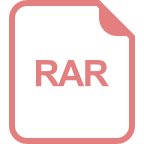
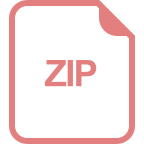















