linux c语言初始化485口
时间: 2023-07-26 07:32:38 浏览: 61
要在 Linux C 语言中初始化 485 口,可以通过以下步骤实现:
1. 打开串口设备文件,例如 /dev/ttyS1。
2. 通过 ioctl 系统调用设置串口属性,包括波特率、数据位、停止位、奇偶校验等。对于 485 口,需要使用 RS485 模式,可以通过设置 RTS 和 CTS 的状态控制传输方向。
3. 可以使用 write 函数向串口发送数据,使用 read 函数从串口读取数据。
以下是一个简单的示例代码:
```
#include <stdio.h>
#include <fcntl.h>
#include <termios.h>
#include <unistd.h>
#include <sys/ioctl.h>
int main()
{
int fd = open("/dev/ttyS1", O_RDWR);
if (fd < 0) {
printf("Failed to open serial port\n");
return -1;
}
struct termios options;
tcgetattr(fd, &options);
cfsetispeed(&options, B9600);
cfsetospeed(&options, B9600);
options.c_cflag |= CLOCAL | CREAD;
options.c_cflag &= ~CSIZE;
options.c_cflag |= CS8;
options.c_cflag &= ~PARENB;
options.c_cflag &= ~CSTOPB;
options.c_cflag &= ~CRTSCTS;
options.c_cflag |= CREAD | CLOCAL | IXON | IXOFF;
options.c_iflag &= ~(IXON | IXOFF | IXANY);
options.c_lflag &= ~(ICANON | ECHO | ECHOE | ISIG);
options.c_oflag &= ~OPOST;
options.c_cc[VMIN] = 1;
options.c_cc[VTIME] = 0;
tcsetattr(fd, TCSANOW, &options);
int status;
ioctl(fd, TIOCMGET, &status);
status &= ~TIOCM_RTS;
ioctl(fd, TIOCMSET, &status);
char data[] = "Hello, world!";
write(fd, data, sizeof(data));
char buffer[256];
int n = read(fd, buffer, sizeof(buffer));
printf("Received %d bytes: %s\n", n, buffer);
close(fd);
return 0;
}
```
注意,上述代码只是一个简单的示例,实际使用时需要根据具体的硬件和应用场景进行调整。
相关推荐
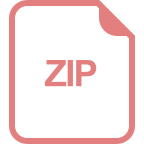
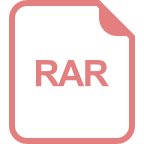














