翻译代码 computed: { column() { return bindKey => { const it = this.columns.filter(item => item.bindKey === bindKey)[0] if (it) return it else return {} } } },
时间: 2023-04-03 17:01:23 浏览: 64
这段代码是一个 Vue 组件中的 computed 计算属性,其中定义了一个 column 方法,该方法接受一个 bindKey 参数,返回一个对象。在该方法中,首先通过 filter 方法筛选出绑定键为 bindKey 的列对象,如果存在则返回该对象,否则返回一个空对象。
相关问题
computed: { enable() { const columns = this.$refs.table.columns; const enableColumns = columns.filter(column => column.id === 'enable'); return enableColumns; }, },这段代码有错误吗
这段代码的语法没有错误,但是可能会存在一些逻辑上的问题。
在这段代码中,computed属性`enable`的值是由对`$refs.table`的引用进行计算得出的。在计算过程中,会从`$refs.table.columns`数组中筛选出所有`id`属性值为`'enable'`的元素,返回一个新的数组。最后,这个新数组将作为`enable`属性的值返回。
但是,这个计算属性返回的是一个数组,而不是一个布尔值。如果您希望返回一个布尔值,您可以将`enableColumns`数组的长度作为计算属性的值返回,例如:
```
computed: {
enable() {
const columns = this.$refs.table.columns;
const enableColumns = columns.filter(column => column.id === 'enable');
return enableColumns.length > 0;
},
},
```
这样计算属性`enable`的值就会是一个布尔值,表示是否存在`id`属性值为`'enable'`的列。
优化此代码 const openKeys = ref<string[]>([]) const selectedKeys = ref<string[]>([]) const { currentMenu, currentMenuTree, currentMenuList } = storeToRefs( useLayoutStore(), ) const rootSubmenuKeys = currentMenuList.value.filter((v: any) => { if (v.type === 0) { return v.parentId } }) watch( () => currentMenu, () => { openKeys.value = [currentMenu.value?.parentId] selectedKeys.value = [currentMenu.value?.id] }, { immediate: true }, ) const router = useRouter() /** * 点击事件 * @param e 事件对象 */ const handleClick = (e: any) => { const item = currentMenuList.value.find((_) => _.id === e.key) if (item) { router.push(item.path) } } /** * SubMenu 展开/关闭的回调 * @param e 展开的openKeys */ const onOpenChange = (e: any) => { const latestOpenKey = e.find((key: any) => openKeys.value.indexOf(key) === -1) if (rootSubmenuKeys.indexOf(latestOpenKey) === -1) { openKeys.value = e } else { openKeys.value = latestOpenKey ? [latestOpenKey] : [] } }
There are a few optimizations that could be made to this code:
1. Instead of using `ref` for `openKeys` and `selectedKeys`, you can use `reactive` to make the code more concise:
```
const state = reactive({
openKeys: [],
selectedKeys: [],
})
```
2. Instead of using `storeToRefs` to convert the store state to refs, you can use the `toRefs` function, which is shorter and more concise:
```
const { currentMenu, currentMenuTree, currentMenuList } = toRefs(useLayoutStore())
```
3. Instead of using `watch` to watch the `currentMenu` state changes, you can use a computed property to update the `openKeys` and `selectedKeys` arrays:
```
const selectedMenu = computed(() => {
const item = currentMenuList.value.find((_) => _.id === currentMenu.value?.id)
return [item?.id] || []
})
const parentMenu = computed(() => {
const item = currentMenuList.value.find((_) => _.id === currentMenu.value?.parentId)
return [item?.id] || []
})
watch([selectedMenu, parentMenu], ([selected, parent]) => {
state.selectedKeys = selected
state.openKeys = parent
})
```
4. Instead of using `router.push` in the `handleClick` function, you can use the `router.push` method directly in the template:
```
<Menu.Item :key="item.id" :to="item.path">{{ item.name }}</Menu.Item>
```
5. Finally, instead of using `rootSubmenuKeys` to filter the list of menu items, you can use a computed property to filter the list of menu items based on their type:
```
const subMenuItems = computed(() => {
return currentMenuList.value.filter((v: any) => v.type === 0 && v.parentId)
})
```
By applying these optimizations, the code can be simplified and made more concise.
相关推荐
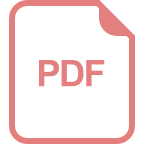
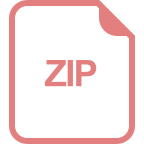
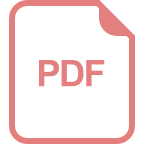












