python同时爬取多个页面数据
时间: 2023-10-06 07:06:36 浏览: 46
可以使用多线程或者异步IO的方式来同时爬取多个页面数据。
使用多线程的方式,可以使用Python标准库中的`threading`模块。具体步骤如下:
1. 定义一个函数,用于爬取单个页面的数据。
2. 创建多个线程,每个线程从一个URL列表中获取一个URL,然后调用爬取函数进行爬取。
3. 使用`join()`方法等待所有线程执行完毕。
示例代码:
```python
import threading
import requests
def get_data(url):
# 爬取数据的函数
response = requests.get(url)
data = response.json()
return data
urls = ['https://example.com/page1', 'https://example.com/page2', 'https://example.com/page3']
results = []
def worker():
# 线程函数
while True:
try:
url = urls.pop(0)
except IndexError:
# 如果URL列表为空,则退出线程
break
else:
result = get_data(url)
results.append(result)
threads = []
for i in range(3):
t = threading.Thread(target=worker)
threads.append(t)
t.start()
for t in threads:
t.join()
print(results)
```
使用异步IO的方式,可以使用Python标准库中的`asyncio`模块。具体步骤如下:
1. 定义一个异步函数,用于爬取单个页面的数据。
2. 使用`asyncio.gather()`函数同时执行多个异步函数,并等待所有函数执行完毕。
示例代码:
```python
import asyncio
import aiohttp
async def get_data(url):
# 爬取数据的异步函数
async with aiohttp.ClientSession() as session:
async with session.get(url) as response:
data = await response.json()
return data
urls = ['https://example.com/page1', 'https://example.com/page2', 'https://example.com/page3']
loop = asyncio.get_event_loop()
tasks = [get_data(url) for url in urls]
results = loop.run_until_complete(asyncio.gather(*tasks))
print(results)
```
相关推荐
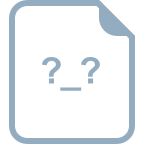
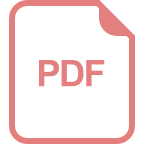














