用c++类和文件写个诗词背诵训练系统
时间: 2023-11-22 19:52:10 浏览: 79
以下是一个简单的诗词背诵训练系统的实现,使用了C++类和文件读写操作:
1. 定义一个Poem类,包含以下成员变量和方法:
```c++
class Poem {
private:
string title; // 诗词标题
string author; // 诗词作者
vector<string> lines; // 诗词每行内容
public:
Poem(); // 默认构造函数
Poem(string _title, string _author, vector<string> _lines); // 带参数构造函数
void setTitle(string _title); // 设置标题
void setAuthor(string _author); // 设置作者
void setLines(vector<string> _lines); // 设置每行内容
string getTitle(); // 获取标题
string getAuthor(); // 获取作者
vector<string> getLines(); // 获取每行内容
void display(); // 显示诗词
bool operator==(const Poem& other) const; // 重载 == 运算符
friend ostream& operator<<(ostream& os, const Poem& poem); // 重载 << 运算符,用于输出诗词
};
```
2. 实现以上成员变量和方法的函数定义,其中setLines方法需要从文件中读取每行内容:
```c++
#include <iostream>
#include <fstream>
#include <vector>
using namespace std;
class Poem {
private:
string title;
string author;
vector<string> lines;
public:
Poem() {}
Poem(string _title, string _author, vector<string> _lines) : title(_title), author(_author), lines(_lines) {}
void setTitle(string _title) {
title = _title;
}
void setAuthor(string _author) {
author = _author;
}
void setLines(vector<string> _lines) {
lines = _lines;
}
string getTitle() {
return title;
}
string getAuthor() {
return author;
}
vector<string> getLines() {
return lines;
}
void display() {
cout << "《" << title << "》" << " 作者:" << author << endl;
for (string line : lines) {
cout << line << endl;
}
}
bool operator==(const Poem& other) const {
return (title == other.title && author == other.author && lines == other.lines);
}
friend ostream& operator<<(ostream& os, const Poem& poem) {
os << "《" << poem.title << "》" << " 作者:" << poem.author << endl;
for (string line : poem.lines) {
os << line << endl;
}
return os;
}
};
vector<Poem> read_poems(string filename) {
vector<Poem> poems;
ifstream infile(filename);
if (!infile) {
cerr << "Unable to open file " << filename << endl;
exit(1);
}
string line;
while (getline(infile, line)) {
string title = line;
getline(infile, line);
string author = line;
vector<string> lines;
while (getline(infile, line)) {
if (line.empty()) {
break;
}
lines.push_back(line);
}
Poem poem(title, author, lines);
poems.push_back(poem);
}
infile.close();
return poems;
}
void write_poems(string filename, const vector<Poem>& poems) {
ofstream outfile(filename);
if (!outfile) {
cerr << "Unable to open file " << filename << endl;
exit(1);
}
for (Poem poem : poems) {
outfile << poem.getTitle() << endl;
outfile << poem.getAuthor() << endl;
for (string line : poem.getLines()) {
outfile << line << endl;
}
outfile << endl;
}
outfile.close();
}
```
3. 定义一个Training类,用于训练背诵诗词的功能,包含以下成员变量和方法:
```c++
class Training {
private:
vector<Poem> poems; // 存储所有诗词
public:
Training(string filename); // 带参数构造函数,从文件中读取诗词
void addPoem(Poem poem); // 添加新诗词
void removePoem(Poem poem); // 移除已有诗词
void displayAll(); // 显示所有诗词
void displayRandom(); // 随机显示一首诗词
void start(); // 开始训练
};
```
4. 实现以上成员变量和方法的函数定义,其中start方法是核心功能,会随机选择一首诗词,并让用户进行背诵和测试:
```c++
#include <iostream>
#include <algorithm>
#include <ctime>
#include <cstdlib>
#include <limits>
using namespace std;
class Training {
private:
vector<Poem> poems;
public:
Training(string filename) {
poems = read_poems(filename);
}
void addPoem(Poem poem) {
poems.push_back(poem);
}
void removePoem(Poem poem) {
poems.erase(remove(poems.begin(), poems.end(), poem), poems.end());
}
void displayAll() {
for (Poem poem : poems) {
poem.display();
cout << endl;
}
}
void displayRandom() {
srand(time(NULL));
int randomIndex = rand() % poems.size();
poems[randomIndex].display();
}
void start() {
srand(time(NULL));
int randomIndex = rand() % poems.size();
Poem poem = poems[randomIndex];
cout << "请背诵以下诗词:" << endl;
poem.display();
cout << "按回车键开始测试" << endl;
cin.ignore(numeric_limits<streamsize>::max(), '\n');
cout << "请在下方输入背诵结果:" << endl;
string input;
getline(cin, input);
if (input == "") {
cout << "未输入背诵结果,测试结束" << endl;
return;
}
vector<string> inputLines;
size_t start = 0, end = 0;
while ((end = input.find('\n', start)) != string::npos) {
inputLines.push_back(input.substr(start, end - start));
start = end + 1;
}
inputLines.push_back(input.substr(start));
int correctCount = 0;
for (int i = 0; i < poem.getLines().size(); i++) {
if (i >= inputLines.size()) {
break;
}
if (poem.getLines()[i] == inputLines[i]) {
correctCount++;
}
}
double accuracy = (double)correctCount / poem.getLines().size();
cout << "测试结果:" << endl;
cout << "正确率:" << accuracy * 100 << "%" << endl;
if (accuracy == 1) {
cout << "恭喜你完美背诵本诗词!" << endl;
} else {
cout << "请继续努力背诵本诗词!" << endl;
}
}
};
```
5. 在main函数中使用Training类进行功能测试:
```c++
int main() {
Training training("poems.txt");
while (true) {
cout << "请输入数字选择功能:" << endl;
cout << "1. 显示所有诗词" << endl;
cout << "2. 随机显示一首诗词" << endl;
cout << "3. 开始训练" << endl;
cout << "4. 退出程序" << endl;
int choice;
cin >> choice;
if (cin.fail()) {
cin.clear();
cin.ignore(numeric_limits<streamsize>::max(), '\n');
cout << "输入无效,请重新输入" << endl;
continue;
}
switch (choice) {
case 1:
training.displayAll();
break;
case 2:
training.displayRandom();
break;
case 3:
training.start();
break;
case 4:
cout << "程序已退出" << endl;
return 0;
default:
cout << "输入无效,请重新输入" << endl;
}
}
}
```
6. 将所有代码保存为一个文件,例如"poem_training_system.cpp",编译运行即可。
这个简单的诗词背诵训练系统可以通过读取文件中的诗词,添加和移除诗词,随机显示诗词和进行训练等功能,帮助用户提高诗词背诵能力。
相关推荐
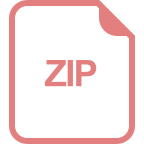
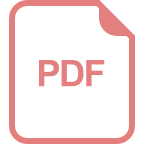














