qt调用com组件获取outlook指定用户的邮件内容
时间: 2023-10-06 22:10:09 浏览: 84
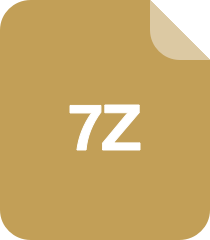
QT生成COM组件并调用COM组件测试工程.7z
您可以使用Qt的ActiveQt模块来调用COM组件,并使用Outlook Object Model来获取指定用户的邮件内容。以下是一个简单的示例代码,演示如何使用Qt和Outlook Object Model来获取指定用户的收件箱邮件内容:
```cpp
#include <QCoreApplication>
#include <QAxObject>
#include <QDebug>
int main(int argc, char *argv[])
{
QCoreApplication a(argc, argv);
// 创建Outlook.Application对象
QAxObject outlook("Outlook.Application");
// 获取指定用户的收件箱对象
QAxObject *namespaceObj = outlook.querySubObject("GetNamespace(const QString&)", "MAPI");
QAxObject *recipientObj = namespaceObj->querySubObject("CreateRecipient(const QString&)", "user@example.com");
QAxObject *recipientResolvedObj = recipientObj->querySubObject("Resolve()");
QAxObject *inboxObj = namespaceObj->querySubObject("GetDefaultFolder(int)", 6); // 6代表收件箱
QAxObject *userInboxObj = inboxObj->querySubObject("GetSharedDefaultFolder(QVariant&)", recipientResolvedObj->asVariant());
// 获取收件箱中的邮件数量
int count = userInboxObj->dynamicCall("Items").property("Count").toInt();
// 遍历收件箱中的邮件
for(int i = 1; i <= count; i++)
{
QAxObject *mailObj = userInboxObj->querySubObject("Items").querySubObject("Item(int)", i);
// 获取邮件主题和内容
QString subject = mailObj->dynamicCall("Subject").toString();
QString body = mailObj->dynamicCall("Body").toString();
qDebug() << "Subject:" << subject;
qDebug() << "Body:" << body;
mailObj->dynamicCall("Release()");
}
userInboxObj->dynamicCall("Release()");
inboxObj->dynamicCall("Release()");
recipientResolvedObj->dynamicCall("Release()");
recipientObj->dynamicCall("Release()");
namespaceObj->dynamicCall("Release()");
outlook.dynamicCall("Release()");
return a.exec();
}
```
请将代码中的"MAPI"和"user@example.com"替换为您实际使用的值。此外,代码中也可以根据需要修改获取的邮件属性,例如获取发件人、收件人、发送时间等等。
阅读全文
相关推荐
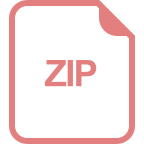
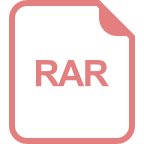










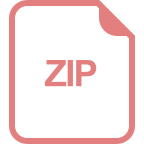
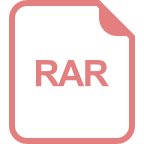
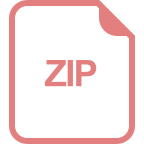
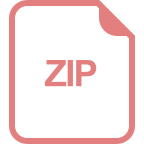
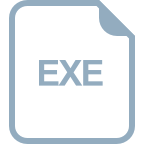