cell3.innerHTML = <select id="menu1" onchange="replaceMenu(this)"> <option value="1">选项1</option> <option value="2">选项2</option> <option value="3">选项3</option> </select>; 正确吗?
时间: 2023-06-16 11:07:01 浏览: 53
不正确。在 JavaScript 中,字符串需要用引号包裹起来。因此,可以这样写:
```
cell3.innerHTML = '<select id="menu1" onchange="replaceMenu(this)"><option value="1">选项1</option><option value="2">选项2</option><option value="3">选项3</option></select>';
```
这样就可以将字符串作为 HTML 代码插入到元素中了。注意,这段代码中的双引号需要转义。
相关问题
<!DOCTYPE html> <html> <head> <title>表格形式</title> <meta charset="UTF-8"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <style> table, th, td { border: 1px solid black; border-collapse: collapse; padding: 5px; } </style> <script> // 添加一行 function addRow(tableID) { var table = document.getElementById(tableID); var rowCount = table.rows.length; var row = table.insertRow(rowCount); for(var i = 0; i < 5; i++){ var cell = row.insertCell(i); cell.innerHTML = '<select><option value="1">选项1</option><option value="2">选项2</option><option value="3">选项3</option></select>'; cell.onclick = function(){ this.firstChild.style.display = 'block'; } cell.firstChild.onblur = function(){ this.style.display = 'none'; } cell.firstChild.style.display = 'none'; } } </script> </head> <body> <table id="myTable"> <thead> <tr> <th>列1</th> <th>列2</th> <th>列3</th> <th>列4</th> <th>列5</th> </tr> </thead> <tbody> <tr> <td><select><option value="1">选项1</option><option value="2">选项2</option><option value="3">选项3</option></select></td> <td><select><option value="1">选项1</option><option value="2">选项2</option><option value="3">选项3</option></select></td> <td><select><option value="1">选项1</option><option value="2">选项2</option><option value="3">选项3</option></select></td> <td><select><option value="1">选项1</option><option value="2">选项2</option><option value="3">选项3</option></select></td> <td><select><option value="1">选项1</option><option value="2">选项2</option><option value="3">选项3</option></select></td> </tr> </tbody> </table> <button onclick="addRow('myTable')">增加</button> </body> </html>将这段代码用bootstrap进行优化
Sure! 这个表格的代码可以用 Bootstrap 进行优化,具体的代码如下:
<!DOCTYPE html>
<html>
<head>
<title>表格形式</title>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<!-- 引入 Bootstrap 的 CSS 文件 -->
<link rel="stylesheet" href="https://cdn.staticfile.org/twitter-bootstrap/4.5.0/css/bootstrap.min.css">
<style>
table { border: 1px solid black; border-collapse: collapse; padding: 5px; }
</style>
</head>
<body>
<div class="container">
<table class="table table-bordered table-hover">
<thead>
<tr>
<th>列1</th>
<th>列2</th>
<th>列3</th>
<th>列4</th>
<th>列5</th>
</tr>
</thead>
<tbody>
<tr>
<td>
<select class="form-control">
<option value="1">选项1</option>
<option value="2">选项2</option>
<option value="3">选项3</option>
</select>
</td>
<td>
<select class="form-control">
<option value="1">选项1</option>
<option value="2">选项2</option>
<option value="3">选项3</option>
</select>
</td>
<td>
<select class="form-control">
<option value="1">选项1</option>
<option value="2">选项2</option>
<option value="3">选项3</option>
</select>
</td>
<td>
<select class="form-control">
<option value="1">选项1</option>
<option value="2">选项2</option>
<option value="3">选项3</option>
</select>
</td>
<td>
<select class="form-control">
<option value="1">选项1</option>
<option value="2">选项2</option>
<option value="3">选项3</option>
</select>
</td>
</tr>
</tbody>
</table>
<div class="text-center">
<button class="btn btn-primary" onClick="addRow('myTable')">增加</button>
</div>
</div>
<!-- 引入 Bootstrap 的 JS 文件 -->
<script src="https://cdn.staticfile.org/jquery/3.5.1/jquery.min.js"></script>
<script src="https://cdn.staticfile.org/popper.js/1.16.0/umd/popper.min.js"></script>
<script src="https://cdn.staticfile.org/twitter-bootstrap/4.5.0/js/bootstrap.min.js"></script>
<script>
// 添加一行
function addRow(tableID) {
var table = document.getElementById(tableID);
var rowCount = table.rows.length;
var row = table.insertRow(rowCount);
for(var i = 0; i < 5; i++) {
var cell = row.insertCell(i);
cell.innerHTML = '<select class="form-control"><option value="1">选项1</option><option value="2">选项2</option><option value="3">选项3</option></select>';
cell.onclick = function(){ this.firstChild.style.display = 'block'; }
cell.firstChild.onblur = function(){ this.style.display = 'none'; }
cell.firstChild.style.display = 'none'; }
}
</script>
</body>
</html>
我希望这个帮助到你!
deleteCell.innerHTML = '<a href="javascript:; value"1" >删除</a>'
这段代码存在语法错误。应该将value属性的值用双引号或单引号括起来,以避免语法错误。修改后的代码如下所示:
```
deleteCell.innerHTML = '<a href="javascript:;" value="1">删除</a>';
```
这样,这个a标签的value属性就被设置为了1。
相关推荐
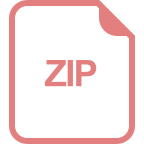
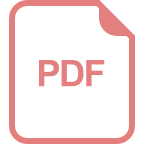
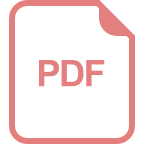












