Opengl三维地形动态显示程序设计代码
时间: 2023-11-12 16:24:21 浏览: 80
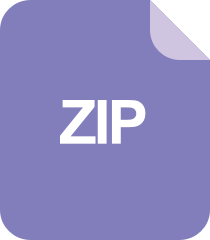
三维地形显示代码
下面是一个OpenGL三维地形动态显示程序的简单代码示例:
```c++
#include <GL/glut.h>
#include <cmath>
const int WIDTH = 800;
const int HEIGHT = 600;
// 地形高度图的宽度和高度
const int MAP_WIDTH = 256;
const int MAP_HEIGHT = 256;
// 地形高度图数据
unsigned char heightMap[MAP_WIDTH][MAP_HEIGHT];
// 地形顶点数组
float vertices[MAP_WIDTH][MAP_HEIGHT][3];
// 地形法向量数组
float normals[MAP_WIDTH][MAP_HEIGHT][3];
// 摄像机位置
float cameraX = 0.0f;
float cameraY = 0.0f;
float cameraZ = 0.0f;
// 摄像机方向
float pitch = 0.0f;
float yaw = 0.0f;
void generateHeightMap()
{
// 生成随机高度图
for (int i = 0; i < MAP_WIDTH; i++)
{
for (int j = 0; j < MAP_HEIGHT; j++)
{
heightMap[i][j] = rand() % 256;
}
}
}
void generateVertices()
{
// 根据高度图生成顶点数组
for (int i = 0; i < MAP_WIDTH; i++)
{
for (int j = 0; j < MAP_HEIGHT; j++)
{
vertices[i][j][0] = i - MAP_WIDTH / 2;
vertices[i][j][1] = heightMap[i][j] / 10.0f;
vertices[i][j][2] = j - MAP_HEIGHT / 2;
}
}
}
void generateNormals()
{
// 根据顶点数组生成法向量数组
for (int i = 0; i < MAP_WIDTH - 1; i++)
{
for (int j = 0; j < MAP_HEIGHT - 1; j++)
{
float x1 = vertices[i][j][0];
float y1 = vertices[i][j][1];
float z1 = vertices[i][j][2];
float x2 = vertices[i+1][j][0];
float y2 = vertices[i+1][j][1];
float z2 = vertices[i+1][j][2];
float x3 = vertices[i][j+1][0];
float y3 = vertices[i][j+1][1];
float z3 = vertices[i][j+1][2];
float ax = x1 - x2;
float ay = y1 - y2;
float az = z1 - z2;
float bx = x3 - x2;
float by = y3 - y2;
float bz = z3 - z2;
float nx = ay * bz - az * by;
float ny = az * bx - ax * bz;
float nz = ax * by - ay * bx;
float length = sqrt(nx * nx + ny * ny + nz * nz);
normals[i][j][0] = nx / length;
normals[i][j][1] = ny / length;
normals[i][j][2] = nz / length;
}
}
}
void init()
{
// 初始化OpenGL窗口
glClearColor(0.0f, 0.0f, 0.0f, 0.0f);
glMatrixMode(GL_PROJECTION);
glLoadIdentity();
gluPerspective(45.0, (double)WIDTH / (double)HEIGHT, 0.1, 1000.0);
glEnable(GL_DEPTH_TEST);
// 生成随机高度图和顶点数组
generateHeightMap();
generateVertices();
generateNormals();
}
void display()
{
// 清除屏幕和深度缓冲区
glClear(GL_COLOR_BUFFER_BIT | GL_DEPTH_BUFFER_BIT);
// 设置摄像机位置和方向
glMatrixMode(GL_MODELVIEW);
glLoadIdentity();
gluLookAt(cameraX, cameraY, cameraZ,
cameraX + sin(yaw), cameraY - sin(pitch), cameraZ - cos(yaw),
0.0f, 1.0f, 0.0f);
// 绘制三角形网格
glBegin(GL_TRIANGLES);
for (int i = 0; i < MAP_WIDTH - 1; i++)
{
for (int j = 0; j < MAP_HEIGHT - 1; j++)
{
float x1 = vertices[i][j][0];
float y1 = vertices[i][j][1];
float z1 = vertices[i][j][2];
float x2 = vertices[i+1][j][0];
float y2 = vertices[i+1][j][1];
float z2 = vertices[i+1][j][2];
float x3 = vertices[i][j+1][0];
float y3 = vertices[i][j+1][1];
float z3 = vertices[i][j+1][2];
float nx1 = normals[i][j][0];
float ny1 = normals[i][j][1];
float nz1 = normals[i][j][2];
float nx2 = normals[i+1][j][0];
float ny2 = normals[i+1][j][1];
float nz2 = normals[i+1][j][2];
float nx3 = normals[i][j+1][0];
float ny3 = normals[i][j+1][1];
float nz3 = normals[i][j+1][2];
glNormal3f(nx1, ny1, nz1);
glVertex3f(x1, y1, z1);
glNormal3f(nx2, ny2, nz2);
glVertex3f(x2, y2, z2);
glNormal3f(nx3, ny3, nz3);
glVertex3f(x3, y3, z3);
x1 = vertices[i+1][j][0];
y1 = vertices[i+1][j][1];
z1 = vertices[i+1][j][2];
x2 = vertices[i+1][j+1][0];
y2 = vertices[i+1][j+1][1];
z2 = vertices[i+1][j+1][2];
x3 = vertices[i][j+1][0];
y3 = vertices[i][j+1][1];
z3 = vertices[i][j+1][2];
nx1 = normals[i+1][j][0];
ny1 = normals[i+1][j][1];
nz1 = normals[i+1][j][2];
nx2 = normals[i+1][j+1][0];
ny2 = normals[i+1][j+1][1];
nz2 = normals[i+1][j+1][2];
nx3 = normals[i][j+1][0];
ny3 = normals[i][j+1][1];
nz3 = normals[i][j+1][2];
glNormal3f(nx1, ny1, nz1);
glVertex3f(x1, y1, z1);
glNormal3f(nx2, ny2, nz2);
glVertex3f(x2, y2, z2);
glNormal3f(nx3, ny3, nz3);
glVertex3f(x3, y3, z3);
}
}
glEnd();
// 刷新屏幕
glutSwapBuffers();
}
void keyboard(unsigned char key, int x, int y)
{
// 处理键盘输入
switch (key)
{
case 'w':
cameraX += sin(yaw);
cameraZ -= cos(yaw);
break;
case 's':
cameraX -= sin(yaw);
cameraZ += cos(yaw);
break;
case 'a':
cameraX += sin(yaw - M_PI / 2);
cameraZ -= cos(yaw - M_PI / 2);
break;
case 'd':
cameraX += sin(yaw + M_PI / 2);
cameraZ -= cos(yaw + M_PI / 2);
break;
case 'q':
cameraY += 0.1f;
break;
case 'e':
cameraY -= 0.1f;
break;
}
}
void mouse(int x, int y)
{
// 处理鼠标输入
static int lastX = 0;
static int lastY = 0;
if (lastX != 0 || lastY != 0)
{
yaw += (x - lastX) * 0.01f;
pitch += (y - lastY) * 0.01f;
if (pitch > M_PI / 2)
{
pitch = M_PI / 2;
}
else if (pitch < -M_PI / 2)
{
pitch = -M_PI / 2;
}
}
lastX = x;
lastY = y;
}
int main(int argc, char** argv)
{
// 初始化OpenGL窗口和GLEW库
glutInit(&argc, argv);
glutInitDisplayMode(GLUT_DOUBLE | GLUT_RGB | GLUT_DEPTH);
glutInitWindowSize(WIDTH, HEIGHT);
glutCreateWindow("Dynamic Terrain");
glewInit();
// 初始化OpenGL状态和数据
init();
// 注册回调函数
glutDisplayFunc(display);
glutKeyboardFunc(keyboard);
glutPassiveMotionFunc(mouse);
// 进入主循环
glutMainLoop();
return 0;
}
```
这个程序使用OpenGL绘制了一个随机生成的三维地形,并且可以使用键盘和鼠标控制摄像机位置和方向。它使用了一个高度图来生成地形,并且计算了每个顶点的法向量,以便在光照计算中使用。
阅读全文
相关推荐
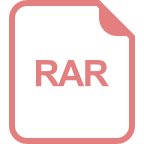

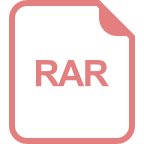
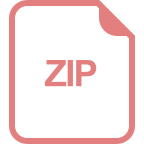
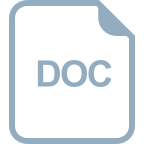

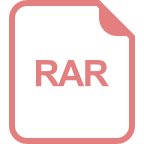
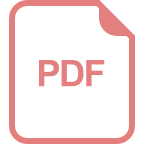
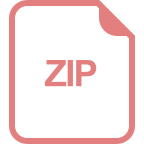
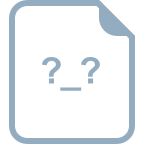
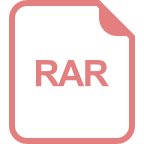
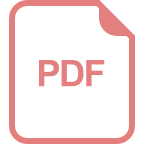
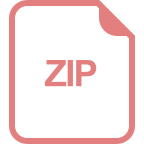
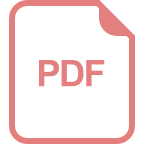
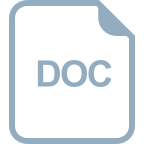