想做一个Unity固定位置十张图片,每隔三秒钟出现一张,每次出现的图片不按照顺序来
时间: 2024-03-20 09:41:25 浏览: 15
你可以通过编写一个脚本来实现这个功能。首先,你需要创建一个空物体,并将其命名为“ImageManager”。然后,将十张图片作为子对象添加到这个空物体中,并将它们命名为“Image1”到“Image10”。
接下来,你需要编写一个C#脚本,将其命名为“ImageSwitcher”。将这个脚本附加到ImageManager物体上。以下是示例代码:
```csharp
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class ImageSwitcher : MonoBehaviour
{
public GameObject[] images;
public float switchInterval = 3.0f;
private int currentIndex = -1;
// Start is called before the first frame update
void Start()
{
StartCoroutine(SwitchImages());
}
IEnumerator SwitchImages()
{
while(true)
{
// 随机选择一个图片
int newIndex = Random.Range(0, images.Length);
while(newIndex == currentIndex)
{
newIndex = Random.Range(0, images.Length);
}
// 隐藏当前图片
if(currentIndex >= 0)
{
images[currentIndex].SetActive(false);
}
// 显示新图片
images[newIndex].SetActive(true);
currentIndex = newIndex;
yield return new WaitForSeconds(switchInterval);
}
}
}
```
这个脚本使用了协程来实现每隔一定时间切换图片的功能。它首先定义了一个包含所有图片的数组和切换时间间隔的变量。然后,在Start()方法中启动协程SwitchImages()。
在SwitchImages()方法中,使用一个无限循环来不断切换图片。每次循环中,使用Random.Range()方法随机选择一个不同于上一次选择的图片,并将其显示出来。为了隐藏上一次显示的图片,需要将其SetActive()方法设置为false。最后,使用yield return new WaitForSeconds()方法等待指定的时间间隔后再进行下一次循环。
将这个脚本附加到ImageManager物体上,并将图片拖放到images数组中即可实现你想要的功能。
相关推荐
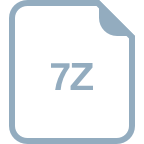














