首页print(xi,n,yi,end='')中end的值如何设置
print(xi,n,yi,end='')中end的值如何设置
时间: 2024-05-01 07:20:47 浏览: 86
在Python中,print函数中的end参数用于指定输出内容的结尾字符,默认值为换行符"\n"。如果想要修改结尾字符,可以将end参数设置为需要的字符或字符串。
例如,如果希望在每个输出值之间使用逗号分隔,可以将end参数设置为逗号:
```
print(xi, n, yi, end=',')
```
如果希望输出值之间没有任何分隔符,可以将end参数设置为空字符串:
```
print(xi, n, yi, end='')
```
如果想要输出值之间使用其他字符或字符串分隔,只需要将end参数设置为需要的分隔符即可。
阅读全文
CSDN会员
开通CSDN年卡参与万元壕礼抽奖
最新推荐
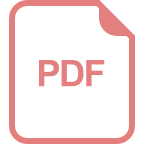
浅谈python print(xx, flush = True) 全网最清晰的解释
在Python编程语言中,`print()`函数是我们日常开发中经常使用的输出工具,它用于将文本、变量等内容打印到控制台或者文件中。然而,对于一些特定情况,`print()`默认的行为可能并不符合我们的期待,特别是在实时输出...
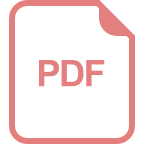
tensorflow实现在函数中用tf.Print输出中间值
例如,在一个循环中,每次迭代都使用`tf.Print`更新的张量,这样每次张量的值改变时,都会触发`tf.Print`的输出。 在示例代码中,`test()`函数展示了不同用法的影响。首先,如果`a_print`只在循环外定义一次,那么`...
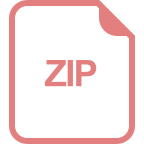
Raspberry Pi OpenCL驱动程序安装与QEMU仿真指南
资源摘要信息:"RaspberryPi-OpenCL驱动程序"
知识点一:Raspberry Pi与OpenCL
Raspberry Pi是一系列低成本、高能力的单板计算机,由Raspberry Pi基金会开发。这些单板计算机通常用于教育、电子原型设计和家用服务器。而OpenCL(Open Computing Language)是一种用于编写程序,这些程序可以在不同种类的处理器(包括CPU、GPU和其他处理器)上执行的标准。OpenCL驱动程序是为Raspberry Pi上的应用程序提供支持,使其能够充分利用板载硬件加速功能,进行并行计算。
知识点二:调整Raspberry Pi映像大小
在准备Raspberry Pi的操作系统映像以便在QEMU仿真器中使用时,我们经常需要调整映像的大小以适应仿真环境或为了确保未来可以进行系统升级而留出足够的空间。这涉及到使用工具来扩展映像文件,以增加可用的磁盘空间。在描述中提到的命令包括使用`qemu-img`工具来扩展映像文件`2021-01-11-raspios-buster-armhf-lite.img`的大小。
知识点三:使用QEMU进行仿真
QEMU是一个通用的开源机器模拟器和虚拟化器,它能够在一台计算机上模拟另一台计算机。它可以运行在不同的操作系统上,并且能够模拟多种不同的硬件设备。在Raspberry Pi的上下文中,QEMU能够被用来模拟Raspberry Pi硬件,允许开发者在没有实际硬件的情况下测试软件。描述中给出了安装QEMU的命令行指令,并建议更新系统软件包后安装QEMU。
知识点四:管理磁盘分区
描述中提到了使用`fdisk`命令来检查磁盘分区,这是Linux系统中用于查看和修改磁盘分区表的工具。在进行映像调整大小的过程中,了解当前的磁盘分区状态是十分重要的,以确保不会对现有的数据造成损害。在确定需要增加映像大小后,通过指定的参数可以将映像文件的大小增加6GB。
知识点五:Raspbian Pi OS映像
Raspbian是Raspberry Pi的官方推荐操作系统,是一个为Raspberry Pi量身打造的基于Debian的Linux发行版。Raspbian Pi OS映像文件是指定的、压缩过的文件,包含了操作系统的所有数据。通过下载最新的Raspbian Pi OS映像文件,可以确保你拥有最新的软件包和功能。下载地址被提供在描述中,以便用户可以获取最新映像。
知识点六:内核提取
描述中提到了从仓库中获取Raspberry-Pi Linux内核并将其提取到一个文件夹中。这意味着为了在QEMU中模拟Raspberry Pi环境,可能需要替换或更新操作系统映像中的内核部分。内核是操作系统的核心部分,负责管理硬件资源和系统进程。提取内核通常涉及到解压缩下载的映像文件,并可能需要重命名相关文件夹以确保与Raspberry Pi的兼容性。
总结:
描述中提供的信息详细说明了如何通过调整Raspberry Pi操作系统映像的大小,安装QEMU仿真器,获取Raspbian Pi OS映像,以及处理磁盘分区和内核提取来准备Raspberry Pi的仿真环境。这些步骤对于IT专业人士来说,是在虚拟环境中测试Raspberry Pi应用程序或驱动程序的关键步骤,特别是在开发OpenCL应用程序时,对硬件资源的配置和管理要求较高。通过理解上述知识点,开发者可以更好地利用Raspberry Pi的并行计算能力,进行高性能计算任务的仿真和测试。

管理建模和仿真的文件
管理Boualem Benatallah引用此版本:布阿利姆·贝纳塔拉。管理建模和仿真。约瑟夫-傅立叶大学-格勒诺布尔第一大学,1996年。法语。NNT:电话:00345357HAL ID:电话:00345357https://theses.hal.science/tel-003453572008年12月9日提交HAL是一个多学科的开放存取档案馆,用于存放和传播科学研究论文,无论它们是否被公开。论文可以来自法国或国外的教学和研究机构,也可以来自公共或私人研究中心。L’archive ouverte pluridisciplinaire
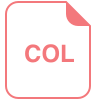
Fluent UDF实战攻略:案例分析与高效代码编写
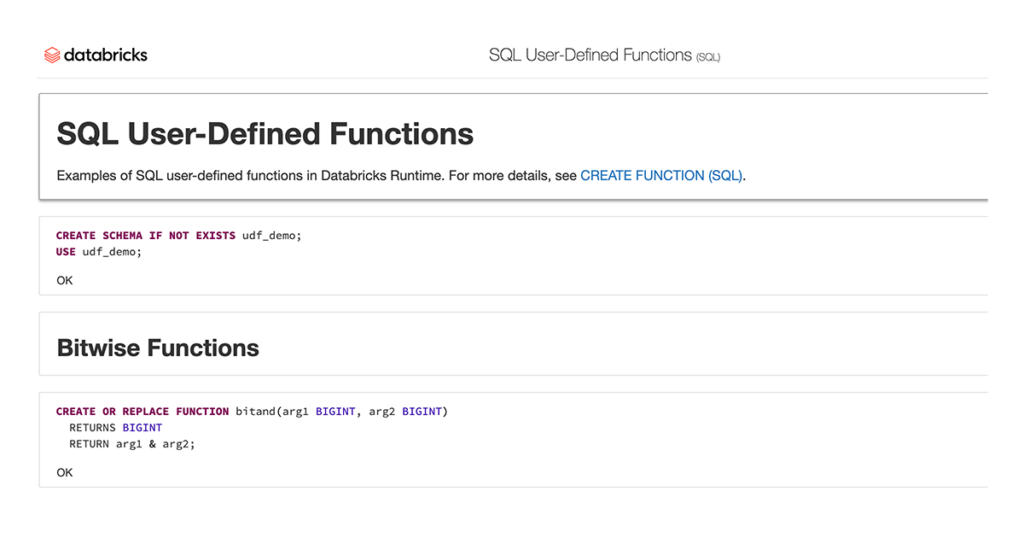
参考资源链接:[fluent UDF中文帮助文档](https://wenku.csdn.net/doc/6401abdccce7214c316e9c28?spm=1055.2635.3001.10343)
# 1. Fluent UDF基础与应用概览
流体动力学仿真软件Fluent在工程领域被广泛应用于流体流动和热传递问题的模拟。Fluent UDF(User-Defin

如何使用DPDK技术在云数据中心中实现高效率的流量监控与网络安全分析?
在云数据中心领域,随着服务的多样化和用户需求的增长,传统的网络监控和分析方法已经无法满足日益复杂的网络环境。DPDK技术的引入,为解决这一挑战提供了可能。DPDK是一种高性能的数据平面开发套件,旨在优化数据包处理速度,降低延迟,并提高网络吞吐量。具体到实现高效率的流量监控与网络安全分析,可以遵循以下几个关键步骤:
参考资源链接:[DPDK峰会:云数据中心安全实践 - 流量监控与分析](https://wenku.csdn.net/doc/1bq8jittzn?spm=1055.2569.3001.10343)
首先,需要了解DPDK的基本架构和工作原理,特别是它如何通过用户空间驱动程序和大
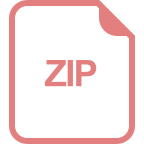
Apache RocketMQ Go客户端:全面支持与消息处理功能
资源摘要信息:"rocketmq-client-go:Apache RocketMQ Go客户端"
Apache RocketMQ Go客户端是专为Go语言开发的RocketMQ客户端库,它几乎涵盖了Apache RocketMQ的所有核心功能,允许Go语言开发者在Go项目中便捷地实现消息的发布与订阅、访问控制列表(ACL)权限管理、消息跟踪等高级特性。该客户端库的设计旨在提供一种简单、高效的方式来与RocketMQ服务进行交互。
核心知识点如下:
1. 发布与订阅消息:RocketMQ Go客户端支持多种消息发送模式,包括同步模式、异步模式和单向发送模式。同步模式允许生产者在发送消息后等待响应,确保消息成功到达。异步模式适用于对响应时间要求不严格的场景,生产者在发送消息时不会阻塞,而是通过回调函数来处理响应。单向发送模式则是最简单的发送方式,只负责将消息发送出去而不关心是否到达,适用于对消息送达不敏感的场景。
2. 发送有条理的消息:在某些业务场景中,需要保证消息的顺序性,比如订单处理。RocketMQ Go客户端提供了按顺序发送消息的能力,确保消息按照发送顺序被消费者消费。
3. 消费消息的推送模型:消费者可以设置为使用推送模型,即消息服务器主动将消息推送给消费者,这种方式可以减少消费者轮询消息的开销,提高消息处理的实时性。
4. 消息跟踪:对于生产环境中的消息传递,了解消息的完整传递路径是非常必要的。RocketMQ Go客户端提供了消息跟踪功能,可以追踪消息从发布到最终消费的完整过程,便于问题的追踪和诊断。
5. 生产者和消费者的ACL:访问控制列表(ACL)是一种权限管理方式,RocketMQ Go客户端支持对生产者和消费者的访问权限进行细粒度控制,以满足企业对数据安全的需求。
6. 如何使用:RocketMQ Go客户端提供了详细的使用文档,新手可以通过分步说明快速上手。而有经验的开发者也可以根据文档深入了解其高级特性。
7. 社区支持:Apache RocketMQ是一个开源项目,拥有活跃的社区支持。无论是使用过程中遇到问题还是想要贡献代码,都可以通过邮件列表与社区其他成员交流。
8. 快速入门:为了帮助新用户快速开始使用RocketMQ Go客户端,官方提供了快速入门指南,其中包含如何设置rocketmq代理和名称服务器等基础知识。
在安装和配置方面,用户通常需要首先访问RocketMQ的官方网站或其在GitHub上的仓库页面,下载最新版本的rocketmq-client-go包,然后在Go项目中引入并初始化客户端。配置过程中可能需要指定RocketMQ服务器的地址和端口,以及设置相应的命名空间或主题等。
对于实际开发中的使用,RocketMQ Go客户端的API设计注重简洁性和直观性,使得Go开发者能够很容易地理解和使用,而不需要深入了解RocketMQ的内部实现细节。但是,对于有特殊需求的用户,Apache RocketMQ社区文档和代码库中提供了大量的参考信息和示例代码,可以用于解决复杂的业务场景。
由于RocketMQ的版本迭代,不同版本的RocketMQ Go客户端可能会引入新的特性和对已有功能的改进。因此,用户在使用过程中应该关注官方发布的版本更新日志,以确保能够使用到最新的特性和性能优化。对于版本2.0.0的特定特性,文档中提到的以同步模式、异步模式和单向方式发送消息,以及消息排序、消息跟踪、ACL等功能,是该版本客户端的核心优势,用户可以根据自己的业务需求进行选择和使用。
总之,rocketmq-client-go作为Apache RocketMQ的Go语言客户端,以其全面的功能支持、简洁的API设计、活跃的社区支持和详尽的文档资料,成为Go开发者在构建分布式应用和消息驱动架构时的得力工具。

"互动学习:行动中的多样性与论文攻读经历"
多样性她- 事实上SCI NCES你的时间表ECOLEDO C Tora SC和NCESPOUR l’Ingén学习互动,互动学习以行动为中心的强化学习学会互动,互动学习,以行动为中心的强化学习计算机科学博士论文于2021年9月28日在Villeneuve d'Asq公开支持马修·瑟林评审团主席法布里斯·勒菲弗尔阿维尼翁大学教授论文指导奥利维尔·皮耶昆谷歌研究教授:智囊团论文联合主任菲利普·普雷教授,大学。里尔/CRISTAL/因里亚报告员奥利维耶·西格德索邦大学报告员卢多维奇·德诺耶教授,Facebook /索邦大学审查员越南圣迈IMT Atlantic高级讲师邀请弗洛里安·斯特鲁布博士,Deepmind对于那些及时看到自己错误的人...3谢谢你首先,我要感谢我的两位博士生导师Olivier和Philippe。奥利维尔,"站在巨人的肩膀上"这句话对你来说完全有意义了。从科学上讲,你知道在这篇论文的(许多)错误中,你是我可以依
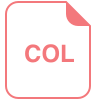
Fluent UDF进阶秘籍:解锁高级功能与优化技巧

参考资源链接:[fluent UDF中文帮助文档](https://wenku.csdn.net/doc/6401abdccce7214c316e9c28?spm=1055.2635.3001.10343)
# 1. Fluent UDF简介与安装配置
## 1.1 Fluent UDF概述
Fluent UDF(User-Defined Functions,用户自定义函数)是Ansys F

在Vue项目中,如何利用Vuex进行高效的状态管理,并简要比较React中Redux或MobX的状态管理模式?
在Vue项目中,状态管理是构建大型应用的关键部分。Vuex是Vue.js的官方状态管理库,它提供了一个中心化的存储来管理所有组件的状态,确保状态的变化可以被跟踪和调试。
参考资源链接:[前端面试必备:全栈面试题及 Vue 面试题解析](https://wenku.csdn.net/doc/5edpb49q1y?spm=1055.2569.3001.10343)
要高效地在Vue项目中实现组件间的状态管理,首先需要理解Vuex的核心概念,包括state、getters、mutations、actions和modules。以下是一些关键步骤:
1. **安装和配置Vuex**:首先,在项目中