qt中使用Windows API函数CreateProcess启动msu文件
时间: 2023-06-02 14:02:19 浏览: 311
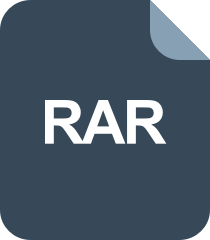
WindowsAPI函数列表.rar_Windows API函数
在Qt中使用Windows API函数CreateProcess启动msu文件的步骤如下:
1. 在Qt项目中包含Windows.h头文件。
```c++
#include <Windows.h>
```
2. 定义CreateProcess函数需要的参数,包括待启动的msu文件路径和命令行参数。
```c++
LPTSTR applicationName = L"C:\\Windows\\System32\\wusa.exe"; // msu文件启动程序路径
LPTSTR commandLine = L"C:\\temp\\update.msu /quiet /norestart"; // msu文件路径和命令行参数
LPSECURITY_ATTRIBUTES processAttributes = NULL;
LPSECURITY_ATTRIBUTES threadAttributes = NULL;
BOOL inheritHandles = FALSE;
DWORD creationFlags = 0;
LPVOID environment = NULL;
LPTSTR currentDirectory = NULL;
LPSTARTUPINFO startupInfo = new STARTUPINFO;
LPPROCESS_INFORMATION processInformation = new PROCESS_INFORMATION;
ZeroMemory(startupInfo, sizeof(STARTUPINFO));
ZeroMemory(processInformation, sizeof(PROCESS_INFORMATION));
startupInfo->cb = sizeof(STARTUPINFO);
```
3. 调用CreateProcess函数启动msu文件。
```c++
BOOL success = CreateProcess(applicationName, commandLine, processAttributes, threadAttributes, inheritHandles, creationFlags, environment, currentDirectory, startupInfo, processInformation);
if (success) {
qDebug() << "Process started";
} else {
qDebug() << "Process failed to start";
}
```
完整代码如下:
```c++
#include <QCoreApplication>
#include <QDebug>
#include <Windows.h>
int main(int argc, char *argv[])
{
QCoreApplication a(argc, argv);
// Define CreateProcess parameters
LPTSTR applicationName = L"C:\\Windows\\System32\\wusa.exe"; // msu file launch program path
LPTSTR commandLine = L"C:\\temp\\update.msu /quiet /norestart"; // msu file path and command line parameters
LPSECURITY_ATTRIBUTES processAttributes = NULL;
LPSECURITY_ATTRIBUTES threadAttributes = NULL;
BOOL inheritHandles = FALSE;
DWORD creationFlags = 0;
LPVOID environment = NULL;
LPTSTR currentDirectory = NULL;
LPSTARTUPINFO startupInfo = new STARTUPINFO;
LPPROCESS_INFORMATION processInformation = new PROCESS_INFORMATION;
ZeroMemory(startupInfo, sizeof(STARTUPINFO));
ZeroMemory(processInformation, sizeof(PROCESS_INFORMATION));
startupInfo->cb = sizeof(STARTUPINFO);
// Start process
BOOL success = CreateProcess(applicationName, commandLine, processAttributes, threadAttributes, inheritHandles, creationFlags, environment, currentDirectory, startupInfo, processInformation);
if (success) {
qDebug() << "Process started";
} else {
qDebug() << "Process failed to start";
}
// Cleanup
delete startupInfo;
CloseHandle(processInformation->hProcess);
CloseHandle(processInformation->hThread);
delete processInformation;
return a.exec();
}
```
阅读全文
相关推荐
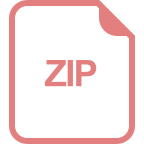
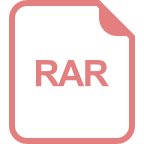















