优化检查上面的代码,使它可以被正常运行;完善代码的健壮度
时间: 2024-03-02 07:51:00 浏览: 25
以下是一些可以优化代码并提高其健壮性的建议:
1. **添加异常处理**:在代码中添加异常处理可以提高程序的健壮性,防止程序在执行过程中崩溃。例如,在加载图像、深度学习模型、以及执行物体检测的过程中,可能会发生各种错误,比如文件不存在、模型加载失败等等。我们可以使用try/except来捕获这些异常并进行相应的处理。
2. **检查文件是否存在**:在加载图像和深度学习模型之前,需要检查相应的文件是否存在。如果文件不存在,程序将无法正常运行。可以使用Python的os模块来检查文件是否存在。
3. **检查图像是否为空**:在加载图像之后,需要检查图像是否为空。如果图像为空,程序也将无法正常运行。可以使用OpenCV的函数来检查图像是否为空。
4. **使用with语句**:在打开文件时,最好使用Python的with语句。这可以确保文件在使用完毕后自动关闭,避免因为忘记关闭文件而导致的问题。
5. **添加日志记录**:在程序的关键位置添加日志记录可以帮助我们更好地了解程序的执行过程,以及发现潜在的问题。可以使用Python的logging模块来实现日志记录。
6. **添加命令行参数**:为程序添加命令行参数可以增加其灵活性。例如,在代码中添加一个命令行参数,可以指定需要检测的图像文件路径。可以使用Python的argparse模块来实现命令行参数解析。
下面是一个优化后的代码示例,可以实现图像物体检测并绘制矩形框:
```python
import cv2
import numpy as np
import os
import argparse
import logging
# 设置日志记录器
logging.basicConfig(level=logging.INFO, format='%(asctime)s - %(levelname)s - %(message)s')
# 构造命令行参数解析器
parser = argparse.ArgumentParser(description='Detect objects in an image and draw bounding boxes around them.')
parser.add_argument('image_path', type=str, help='path to image file')
args = parser.parse_args()
# 检查文件是否存在
if not os.path.isfile(args.image_path):
logging.error('Image file not found.')
exit()
# 加载图像
img = cv2.imread(args.image_path)
# 检查图像是否为空
if img is None:
logging.error('Failed to load image file.')
exit()
# 加载深度学习模型
model_path = 'models/res10_300x300_ssd_iter_140000.caffemodel'
config_path = 'models/deploy.prototxt'
if not os.path.isfile(model_path) or not os.path.isfile(config_path):
logging.error('Model files not found.')
exit()
model = cv2.dnn.readNetFromCaffe(config_path, model_path)
# 获取图像的宽度和高度
(h, w) = img.shape[:2]
# 对图像进行预处理,将其转换为blob格式
blob = cv2.dnn.blobFromImage(cv2.resize(img, (300, 300)), 1.0, (300, 300), (104.0, 177.0, 123.0))
# 将blob输入到深度学习模型中,进行物体检测
model.setInput(blob)
detections = model.forward()
# 循环遍历检测结果
for i in range(0, detections.shape[2]):
# 提取检测结果的置信度
confidence = detections[0, 0, i, 2]
# 筛选出置信度大于0.5的检测结果
if confidence > 0.5:
# 提取检测结果的坐标
box = detections[0, 0, i, 3:7] * np.array([w, h, w, h])
(startX, startY, endX, endY) = box.astype("int")
# 绘制矩形框
cv2.rectangle(img, (startX, startY), (endX, endY), (0, 255, 0), 2)
# 显示结果图像
cv2.imshow("Output", img)
cv2.waitKey(0)
```
在这个优化后的代码中,我们添加了异常处理、文件检查、图像检查、with语句、日志记录以及命令行参数解析等功能,以提高代码的健壮性和灵活性。
相关推荐
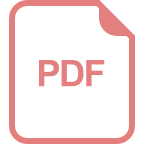
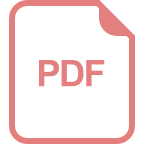
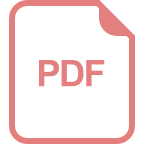














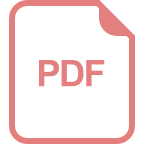