用C语言写编写函数检测输入的一个字符是大写字母还是小写字母或不是26英文字母
时间: 2023-06-15 11:06:35 浏览: 98
可以使用C语言中的标准函数isupper和islower来判断输入的字符是否为大写字母或小写字母。具体实现如下:
```c
#include <stdio.h>
#include <ctype.h>
void detectCharType(char c){
if(islower(c)){
printf("%c是小写字母\n", c);
}else if(isupper(c)){
printf("%c是大写字母\n", c);
}else{
printf("%c不是26英文字母\n", c);
}
}
int main(){
char c;
printf("请输入一个字符:");
scanf("%c", &c);
detectCharType(c);
return 0;
}
```
首先,我们通过scanf函数读取输入的字符c。然后调用detectCharType函数来判断字符类型。如果是小写字母,则调用islower函数返回非零值,输出“是小写字母”;如果是大写字母,则调用isupper函数返回非零值,输出“是大写字母”;如果不是26英文字母,则输出“不是26英文字母”。
相关问题
编写一个函数来检验输入的一个字符是大写字母还是小写字母或不是26个英文字母。C语言
的代码怎么写?
以下是 C 语言的代码实现:
```c
#include <stdio.h>
int checkAlphabet(char ch) {
if ((ch >= 'a' && ch <= 'z') || (ch >= 'A' && ch <= 'Z')) {
if (ch >= 'a' && ch <= 'z') {
printf("The input is a lowercase alphabet.\n");
} else {
printf("The input is an uppercase alphabet.\n");
}
} else {
printf("The input is not an alphabet.\n");
}
}
int main() {
char input;
printf("Please enter a character: ");
scanf("%c", &input);
checkAlphabet(input);
return 0;
}
```
此代码中,checkAlphabet() 函数接收一个字符作为参数,并通过判断字符是否在 `'a'` 到 `'z'` 或 `'A'` 到 `'Z'` 之间来判断输入是否为字母,并输出结果。在 main() 函数中,用户输入一个字符并调用 checkAlphabet() 函数来检验该字符是否为字母。
用c语言输入一个字符判断大写字母还是小写字母或者是数字
可以使用 C 语言中的 `isupper()`、`islower()` 和 `isdigit()` 函数来判断一个字符是大写字母、小写字母还是数字。以下是一个示例程序:
```
#include <stdio.h>
#include <ctype.h>
int main() {
char ch;
printf("请输入一个字符:\n");
scanf("%c", &ch);
if (isupper(ch)) {
printf("%c 是大写字母。\n", ch);
} else if (islower(ch)) {
printf("%c 是小写字母。\n", ch);
} else if (isdigit(ch)) {
printf("%c 是数字。\n", ch);
} else {
printf("%c 不是字母或数字。\n", ch);
}
return 0;
}
```
在上面的程序中,`isupper()`、`islower()` 和 `isdigit()` 分别用于判断一个字符是否是大写字母、小写字母和数字。当输入的字符不是字母或数字时,会输出相应的提示信息。
阅读全文
相关推荐
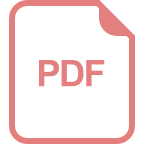













