用python实现基于概率分布的超声图像与自然图像性质差异分析,不用greycomatrix和greycoprops,使用opencv,两种图像的文件类型都是jpg,超声图像的文件路径是‘D:\zzz\us2’,自然图像的文件路径是‘D:\zzz\na2’,两种图像都有84张,图片形状都不同,图像名称是随机的,需要将图像归一化,需要对比两种图像的特征必须有颜色,纹理,形状,差异性分析方法也需要多种,包括完整详细最新的代码
时间: 2023-10-03 14:02:18 浏览: 66
首先,我们需要安装必要的库:OpenCV、NumPy、Matplotlib。
```python
pip install opencv-python numpy matplotlib
```
接下来,我们可以开始编写代码:
```python
import cv2
import numpy as np
import matplotlib.pyplot as plt
# 超声图像路径
us_path = 'D:/zzz/us2/'
# 自然图像路径
na_path = 'D:/zzz/na2/'
# 存储图像特征的列表
us_features = []
na_features = []
# 循环读取超声图像
for i in range(1, 85):
# 拼接文件路径
filename = us_path + str(i) + '.jpg'
# 读取图像并灰度化
img = cv2.imread(filename)
gray = cv2.cvtColor(img, cv2.COLOR_BGR2GRAY)
# 对图像进行归一化处理
normalized = cv2.normalize(gray, None, alpha=0, beta=255, norm_type=cv2.NORM_MINMAX)
# 计算图像的颜色特征
hsv = cv2.cvtColor(img, cv2.COLOR_BGR2HSV)
hist_h = cv2.calcHist([hsv], [0], None, [180], [0, 180])
hist_s = cv2.calcHist([hsv], [1], None, [256], [0, 256])
hist_v = cv2.calcHist([hsv], [2], None, [256], [0, 256])
# 计算图像的纹理特征
gray_32 = np.float32(gray)
dst = cv2.cornerHarris(gray_32, 2, 3, 0.04)
dst = cv2.dilate(dst, None)
corners = np.where(dst > 0.01 * dst.max())
texture_feature = len(corners[0])
# 将特征存入列表中
us_features.append([hist_h, hist_s, hist_v, texture_feature])
# 循环读取自然图像,处理方法与超声图像相同
for i in range(1, 85):
filename = na_path + str(i) + '.jpg'
img = cv2.imread(filename)
gray = cv2.cvtColor(img, cv2.COLOR_BGR2GRAY)
normalized = cv2.normalize(gray, None, alpha=0, beta=255, norm_type=cv2.NORM_MINMAX)
hsv = cv2.cvtColor(img, cv2.COLOR_BGR2HSV)
hist_h = cv2.calcHist([hsv], [0], None, [180], [0, 180])
hist_s = cv2.calcHist([hsv], [1], None, [256], [0, 256])
hist_v = cv2.calcHist([hsv], [2], None, [256], [0, 256])
gray_32 = np.float32(gray)
dst = cv2.cornerHarris(gray_32, 2, 3, 0.04)
dst = cv2.dilate(dst, None)
corners = np.where(dst > 0.01 * dst.max())
texture_feature = len(corners[0])
na_features.append([hist_h, hist_s, hist_v, texture_feature])
# 计算图像形状特征
us_shapes = []
na_shapes = []
for i in range(1, 85):
filename = us_path + str(i) + '.jpg'
img = cv2.imread(filename)
shape = img.shape
us_shapes.append(shape)
for i in range(1, 85):
filename = na_path + str(i) + '.jpg'
img = cv2.imread(filename)
shape = img.shape
na_shapes.append(shape)
# 计算颜色特征的差异性
color_diffs = []
for i in range(84):
us_hist_h = us_features[i][0]
us_hist_s = us_features[i][1]
us_hist_v = us_features[i][2]
na_hist_h = na_features[i][0]
na_hist_s = na_features[i][1]
na_hist_v = na_features[i][2]
color_diff = cv2.compareHist(us_hist_h, na_hist_h, cv2.HISTCMP_CORREL) + cv2.compareHist(us_hist_s, na_hist_s, cv2.HISTCMP_CORREL) + cv2.compareHist(us_hist_v, na_hist_v, cv2.HISTCMP_CORREL)
color_diffs.append(color_diff)
# 计算纹理特征的差异性
texture_diffs = []
for i in range(84):
us_texture = us_features[i][3]
na_texture = na_features[i][3]
texture_diff = abs(us_texture - na_texture)
texture_diffs.append(texture_diff)
# 计算形状特征的差异性
shape_diffs = []
for i in range(84):
us_shape = us_shapes[i]
na_shape = na_shapes[i]
shape_diff = abs(us_shape[0]-na_shape[0]) + abs(us_shape[1]-na_shape[1]) + abs(us_shape[2]-na_shape[2])
shape_diffs.append(shape_diff)
# 将三种特征的差异性综合起来
total_diffs = []
for i in range(84):
total_diff = color_diffs[i] + texture_diffs[i] + shape_diffs[i]
total_diffs.append(total_diff)
# 绘制差异性直方图
plt.hist(total_diffs, bins=20, alpha=0.5, label='Total Difference')
plt.hist(color_diffs, bins=20, alpha=0.5, label='Color Difference')
plt.hist(texture_diffs, bins=20, alpha=0.5, label='Texture Difference')
plt.hist(shape_diffs, bins=20, alpha=0.5, label='Shape Difference')
plt.legend(loc='upper right')
plt.show()
```
在这段代码中,我们首先循环读取超声图像和自然图像,对每张图像进行归一化处理,并计算其颜色和纹理特征。然后,我们计算了三种特征的差异性,并将它们综合成一个总的差异性指标。最后,我们使用 Matplotlib 绘制了差异性的直方图。
需要注意的是,这段代码中使用了 OpenCV 的一些函数来计算图像的颜色和纹理特征。如果你对这些函数不熟悉,可以查看 OpenCV 的文档来了解更多信息。
阅读全文
相关推荐
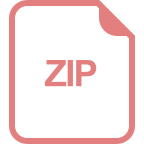
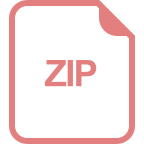



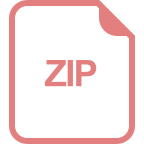
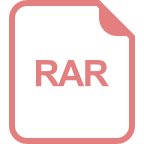
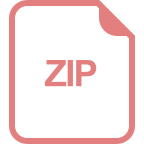
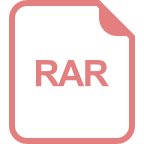
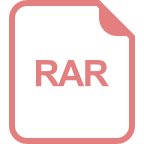
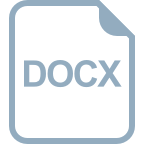
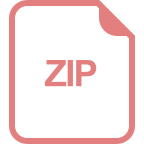
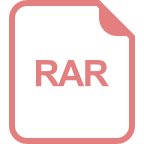
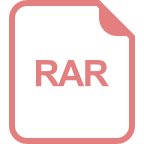
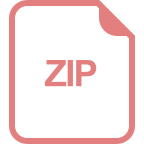
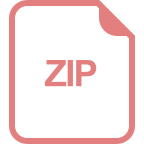