c#的winform实现低功耗蓝牙ble的搜索和数据读写功能代码
时间: 2024-03-25 11:37:21 浏览: 257
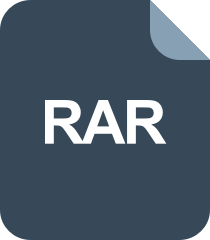
C#实现BLE低功耗蓝牙调试助手源代码

以下是一个简单的C# WinForms程序,实现BLE设备的搜索和数据读写功能。需要使用NuGet包管理器安装`Windows.Devices.Bluetooth`和`Windows.Devices.Bluetooth.GenericAttributeProfile`两个库。
```
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Linq;
using System.Runtime.InteropServices.WindowsRuntime;
using System.Text;
using System.Threading.Tasks;
using System.Windows.Forms;
using Windows.Devices.Bluetooth;
using Windows.Devices.Bluetooth.Advertisement;
using Windows.Devices.Bluetooth.GenericAttributeProfile;
namespace BLETest
{
public partial class Form1 : Form
{
private BluetoothLEAdvertisementWatcher _watcher;
private GattCharacteristic _characteristic;
public Form1()
{
InitializeComponent();
// 初始化BLE搜索器
_watcher = new BluetoothLEAdvertisementWatcher();
_watcher.Received += OnAdvertisementReceived;
}
private async void OnAdvertisementReceived(BluetoothLEAdvertisementWatcher sender, BluetoothLEAdvertisementReceivedEventArgs args)
{
// 获取广告中的设备名称
string deviceName = args.Advertisement.LocalName;
// 如果设备名称包含“BLE设备”字样,则连接该设备
if (deviceName.Contains("BLE设备"))
{
// 停止搜索
_watcher.Stop();
// 连接设备
BluetoothLEDevice device = await BluetoothLEDevice.FromBluetoothAddressAsync(args.BluetoothAddress);
// 获取设备的服务和特征
GattDeviceServicesResult result = await device.GetGattServicesAsync();
GattDeviceService service = result.Services.FirstOrDefault(s => s.Uuid == new Guid("0000FFF0-0000-1000-8000-00805F9B34FB"));
GattCharacteristicsResult charsResult = await service.GetCharacteristicsAsync();
_characteristic = charsResult.Characteristics.FirstOrDefault(c => c.Uuid == new Guid("0000FFF1-0000-1000-8000-00805F9B34FB"));
// 订阅特征的通知
GattCommunicationStatus status = await _characteristic.WriteClientCharacteristicConfigurationDescriptorAsync(GattClientCharacteristicConfigurationDescriptorValue.Notify);
if (status == GattCommunicationStatus.Success)
{
_characteristic.ValueChanged += OnCharacteristicValueChanged;
// 显示连接成功的提示信息
MessageBox.Show("连接成功!");
}
else
{
// 显示连接失败的提示信息
MessageBox.Show("连接失败!");
}
}
}
private async void OnCharacteristicValueChanged(GattCharacteristic sender, GattValueChangedEventArgs args)
{
// 读取设备发送的数据
byte[] buffer = args.CharacteristicValue.ToArray();
string message = Encoding.ASCII.GetString(buffer);
// 将数据显示在文本框中
textBox1.Text = message;
}
private void btnSearch_Click(object sender, EventArgs e)
{
// 开始搜索BLE设备
_watcher.Start();
}
private async void btnConnect_Click(object sender, EventArgs e)
{
// 发送数据到设备
byte[] buffer = Encoding.ASCII.GetBytes(textBox2.Text);
GattCommunicationStatus status = await _characteristic.WriteValueAsync(buffer.AsBuffer());
if (status == GattCommunicationStatus.Success)
{
// 显示发送成功的提示信息
MessageBox.Show("发送成功!");
}
else
{
// 显示发送失败的提示信息
MessageBox.Show("发送失败!");
}
}
}
}
```
在上面的代码中,我们使用`BluetoothLEAdvertisementWatcher`类进行BLE设备的搜索,使用`BluetoothLEDevice`类连接设备,使用`GattDeviceService`类获取设备的服务和特征,使用`GattCharacteristic`类订阅特征的通知,并使用`WriteValueAsync`方法向设备发送数据。当设备发送数据时,我们通过`ValueChanged`事件读取设备的数据,并将数据显示在文本框中。
阅读全文
相关推荐
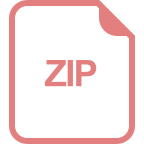
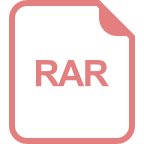
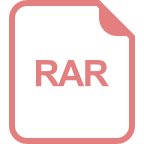
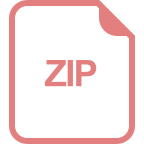
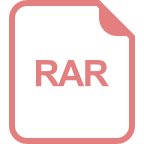
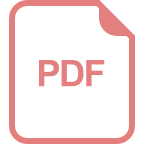
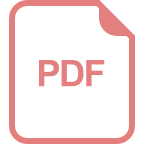
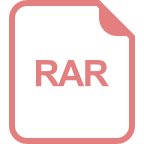
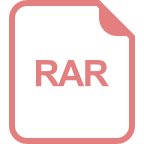
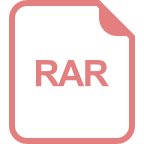
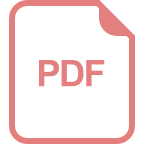
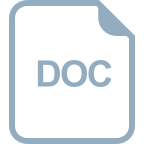