1.编写程序,对于链表list对象t中的数据,利用sort算法实现排序,然后输出
时间: 2024-05-11 09:20:15 浏览: 58
以下是一个示例代码:
```python
t = [3, 1, 4, 1, 5, 9, 2, 6, 5, 3, 5]
t.sort()
print(t)
```
输出结果为:
```
[1, 1, 2, 3, 3, 4, 5, 5, 5, 6, 9]
```
在这个示例代码中,我们使用了 Python 的内置函数 `sort()` 来对列表 `t` 中的数据进行排序,然后输出排序后的结果。如果您想对其他类型的链表进行排序,只需要将 `t` 替换成您自己的链表对象即可。
相关问题
.编写程序,对于链表list对象t中的数据,利用sort算法实现排序,然后输出。(sy4_3.cpp)
以下是示例代码:
```cpp
#include <iostream>
#include <list>
#include <algorithm>
using namespace std;
int main() {
list<int> t = {5, 3, 1, 4, 2};
t.sort();
for(auto i : t) {
cout << i << " ";
}
return 0;
}
```
输出结果为:
```
1 2 3 4 5
```
在这个示例中,我们使用了STL中的list容器来存储数据,并使用sort算法对其进行排序。最后,我们遍历list中的元素,输出排序后的结果。
编写程序,对于链表list对象t中的数据,利用sort算法实现排序,然后输出。
下面是一个使用C++ STL中的list容器和sort算法对链表进行排序的示例代码:
```c++
#include <iostream>
#include <list>
#include <algorithm>
int main() {
std::list<int> t = {5, 2, 9, 1, 3};
// 使用sort算法对链表进行排序
t.sort();
// 输出排序后的链表元素
for (auto it = t.begin(); it != t.end(); ++it) {
std::cout << *it << " ";
}
return 0;
}
```
程序首先创建了一个list对象t,并初始化了一些数据。然后使用list容器的sort成员函数对链表进行排序,最后使用迭代器循环输出排序后的链表元素。
阅读全文
相关推荐
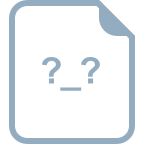
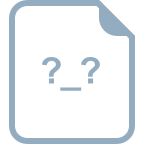




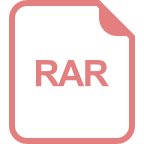
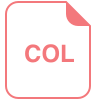





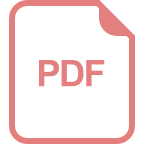