1. 用ArrayList或 2. 利用Hashset实现彩票机选功能,随机生成n个不同的整数,保存在Hash中,输出生成的顺序和号码
时间: 2024-02-18 16:01:12 浏览: 30
好的,我明白了你的问题。这是一个关于彩票机选的问题,你想知道如何使用ArrayList或Hashset来随机生成n个不同的整数,并将它们保存在Hash中,最后输出它们的顺序和号码。下面是两种实现方法:
1. 使用ArrayList来实现彩票机选功能:
```java
import java.util.ArrayList;
import java.util.Collections;
public class LotteryMachine {
public static void main(String[] args) {
int n = 5; // 生成的不同整数个数
ArrayList<Integer> numbers = new ArrayList<Integer>();
// 随机生成n个不同的整数
for (int i = 0; i < n; i++) {
int num = (int) (Math.random() * 100) + 1; // 生成1~100之间的随机整数
if (!numbers.contains(num)) {
numbers.add(num);
} else {
i--;
}
}
// 输出生成的顺序和号码
System.out.println("生成的顺序和号码为:");
for (int i = 0; i < numbers.size(); i++) {
System.out.print(numbers.get(i) + " ");
}
}
}
```
2. 使用Hashset来实现彩票机选功能:
```java
import java.util.HashSet;
import java.util.Iterator;
public class LotteryMachine {
public static void main(String[] args) {
int n = 5; // 生成的不同整数个数
HashSet<Integer> numbers = new HashSet<Integer>();
// 随机生成n个不同的整数
while (numbers.size() < n) {
int num = (int) (Math.random() * 100) + 1; // 生成1~100之间的随机整数
numbers.add(num);
}
// 输出生成的顺序和号码
System.out.println("生成的顺序和号码为:");
Iterator<Integer> it = numbers.iterator();
while (it.hasNext()) {
System.out.print(it.next() + " ");
}
}
}
```
这两种方法都可以实现彩票机选功能,你可以根据自己的需求选择其中一种来使用。
相关推荐
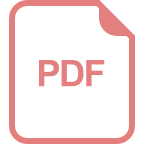
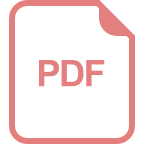
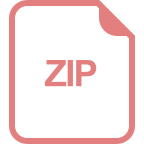














