使用vue帮我写一个好看的文件发送模板.
时间: 2024-02-12 18:02:59 浏览: 122
好的,以下是一个使用 Vue 编写的简单但美观的文件发送模板示例:
首先,我们需要创建一个Vue组件来实现文件发送模板。以下是示例代码:
```html
<template>
<div class="container">
<h2>文件发送</h2>
<form>
<div class="form-group">
<label for="recipient">收件人:</label>
<input type="text" id="recipient" v-model="recipient" />
</div>
<div class="form-group">
<label for="subject">主题:</label>
<input type="text" id="subject" v-model="subject" />
</div>
<div class="form-group">
<label for="message">消息:</label>
<textarea id="message" v-model="message"></textarea>
</div>
<div class="form-group">
<label for="file">选择文件:</label>
<input type="file" id="file" ref="fileInput" @change="onFileSelected" />
</div>
<div class="form-group">
<button type="button" @click="sendFile">发送</button>
</div>
</form>
</div>
</template>
<script>
export default {
data() {
return {
recipient: '',
subject: '',
message: '',
file: null,
};
},
methods: {
onFileSelected(event) {
this.file = event.target.files[0];
},
sendFile() {
// 发送文件
},
},
};
</script>
<style>
.container {
max-width: 800px;
margin: 0 auto;
padding: 2rem;
border: 1px solid #ccc;
border-radius: 5px;
box-shadow: 0 2px 10px rgba(0, 0, 0, 0.1);
}
form {
display: flex;
flex-direction: column;
}
.form-group {
display: flex;
flex-direction: column;
margin-bottom: 1rem;
}
label {
font-weight: bold;
}
input[type='text'],
textarea {
padding: 0.5rem;
border: 1px solid #ccc;
border-radius: 5px;
resize: none;
}
button {
padding: 0.5rem 1rem;
background-color: #007bff;
color: #fff;
border: none;
border-radius: 5px;
font-size: 1rem;
cursor: pointer;
}
button:hover {
background-color: #0069d9;
}
</style>
```
这个组件包含一个表单,用于输入收件人、主题、消息和要发送的文件。我们使用了一些 CSS 属性来美化页面。
在 `sendFile` 方法中,我们可以使用 `axios` 库来发送文件。以下是示例代码:
```javascript
import axios from 'axios';
methods: {
sendFile() {
const formData = new FormData();
formData.append('recipient', this.recipient);
formData.append('subject', this.subject);
formData.append('message', this.message);
formData.append('file', this.file);
axios.post('/api/sendfile', formData).then(() => {
// 文件已发送
});
},
},
```
在这个方法中,我们首先创建一个 `FormData` 对象,然后将表单数据添加到其中。最后,我们使用 `axios.post` 方法发送文件。
在 `onFileSelected` 方法中,我们需要获取用户选择的文件。我们可以使用 `$refs` 来访问文件输入框,并从 `event.target.files` 中获取文件。以下是示例代码:
```javascript
onFileSelected(event) {
this.file = event.target.files[0];
},
```
最后,我们需要在 Vue 组件中引入 axios 库,以便我们可以发送 HTTP 请求。以下是示例代码:
```javascript
<script>
import axios from 'axios';
export default {
// ...
methods: {
// ...
},
};
</script>
```
这就是一个简单的文件发送模板的实现示例。您可以根据您的实际需求进行修改和扩展。希望可以帮助到您!
阅读全文
相关推荐
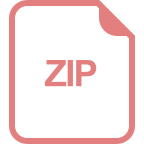
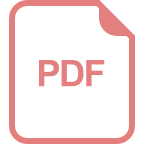
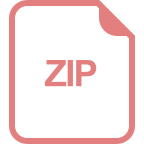
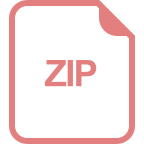
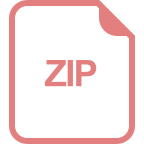
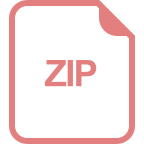
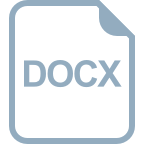
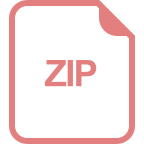
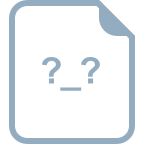
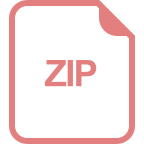
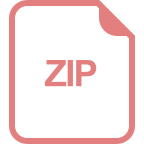
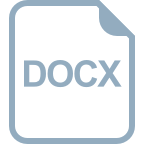






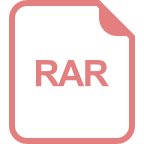