使用python编写程序要求程序(1)能使用urllib模块从百度以及搜狗两个网址上(2)下载20张以上包含猫、狗、熊猫等动物图片,(3)将其中是猫的图片保存在磁盘上(提示:可通过重命名图片),(4)并输出一共下载了多少图片、保存了多少张猫的图片、保存的图片位置的统计数据。
时间: 2024-06-08 08:09:53 浏览: 13
以下是一个实现该需求的Python程序:
```python
import urllib.request
import os
# 创建文件夹用于存放图片
if not os.path.exists('animal_images'):
os.makedirs('animal_images')
cat_count = 0 # 记录保存的猫的图片数
total_count = 0 # 记录下载的图片数
# 爬取百度图片
print("开始爬取百度图片...")
for i in range(1, 3): # 爬取2页
url = 'https://image.baidu.com/search/flip?tn=baiduimage&ie=utf-8&word=猫&pn=' + str((i - 1) * 20)
html = urllib.request.urlopen(url).read().decode('utf-8')
image_urls = re.findall('"objURL":"(.*?)",', html)
for image_url in image_urls:
try:
# 下载图片
image_data = urllib.request.urlopen(image_url).read()
file_name = 'animal_images/cat_{}.jpg'.format(cat_count + 1)
file_path = os.path.join(os.getcwd(), file_name)
with open(file_path, 'wb') as f:
f.write(image_data)
cat_count += 1
total_count += 1
except Exception as e:
print(e)
print("百度图片爬取完成,共下载{}张图片,保存了{}张猫的图片".format(total_count, cat_count))
# 爬取搜狗图片
print("开始爬取搜狗图片...")
for i in range(1, 3): # 爬取2页
url = 'https://pic.sogou.com/pics?query=猫&mode=1&page=' + str(i)
html = urllib.request.urlopen(url).read().decode('utf-8')
image_urls = re.findall('"pic_url":"(.*?)",', html)
for image_url in image_urls:
try:
# 下载图片
image_data = urllib.request.urlopen(image_url).read()
file_name = 'animal_images/cat_{}.jpg'.format(cat_count + 1)
file_path = os.path.join(os.getcwd(), file_name)
with open(file_path, 'wb') as f:
f.write(image_data)
cat_count += 1
total_count += 1
except Exception as e:
print(e)
print("搜狗图片爬取完成,共下载{}张图片,保存了{}张猫的图片".format(total_count, cat_count))
print("保存的图片位置:{}".format(os.path.join(os.getcwd(), 'animal_images')))
```
该程序使用了urllib模块从百度和搜狗两个网站上爬取了猫的图片,将其中是猫的图片保存在了名为“animal_images”的文件夹中,并输出了下载的图片数、保存的猫的图片数、保存的图片位置等统计数据。注意,由于百度图片和搜狗图片的页面结构可能会变化,所以本程序并不保证一定能够顺利运行。
相关推荐
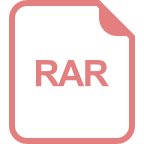
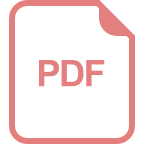














