用python代码实现小批量梯度上升算法
时间: 2024-02-28 13:26:43 浏览: 167
好的,以下是用Python实现小批量梯度上升算法的示例代码:
```python
import numpy as np
# 定义 sigmoid 函数
def sigmoid(x):
return 1.0 / (1 + np.exp(-x))
# 定义小批量梯度上升算法
def batch_gradient_ascent(data, labels, batch_size=20, alpha=0.001, max_iter=5000):
data_matrix = np.mat(data)
label_matrix = np.mat(labels).transpose()
m, n = np.shape(data_matrix)
# 初始化权重向量
weights = np.ones((n, 1))
# 迭代更新权重
for i in range(max_iter):
# 随机选择一个样本
batch_index = np.random.choice(m, batch_size)
batch_data = data_matrix[batch_index]
batch_label = label_matrix[batch_index]
# 计算梯度
h = sigmoid(batch_data * weights)
error = batch_label - h
weights = weights + alpha * batch_data.transpose() * error
return weights
```
其中 `data` 是训练数据,`labels` 是标签,`batch_size` 是批量大小,`alpha` 是学习率,`max_iter` 是最大迭代次数。函数返回的是训练后的权重向量。
阅读全文
相关推荐
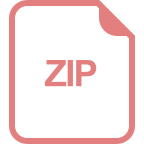
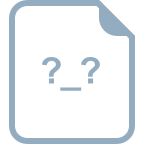







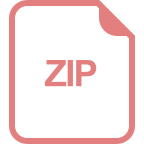
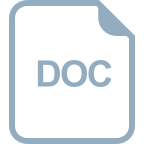
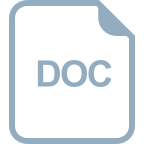
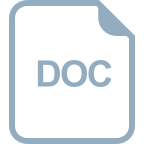




