w25q128用C实现读写操作
时间: 2023-10-10 12:05:50 浏览: 80
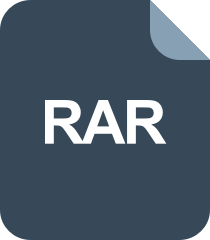
用C语言实现文件的读写操作
以下为w25q128的C语言读写操作代码示例:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <wiringPiSPI.h>
#define W25Q128_CS 0 // W25Q128芯片片选引脚
#define SPI_SPEED 500000 // SPI通信速度
// W25Q128的命令集
#define W25Q128_CMD_WRITE_ENABLE 0x06
#define W25Q128_CMD_WRITE_DISABLE 0x04
#define W25Q128_CMD_READ_STATUS_REG1 0x05
#define W25Q128_CMD_READ_DATA 0x03
#define W25Q128_CMD_PAGE_PROGRAM 0x02
#define W25Q128_CMD_ERASE_SECTOR 0x20
#define W25Q128_CMD_ERASE_CHIP 0xC7
// W25Q128的状态寄存器1位定义
#define W25Q128_SR1_BUSY_BIT (1 << 0)
// W25Q128的函数声明
static void w25q128_write_enable();
static void w25q128_write_disable();
static void w25q128_wait_for_busy();
static void w25q128_read_status_reg1(unsigned char *status);
static void w25q128_write_data(unsigned int addr, unsigned char *buf, unsigned int len);
static void w25q128_read_data(unsigned int addr, unsigned char *buf, unsigned int len);
static void w25q128_erase_sector(unsigned int addr);
static void w25q128_erase_chip();
int main(int argc, char *argv[])
{
unsigned char buf[256];
// 初始化SPI
wiringPiSPISetup(0, SPI_SPEED);
// 读取W25Q128的状态寄存器1
unsigned char status;
w25q128_read_status_reg1(&status);
printf("W25Q128 Status Reg1: 0x%02x\n", status);
// 写入数据
memset(buf, 0xaa, sizeof(buf));
w25q128_write_data(0x10000, buf, sizeof(buf));
// 读取数据
memset(buf, 0x00, sizeof(buf));
w25q128_read_data(0x10000, buf, sizeof(buf));
printf("Data: %02x%02x%02x%02x...\n", buf[0], buf[1], buf[2], buf[3]);
// 擦除扇区
w25q128_erase_sector(0x10000);
// 擦除整个芯片
w25q128_erase_chip();
return 0;
}
// 写使能
static void w25q128_write_enable()
{
unsigned char cmd = W25Q128_CMD_WRITE_ENABLE;
wiringPiSPIDataRW(0, &cmd, 1);
}
// 写禁止
static void w25q128_write_disable()
{
unsigned char cmd = W25Q128_CMD_WRITE_DISABLE;
wiringPiSPIDataRW(0, &cmd, 1);
}
// 等待忙结束
static void w25q128_wait_for_busy()
{
unsigned char status = 0;
do {
w25q128_read_status_reg1(&status);
delay(1);
} while (status & W25Q128_SR1_BUSY_BIT);
}
// 读取状态寄存器1
static void w25q128_read_status_reg1(unsigned char *status)
{
unsigned char cmd = W25Q128_CMD_READ_STATUS_REG1;
wiringPiSPIDataRW(0, &cmd, 1);
wiringPiSPIDataRW(0, status, 1);
}
// 写入数据
static void w25q128_write_data(unsigned int addr, unsigned char *buf, unsigned int len)
{
w25q128_write_enable();
unsigned char cmd[4];
cmd[0] = W25Q128_CMD_PAGE_PROGRAM;
cmd[1] = (addr >> 16) & 0xff;
cmd[2] = (addr >> 8) & 0xff;
cmd[3] = addr & 0xff;
wiringPiSPIDataRW(0, cmd, 4);
wiringPiSPIDataRW(0, buf, len);
w25q128_wait_for_busy();
w25q128_write_disable();
}
// 读取数据
static void w25q128_read_data(unsigned int addr, unsigned char *buf, unsigned int len)
{
unsigned char cmd[4];
cmd[0] = W25Q128_CMD_READ_DATA;
cmd[1] = (addr >> 16) & 0xff;
cmd[2] = (addr >> 8) & 0xff;
cmd[3] = addr & 0xff;
wiringPiSPIDataRW(0, cmd, 4);
wiringPiSPIDataRW(0, buf, len);
}
// 擦除扇区
static void w25q128_erase_sector(unsigned int addr)
{
w25q128_write_enable();
unsigned char cmd[4];
cmd[0] = W25Q128_CMD_ERASE_SECTOR;
cmd[1] = (addr >> 16) & 0xff;
cmd[2] = (addr >> 8) & 0xff;
cmd[3] = addr & 0xff;
wiringPiSPIDataRW(0, cmd, 4);
w25q128_wait_for_busy();
w25q128_write_disable();
}
// 擦除整个芯片
static void w25q128_erase_chip()
{
w25q128_write_enable();
unsigned char cmd = W25Q128_CMD_ERASE_CHIP;
wiringPiSPIDataRW(0, &cmd, 1);
w25q128_wait_for_busy();
w25q128_write_disable();
}
```
需要注意的是,在使用SPI通信时,需要先初始化SPI,然后通过wiringPiSPIDataRW函数进行读写操作。同时,为了避免写入数据时出现错误,需要先执行写使能操作,然后等待芯片忙结束后再执行写禁止操作。
阅读全文
相关推荐

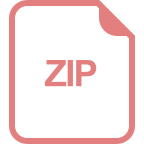

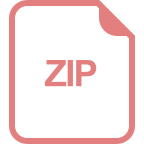
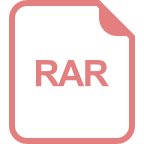
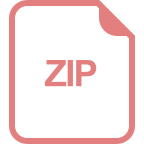
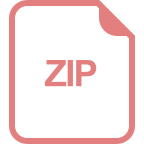
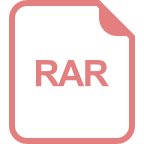
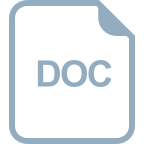
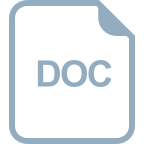
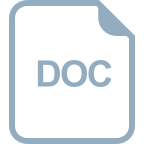
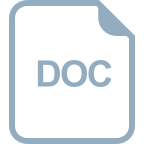



