用java给出实现基于TCP socket的图形化点对点聊天应用的代码
时间: 2024-02-18 16:03:40 浏览: 18
以下是一个简单的基于TCP socket的图形化点对点聊天应用的Java代码示例:
Server端:
```java
import java.io.*;
import java.net.*;
import javax.swing.*;
public class Server extends JFrame {
private JTextField textField;
private JTextArea chatArea;
private ObjectOutputStream output;
private ObjectInputStream input;
private ServerSocket server;
private Socket connection;
public Server() {
super("Server");
textField = new JTextField();
textField.setEditable(false);
textField.addActionListener(e -> sendMessage(e.getActionCommand()));
add(textField, BorderLayout.NORTH);
chatArea = new JTextArea();
add(new JScrollPane(chatArea));
setSize(300, 150);
setVisible(true);
}
public void startRunning() {
try {
server = new ServerSocket(12345, 100);
while (true) {
try {
waitForConnection();
setupStreams();
whileChatting();
} catch (EOFException eofException) {
showMessage("\nServer terminated the connection!");
} finally {
closeConnection();
}
}
} catch (IOException ioException) {
ioException.printStackTrace();
}
}
private void waitForConnection() throws IOException {
showMessage("Waiting for someone to connect...\n");
connection = server.accept();
showMessage("Connected to " + connection.getInetAddress().getHostName());
}
private void setupStreams() throws IOException {
output = new ObjectOutputStream(connection.getOutputStream());
output.flush();
input = new ObjectInputStream(connection.getInputStream());
showMessage("\nStreams are now setup!\n");
}
private void whileChatting() throws IOException {
String message = "You are now connected!";
sendMessage(message);
ableToType(true);
do {
try {
message = (String) input.readObject();
showMessage("\n" + message);
} catch (ClassNotFoundException classNotFoundException) {
showMessage("\nUnknown object type received!");
}
} while (!message.equals("CLIENT - END"));
}
private void closeConnection() {
showMessage("\nClosing connection...\n");
ableToType(false);
try {
output.close();
input.close();
connection.close();
} catch (IOException ioException) {
ioException.printStackTrace();
}
}
private void sendMessage(String message) {
try {
output.writeObject("SERVER - " + message);
output.flush();
showMessage("\nSERVER - " + message);
} catch (IOException ioException) {
chatArea.append("\nError: Unable to send message!");
}
}
private void showMessage(String message) {
SwingUtilities.invokeLater(() -> chatArea.append(message));
}
private void ableToType(boolean isAble) {
SwingUtilities.invokeLater(() -> textField.setEditable(isAble));
}
}
```
Client端:
```java
import java.io.*;
import java.net.*;
import javax.swing.*;
public class Client extends JFrame {
private JTextField textField;
private JTextArea chatArea;
private ObjectOutputStream output;
private ObjectInputStream input;
private String message = "";
private String serverIP;
private Socket connection;
public Client(String host) {
super("Client");
serverIP = host;
textField = new JTextField();
textField.setEditable(false);
textField.addActionListener(e -> sendMessage(e.getActionCommand()));
add(textField, BorderLayout.NORTH);
chatArea = new JTextArea();
add(new JScrollPane(chatArea));
setSize(300, 150);
setVisible(true);
}
public void startRunning() {
try {
connectToServer();
setupStreams();
whileChatting();
} catch (EOFException eofException) {
showMessage("\nClient terminated the connection!");
} catch (IOException ioException) {
ioException.printStackTrace();
} finally {
closeConnection();
}
}
private void connectToServer() throws IOException {
showMessage("Attempting connection...\n");
connection = new Socket(InetAddress.getByName(serverIP), 12345);
showMessage("Connected to " + connection.getInetAddress().getHostName());
}
private void setupStreams() throws IOException {
output = new ObjectOutputStream(connection.getOutputStream());
output.flush();
input = new ObjectInputStream(connection.getInputStream());
showMessage("\nStreams are now setup!\n");
}
private void whileChatting() throws IOException {
ableToType(true);
do {
try {
message = (String) input.readObject();
showMessage("\n" + message);
} catch (ClassNotFoundException classNotFoundException) {
showMessage("\nUnknown object type received!");
}
} while (!message.equals("SERVER - END"));
}
private void closeConnection() {
showMessage("\nClosing connection...\n");
ableToType(false);
try {
output.close();
input.close();
connection.close();
} catch (IOException ioException) {
ioException.printStackTrace();
}
}
private void sendMessage(String message) {
try {
output.writeObject("CLIENT - " + message);
output.flush();
showMessage("\nCLIENT - " + message);
} catch (IOException ioException) {
chatArea.append("\nError: Unable to send message!");
}
}
private void showMessage(String message) {
SwingUtilities.invokeLater(() -> chatArea.append(message));
}
private void ableToType(boolean isAble) {
SwingUtilities.invokeLater(() -> textField.setEditable(isAble));
}
}
```
在主方法中启动Server和Client:
```java
public class Main {
public static void main(String[] args) {
Server server = new Server();
server.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
server.startRunning();
Client client = new Client("127.0.0.1");
client.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
client.startRunning();
}
}
```
这个例子实现了一个简单的基于TCP socket的图形化点对点聊天应用。Server和Client分别运行在两个不同的Java进程中。当Client连接到Server时,它会向Server发送一个连接请求,如果Server接受了这个请求,那么Server和Client之间的连接就建立了。一旦连接建立,Server和Client就可以通过输入文本消息来互相聊天。在这个例子中,我们使用了Java的Socket和ServerSocket类来实现TCP socket通信。
相关推荐
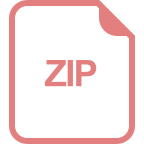
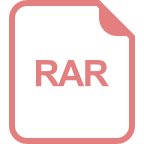
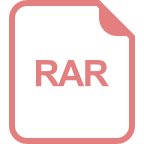
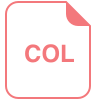
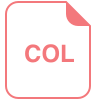












