用python帮我写一个涂鸦跳跃小游戏‘
时间: 2023-07-04 11:18:59 浏览: 75
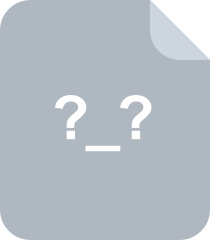
python 实现跳跃游戏
好的,下面是一个简单的涂鸦跳跃小游戏的 Python 代码实现,希望能够帮助到你:
```python
import pygame
import random
# 初始化 Pygame
pygame.init()
# 设置游戏窗口大小
window_width = 500
window_height = 500
game_window = pygame.display.set_mode((window_width, window_height))
# 设置游戏窗口标题
pygame.display.set_caption("涂鸦跳跃小游戏")
# 设置游戏时钟
clock = pygame.time.Clock()
# 加载玩家和障碍物图片
player_image = pygame.image.load("player.png")
obstacle_image = pygame.image.load("obstacle.png")
# 设置玩家和障碍物的大小
player_width = 50
player_height = 50
obstacle_width = 50
obstacle_height = 50
# 设置玩家的初始位置
player_x = window_width // 2 - player_width // 2
player_y = window_height - player_height
# 设置障碍物的初始位置和速度
obstacle_x = random.randint(0, window_width - obstacle_width)
obstacle_y = 0
obstacle_speed = 5
# 设置得分变量和字体
score = 0
font = pygame.font.SysFont("Arial", 30)
# 游戏主循环
running = True
while running:
# 处理游戏事件
for event in pygame.event.get():
if event.type == pygame.QUIT:
running = False
# 检测玩家是否与障碍物碰撞
if player_x < obstacle_x + obstacle_width and player_x + player_width > obstacle_x and player_y < obstacle_y + obstacle_height and player_y + player_height > obstacle_y:
running = False
# 移动玩家和障碍物
keys = pygame.key.get_pressed()
if keys[pygame.K_LEFT] and player_x > 0:
player_x -= 5
if keys[pygame.K_RIGHT] and player_x < window_width - player_width:
player_x += 5
obstacle_y += obstacle_speed
# 检测障碍物是否越界
if obstacle_y > window_height:
obstacle_x = random.randint(0, window_width - obstacle_width)
obstacle_y = 0
score += 1
obstacle_speed += 1
# 绘制游戏画面
game_window.fill((255, 255, 255))
game_window.blit(player_image, (player_x, player_y))
game_window.blit(obstacle_image, (obstacle_x, obstacle_y))
score_text = font.render("Score: " + str(score), True, (0, 0, 0))
game_window.blit(score_text, (10, 10))
pygame.display.update()
# 控制游戏帧率
clock.tick(60)
# 退出 Pygame
pygame.quit()
```
在运行这个程序之前,请确保你已经准备好了 `player.png` 和 `obstacle.png` 两个图片文件。你可以在网上搜索这两个图片,或者自己用涂鸦工具画出来。将这两个图片文件放在同一个目录下,然后运行这个程序,就可以开始玩涂鸦跳跃小游戏了。
阅读全文
相关推荐
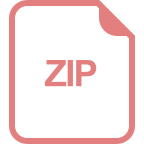
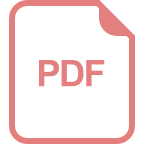
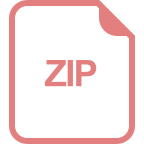
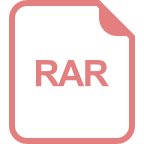
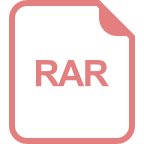
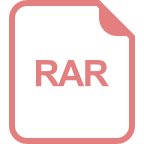
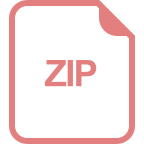
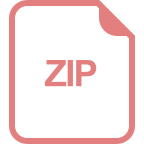
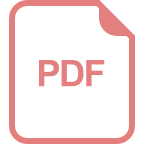
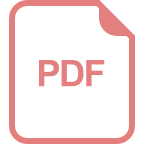
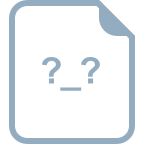
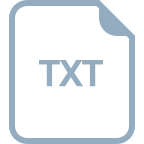
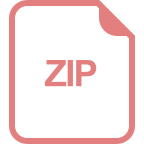
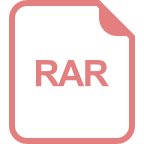