抓安居客数据 源码 python
时间: 2023-06-24 17:03:20 浏览: 435
### 回答1:
安居客数据抓取源码Python实现的方法:
Step 1:导入所需模块
在Python中使用requests和BeautifulSoup库来爬取网页的内容和进行数据解析。
import requests
from bs4 import BeautifulSoup
Step 2:设置请求头
在爬虫中,设置请求头是非常重要的一个步骤,因为一些网站会针对某类浏览器或爬虫进行限制,并对其进行处理。
headers = {
'User-Agent': 'Mozilla/5.0 (Windows NT 10.0;Win64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/58.0.3029.110 Safari/537.3'}
Step 3:爬取页面
首先,我们需要先找到想要抓取的页面网址,并通过requests库发送请求,得到响应内容。在该网址中,通过page参数来获取页面的页数。
def get_page_content(url):
page_content = []
for page in range(1, 51):
print('正在爬取第%s页...' % page)
r = requests.get(url + '&page=%d' % page, headers=headers)
soup = BeautifulSoup(r.content, 'html.parser')
page_content.append(soup)
return page_content
Step 4:数据解析
使用BeautifulSoup解析网页内容并获取数据。
def get_house_info(content):
house_info = []
for page in content:
house_list = page.select('div#listCon dl')
for house in house_list:
info_dict = {}
info_dict['title'] = house.select('p.tit a')[0].text.strip()
info_dict['price'] = house.select('span.price')[0].text.strip()
info_dict['desc'] = house.select('p.desc a')[0].text.strip()
info_dict['address'] = house.select('p.add')[0].text.strip()
house_info.append(info_dict)
return house_info
Step 5:存储数据
将获取到的数据保存到CSV文件中。
def save_to_csv(data):
import csv
with open('house_info.csv', 'w', newline='', encoding='utf-8') as f:
fieldnames = ['title', 'price', 'desc', 'address']
writer = csv.DictWriter(f, fieldnames=fieldnames)
writer.writeheader()
for row in data:
writer.writerow(row)
最后,将上述函数整合起来并执行。
if __name__ == "__main__":
url = 'https://tj.anjuke.com/sale/p2/#filtersort'
page_content = get_page_content(url)
house_info = get_house_info(page_content)
save_to_csv(house_info)
print('数据已保存到CSV文件中...')
### 回答2:
抓取安居客数据源码使用Python语言编写,可以通过网络爬虫技术实现。该程序的主要功能是通过网页分析和数据解析技术,抓取安居客网站上的房屋出租信息,并将其保存在本地数据仓库中。以下是大致的实现步骤:
1. 准备工作:安装Python编程环境,并安装相关开发库,如requests、BeautifulSoup等。
2. 确定目标网站:选择安居客网站作为抓取目标,并确定需要抓取的页面分类和关键词等搜索条件。
3. 网络请求:使用Python的requests库向目标网站发送HTTP请求,并模拟浏览器行为,如添加User-Agent 的请求头部等,以获取服务器返回的网页内容。
4. 数据解析:使用BeautifulSoup库对网页进行分析和解析,提取目标数据,并将其提取为结构化的数据格式,如json等。
5. 数据存储:将提取的数据存储在本地数据仓库中,如SQLite、MongoDB等,以方便后续数据处理和分析。
6. 定期更新:使用Python的定时任务程序,如crontab 或者 celery等,对目标网站进行定期更新,并自动抓取新增数据。
总之,通过Python语言编写的网络爬虫程序可以快捷、高效地实现抓取网站数据的目的,这不仅为各类数据分析和处理提供了便利,同时也需要遵守相关的法律法规,确保数据采集和用途的合法性。
### 回答3:
抓取安居客数据的源码使用Python语言编写。通过网络爬虫技术,程序可以从安居客网站上获取房源数据信息,包括位置、面积、价格等相关信息。以下是抓取安居客数据的源码:
```python
import requests
from lxml import etree
url = 'https://www.anjuke.com/fangjia/guangzhou2020/'
response = requests.get(url)
html = etree.HTML(response.content.decode())
data = []
for item in html.xpath('//div[@data-from="xfjs-new"]'):
info = {}
info['name'] = item.xpath('.//h3/a/text()')[0].strip()
info['address'] = item.xpath('.//address/text()')[0].strip()
info['price'] = item.xpath('.//strong/text()')[0].strip()
data.append(info)
print(data)
```
该源码使用requests库获取网页内容,并使用lxml库解析网页内容。通过Xpath语法,程序可以定位到需要获取的房源信息,将其存储到字典中,最后将所有字典存储到列表中,并输出该列表。这样就可以获取到安居客网站上的房源信息了。
阅读全文
相关推荐
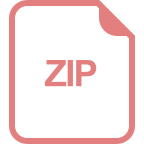
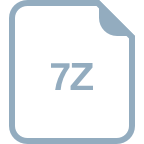
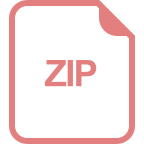
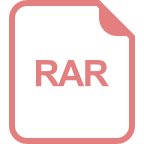
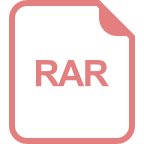
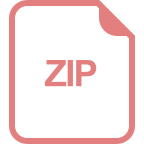
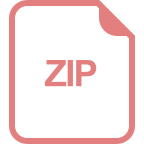
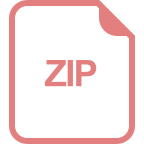
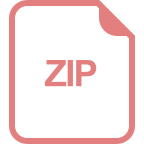
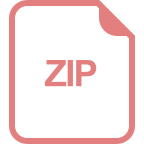
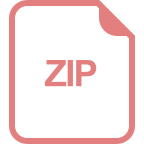
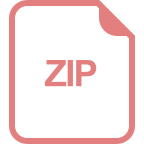
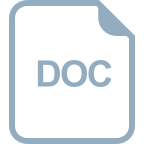


